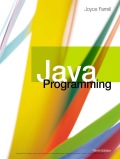
Concept explainers
Explanation of Solution
Program code:
Card.java
//create a class Card
public class Card
{
//declare class variables
private static char suit;
private static int value;
//define a class method getSuit()
public static char getSuit()
{
//return the value of suit
return suit;
}
//define a class method setSuit()
public static void setSuit(char suit)
{
//set the value of suit
Card.suit = suit;
}
//define a class method getValue()
public static int getValue()
{
//return the value of value
return value;
}
//define a class method setValue()
public static void setValue(int value)
{
//set the value of value
Card.value = value;
}
}
Explanation:
The above snippet of code is used to create a class “Card”. In the code,
- Import the required header files.
- Define a class “Card”
- Declare the class variables “suit” and “value”.
- Define the “getSuit()” method.
- Return the value of “suit”
- Define the “setSuit()” method.
- Set the value of “suit”
- Define the “getValue()” method.
- Return the value of “value”
- Define the “setValue()” method.
- Set the value of “value”
PickTwoCards.java
//import the required files
import java.util.Random;
//define a class PickTwoCards
public class PickTwoCards
{
//declare class member variables
final static int CARDS_IN_SUIT = 13;
final static char[] suits = {'s','h','d','c'};
//define the main() method
public static void main(String args[])
{
//create the object of class Card
Card firstCardSelected = selectACard();
//print the statement
System.out.println("Your FIRST Card is Selected with::");
System.out.println("********************************");
//call the method getSuit()
System.out.println("Suit ::"+firstCardSelected.getSuit());
//call the method getValue()
System.out.println("Value ::"+firstCardSelected.getValue()+"\n");
//create the object of class Card
Card secondCardSelected = selectACard();
//print the statement
System.out.println("Your SECOND Card is Selected with::");
System.out.println("********************************");
//call the method getSuit()
System.out.println("Suit ::"+secondCardSelected.getSuit());
//call the method getValue()
System.out.println("Value ::"+secondCardSelected.getValue()+"\n");
}
//define a method selectACard()
public static Card selectACard()
{
//create the object of class Card
Card card = new Card();
//call the method setSuit()
card...

Trending nowThis is a popular solution!

Chapter 3 Solutions
EBK JAVA PROGRAMMING
- Assignment Description This program will simulate part of the game of Yahtzee! This is a dice game that involves rolling five dice and scoring points based on what show up on those five dice. The players would record their scores on a score card, and then total them up, and the player with the larger total wins the game. A Yahtzee score card has two portions: The upper portion has spaces for six scores, obtained by adding up all of the 1's, 2's, 3's, etc. The lower portion has special scores for various combinations: Three of a kind -- at least 3 dice are the same number;the score is the sum of all five dice Four of a kind -- at least 4 dice are the same number;the score is the sum of all five dice Small straight -- four consecutive numbers are represented, e.g. 2345;the score is 25 points Large straight -- five consecutive numbers are represented, e.g. 23456;the score is 30 points Full House -- three of one kind, two of another; the score is 30 points Yahtzee! -- five of a kind; the…arrow_forwardPlease help with the following: Code in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple. Class TextEdit In text editing class. Its constructor it receives a string. The string will be stored in a field called initialValue using proper setter methods and proper documentation for Each of the methods. This class also contains another String field called: ”mixedResult” which is only defined globally and will be initialized using a method in the future in the main class namely the mix method. The public String toToken() method is a method that will use the initialValue field and it for the letter ’p’ or the letter ’A’. If it finds the letter, every element on the left side of the found letter will be stored in another string and returned. Example: If the initialValue was: ”This is a possible resource allocation”. The returning String will be: ”possible resource allocation”. Use control statements here iterate through the…arrow_forwardPYTHON: In this assignment, you will use all of the graphics commands you have learned to create an animated scene. Your program should have a clear theme and tell a story. You may pick any school-appropriate theme that you like. The program must include a minimum of: 5 circles 5 polygons 5 line commands 2 for loops 1 global variable You may wish to use the standard code for simplegui graphics below: import simplegui def draw_handler(canvas): frame = simplegui.create_frame('Testing', 600, 600) frame.set_canvas_background("Black") frame.set_draw_handler(draw_handler) frame.start()arrow_forward
- Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. Accept the ticket number from the agent and verify whether it is a valid number. Test the application with the following ticket numbers: . 123454; the comparison should evaluate to true . 147103; the comparison should evaluate to true . 154123; the comparison should evaluate to false Save the program as TicketNumber.java.arrow_forwardCode in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple. Class TextEdit In text editing class. Its constructor it receives a string. The string will be stored in a field called initialValue using proper setter methods and proper documentation for Each of the methods. This class also contains another String field called: ”mixedResult” which is only defined globally and will be initialized using a method in the future in the main class namely the mix method. The public String toToken() method is a method that will use the initialValue field and it for the letter ’p’ or the letter ’A’. If it finds the letter, every element on the left side of the found letter will be stored in another string and returned. Example: If the initialValue was: ”This is a possible resource allocation”. The returning String will be: ”possible resource allocation”. Use control statements here iterate through the string using a counter control…arrow_forwardshapes = [{'type': 'circle', 'x': 300, 'y': 300, 'radius': 100, 'color': 'cyan'},{'type': 'circle', 'x': 300, 'y': 300, 'radius': 10, 'color': 'white'},{'type': 'rectangle', 'x1': 500, 'y1': 500, 'x2': 550, 'y2': 580, 'color': 'green'},{'type': 'line', 'x': 0, 'y': 0, 'a': 100, 'b': 300, 'color': "black", 'width': 7},{'type': 'point', 'x': 200, 'y': 50, 'color': 'black'},{'type': 'point', 'x': 205, 'y': 50, 'color': 'black'},{'type': 'point', 'x': 210, 'y': 50, 'color': 'black'},{'type': 'triangle', 'x': 500, 'y': 100, 'a': 600, 'b': 100, 'c': 550, 'd': 200, 'color': 'yellow'},{'type': 'oval', 'x': 100, 'y': 100, 'a': 400, 'b': 400, 'color': 'red'},{'type': 'text', 'x': 500, 'y': 50, 'message': 'hello world!', 'color': 'blue'}] I'd like to take this list and write it to a new txt file. Ignoring the ':' , {}, and words within quotation marks. so the txt file created would look like this: circle, 300,300, 100, cyancircle, 300,300, 10, whiterectangle, 500,500, 550,580, greenline, 0,0,…arrow_forward
- The __str__ method of the Bank class (in bank.py) returns a string containing the accounts in random order. Design and implement a change that causes the accounts to be placed in the string in ascending order of name. Implement the __str__ method of the bank class so that it sorts the account values before printing them to the console. In order to sort the account values you will need to define the __eq__ and __lt__ methods in the SavingsAccount class (in savingsaccount.py). The __eq__ method should return True if the account names are equal during a comparison, False otherwise. The __lt__ method should return True if the name of one account is less than the name of another, False otherwise. The program should output in the following format: Test for createBank: Name: Jack PIN: 1001 Balance: 653.0 Name: Mark PIN: 1000 Balance: 377.0 ... ... ... Name: Name7 PIN: 1006 Balance: 100.0 bank.py """ File: bank.py Project 9.3 The str for Bank returns a string of accounts sorted by name.…arrow_forwardThe __str__ method of the Bank class (in bank.py) returns a string containing the accounts in random order. Design and implement a change that causes the accounts to be placed in the string in ascending order of name. Implement the __str__ method of the bank class so that it sorts the account values before printing them to the console. In order to sort the account values you will need to define the __eq__ and __lt__ methods in the SavingsAccount class (in savingsaccount.py). The __eq__ method should return True if the account names are equal during a comparison, False otherwise. The __lt__ method should return True if the name of one account is less than the name of another, False otherwise.arrow_forwardA class object can encapsulate more than one [answer].arrow_forward
- Gradient FillIn this labwork are asked to write a GUI application again using AWT. This is a fairly easy labworkthat is more about getting used to synchronized online learning. You are expected to:• Draw two rectangles.• Both of them should be filled using GradientPaint() function of AWT. (Check out itsfunction definition that is listed below.)• The first gradient should be parallel to the diagonal of the first rectangle. The colorgradient should not be repeated (acyclic).• The second gradient should be horizontal. The color gradient should be repeated forthis one (cyclic).• You are free to choose the colors but other than that your output should be similar tothe example screenshot given below.arrow_forward#include "TerminalPlayer.h" Card TerminalPlayer::playCard(const Card& opponentCard) { // if the opponentCard is a Joker we are going first // if the opponentCard is not a Joker we are going second and opponentCard is what our opponent played // Display the player's hand // prompt them to choose a card // remove that card from the hand and return that cardarrow_forwardC#Language: Create a Windows application using Visual Studio. Create a simple word-guessing game using ArrayList and StringBuilder. Create a short description of the game created and explain How it plays. Must show hints for the player what is the word should be guess.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
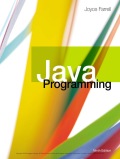