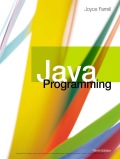
Explanation of Solution
Program code:
InchConversion.java
//import the packages
import java.util.Scanner;
//define a class InchConversion
public class InchConversion
{
//define main() method
public static void main(String[] args)
{
//create the object of Scanner class
Scanner in = new Scanner(System.in);
//prompt the user to enter the inches
System.out.print("Enter number of inches: ");
//scan for the input
int inches = in.nextInt();
//call the static methods
convertToFeet(inches);
convertToYards(inches);
}
//define the method convertToFeet()
public static void convertToFeet(int inches)
{
//convert the inches to feets and print on the screen
System.out.println(inches + " inches is " + (inches/12.0) + " feets.");
}
//define the method convertToYards()
public static void convertToYards(int inches)
{
//convert the inches to yards and print on the screen
System.out...

Trending nowThis is a popular solution!

Chapter 3 Solutions
EBK JAVA PROGRAMMING
- In java Create a class called StudentAge. This class should allow the student to enter name and date of birth. After that, determine student age. Lastly, display student name, date of birth and age.arrow_forwardusing JAVA Create a class called Transcript which will take the following parameters for its constructor:- 1. The UoF ID of the student 2. The full name of the student 3. The year in which the student joined 4. The student's GPA score NOTE: The class also has a class field which is a 2D array with 32 elements containing the course codes and grades obtained by the studentarrow_forwardWrite code to declare and create a Random class object (use the rand object reference variable). Then, using the nextInt method, create a list of expressions that produce random numbers in the following ranges, including the end points. Use the nextInt method's iteration that only takes an integer input.a. 0 to 10 b. 0 to 500c. 1 to 10d. 1 to 500e. 25 to 50f. -10 to 15 Write code to declare and create a Random class object (use the rand object reference variable). Then, using the nextInt method, create a list of expressions that produce random numbers in the following ranges, including the end points. Use the nextInt method's iteration that only takes an integer input.a. 0 to 10 b. 0 to 500c. 1 to 10d. 1 to 500e. 25 to 50f. -10 to 15arrow_forward
- TOPICS: Using Classes and Objects MUST BE IN JAVA. PLEASE USE COMMENTS AND WRITE THE CODE IN SIMPLEST FORM. 1. Write an application that prompts for and reads the user’s first and last name (separately), then displays a string composed of the first four characters of the user’s last name, followed by the first two letters of the user’s first name, followed by a random number in the range of 10 to 99. Assume that the last name is at least four letters long. Similar algorithms are sometimes used to generate usernames for new computer accounts. Testing: If the inputs were “Michael” and “Jackson,” the output should look like “JackMi42.” Include several tests with different inputs, including your own name.arrow_forwardPlease provide JAVA source code for following assignment. Please attach proper comments and read the full requirements. The local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license test The test has 20 multiple choice questions. Here are the correct answers: B D A A C A B A C D B C D A D C C D D A A student must correctly answer 15 of the 20 questions to pass the exam. Write a class named Drivertest that holds the correct answers to the test in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: passed. Returns true if the student passed the test, or false if the student failed totalCorrect. Returns the total number of correctly answered questions totalIncorrect. Returns the total number of incorrectly answered questions questionsMissed. An int array containing the…arrow_forwardA company accepts user orders by part numbers interactively. Users might make the following errors as they enter data: - The part number is not numeric. The quantity is not numeric. - The part number is too low (less than 0). - The part number is too high (more than 999). - The quantity ordered is too low (less than 1). - The quantity ordered is too high (more than 5,000). Create a class that stores an array of usable error messages; save the file as DataMessages.java. Create a DataException class; each object of this class will store one of the messages. Save the file as DataException.java. Create an application that prompts the user for a part number and quantity. Allow for the possibility of nonnumeric entries as well as out-of-range entries, and display the appropriate message when an error occurs. If no error occurs, display the message "Valid entry". Save the program as PartAndQuantityEntry.java.arrow_forward
- The LocalDate class includes an instance method named lengthOfMonth() that returns the number of days in the month. Write an application that uses methods in the LocalDate class to calculate how many days are left until the first day of next month. Display the result, including the name of the next month. Save the file as DaysTilNextMonth.java.arrow_forwardCreate an application named SalesTransactiobDemo that declares several SalesTransaction objects and displays their values and their sum. Name - The salesperson name (as a string) sales Amount- The sales amount (as a double) commission- The commission (as a double) RATE- A readonly field that stores the commission rate (as a double). Define a getRate() avcessor method that returns the RATE Include 3 constructors for the class. One constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value times commission rate. The second constructor accepts a name and sales amount, but sets the commission rate to 0 The third constructor accepts a name and sets all the other fields to 0arrow_forwardCreate a class name Taxpayer. Data fields for Taxpayer include yearly gross income and Social Security number (use an int for the type, and do not use dashes within the Social Security number). Methods include a constructor that requires a value for both data fields and two methods that each return one of the data field values. Write an application named USeTaxpayer that declares an array of 10 Taxpayer objects. Set each Social Security number to 999999999 and each gross income to zero. Display the 10 Taxpayer objects. Save the files as TAxpayer.java and USeTAxpayer.javaarrow_forward
- - Declare and create the following two Recipe objects then invoke the printRecipe method to print their info to the console: 1) Recipe name: Beef-Stuffed Peppers Preparation time: 55 minutes Servings: 4 Ingredients: 1 tablespoon olive oil 1 pound lean ground beef 1% cups water 1 (6 ounce) can tomato paste 4 green bell peppers, tops and seeds removed 2) Lemonade Preparation time: 15 minutes Servings: 10 Ingredients: Sugar Water Lemon juice Icearrow_forwardCreate an application in Java that uses card layout with three cards. The first card - a login card - should have two text fields, one for username and other for password. There are two users - Bob and Fred - whose passwords are "mubby and "goolag" respectively. If Bob logs in, switch to a card - the bob card - that has a text field, a text area and two buttons. If the first button is pressed, get the text from the text field and append it to the text area. If the second button is pressed, return to the login card. If Fred logs in, switch to a card - the fred card - that has three buttons. If the first button is pressed, change the background color to green. If the second button is pressed, change the background color to red. If the third button is pressed, return to login card.arrow_forwardCreate a constructor for the Student class you created. The constructor should initialize each Student’s ID number to 9999, his or her points earned to 12, and credit hours to 3 (resulting in a grade point average of 4.0). Write a program that demonstrates that the constructor works by instantiating an object and displaying the initial values. Save the application as ShowStudent2.java.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
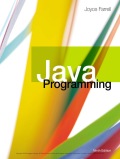
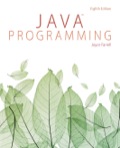