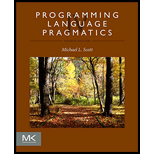
Explanation of Solution
Code which supports unary negation and the four standard arithmetic operators:
//include the required header files
#include <cmath>
using std::abs;
using std::round;
#include <iostream>
using std::ostream;
using std::cout;
//definition of class "rational"
class rational
{
//declare the required variables
int num;
int den;
//definition of "gcd" function
int gcd(int i, int j)
{
//set the absolute values
i = abs(i);
j = abs(j);
//check "i" is not equal to "j"
while (i != j)
{
//check "i" is greater than "j"
if (i > j)
/*subtract the values and store the result in "i"*/
i = i - j;
//otherwise
else
/*subtract the values and store the result in "j"*/
j = j - i;
}
//return the "i" value
return i;
}
//definition of "normalize" function
void normalize()
{
//check "den" is less than 0
if (den < 0)
{
//calculate numerator value
num = -num;
//calculate denominator value
den = -den;
}
//call the "gcd" function with a parameters
int g = gcd(num, den);
//calculate numerator value
num /= g;
//calculate denominator value
den /= g;
}
//access specifier
public:
//definition constructor
rational(int n, int d)
{
//set the values
num = n;
den = d;
//call the function
normalize();
}
// definition of constructor
rational(double r, double e)
{
//set the values
num = (int) r;
den = 1;
//check the condition
while (abs(r - ((double)num)/((double)den)) > e)
{
//increment the value
++den;
//calculate numerator value
num = round(den*r);
}
}
//definition of operator "-"
rational operator-()
{
//calculate numerator value
num = -num;
//return the value
return *this;
}
//definition of operator "+"
rational operator+(const rational o)
{
//declare the variable and calculate the value
int p = den * o.den;
//calculate numerator value
num = num * o.den + den * o.num;
//set the value
den = p;
//call the function
normalize();
//return the value
return *this;
}
//definition of operator "-"
rational operator-(const rational o)
{
//declare the variable and calculate the value
int p = den * o.den;
//calculate numerator value
num = num * o.den - den * o.num;
//set the value
den = p;
//call the function
normalize();
//return the value
return *this;
}
//definition of operator "*"
rational operator*(const rational o)
{
//calculate numerator value
num *= o.num;
//calculate denominator value
den *= o.den;
//call the function
normalize();
//return the value
return *this;
}
//definition of operator "/"
rational operator/(const rational o)
{
//calculate numerator value
num *= o.den;
//calculate denominator value
den *= o.num;
//call the function
normalize();
//return the value
return *this;
}
};
Explanation:
- The header files are included in the beginning of the program.
- The “rational” class is defined. Inside the class,
- The “num” and “den” variables are declared.
- The “gcd” function is defined
- Get the absolute values of “i” and “j” and store it in the corresponding variables.
- The “while” loop is used to check “i” is not equal to “j”.
- If the “i” value is greater than “j” value, then subtract the “i” and “j” value and store the result in the “i” variable.
- Otherwise, subtract the “j” and “i” value and store the result in the “j” variable.
- Return the “i” value.
- The “normalize” function is defined.
- If the “den” is less than 0, then calculate the “num” and “den” values.
- Call the “gcd” function with the parameters.
- Calculate the numerator and denominator values.
- The constructor of “rational” is defined with two parameters.
- Set the values
- Call the “normalize” function.
- Again, the constructor of “rational” is defined with another two parameters.
- Set the values.
- Check the “while” condition
- Increment the denominator value
- Calculate the numerator
- The operator “-” is defined
- Calculate the “num” value
- Return the result
- The operator “+” is defined with parameter
- Calculate the “p” and “num” values
- Set the value
- Call the “normalize” function
- Return the result
- The operator “-” is defined with parameter
- Calculate the “p” and “num” values
- Set the value
- Call the “normalize” function
- Return the result
- The operator “*” is defined with parameter
- Calculate the “den” and “num” values
- Call the “normalize” function
- Return the result
- The operator “/” is defined with parameter
- Calculate the “den” and “num” values
- Call the “normalize” function
- Return the result
Want to see more full solutions like this?
Chapter 3 Solutions
Programming Language Pragmatics, Fourth Edition
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
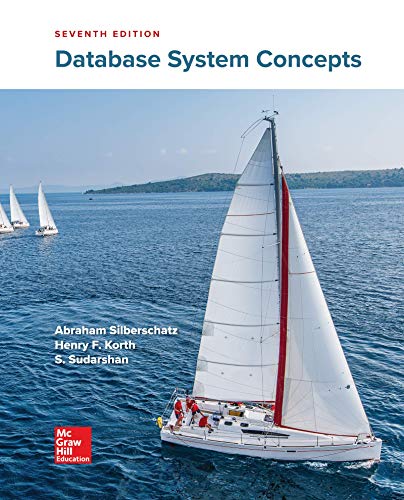
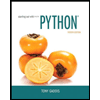
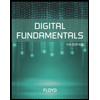
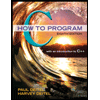
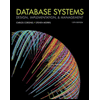
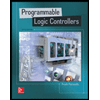