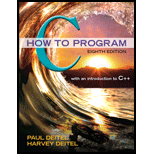
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 23, Problem 23.3E
Program Plan Intro
- To include header files and required namespaces.
- To define function template equalTemplate of type class.
- To define tool function isEqualTo with two values to be compare as arguments using template type EqualTemplate.
- Intiaiize the main() function.
- Prompt user to input two entities of same type.
- Call function isEqualTo to evaluate for eachtype.
Summary Introduction- This program evaluates equality of two entities using function templates.
Program Description- The program uses user defined function: isEqualTo() and evaluates the equality of two entities using function templates.
Expert Solution & Answer

Explanation of Solution
Program:
/* Program toevaluate the equality of two entities using function templates. . */ //header files #include<iostream> usingnamespacestd; //template defined template<classequalTemplate> //evaluates equality boolisEqualTo(equalTemplate input1,equalTemplate input2) { //check for qualtiy if( input1== input2) returntrue; elsereturnfalse; } //initialize main() function intmain() { int intVal1, intVal2; double floatVal1, floatVal2; char charVal1, charVal2; //Integer Comparison cout<<"Input two integer values:"; cin>>intVal1>>intVal2; cout<<intVal1<<" and "<<intVal2<<" are "<<(isEqualTo(intVal1, intVal2)?"Equal":"Not Equal")<<endl; //Double Comparison cout<<"Input two floating-point values:"; cin>>floatVal1>>floatVal2; cout<<floatVal1<<" and "<<floatVal2<<" are "<<(isEqualTo(floatVal1, floatVal2)?"Equal":"Not Equal")<<endl; //Character Comparison cout<<"Input two character values:"; cin>>charVal1>>charVal2; cout<<charVal1<<" and "<<charVal2<<" are "<<(isEqualTo(charVal1, charVal2)?"Equal":"Not Equal")<<endl; return0; }
Sample Output-
Input two integer values:23 44
23 and 44 are Not Equal
Input two floating-point values:23.33 45.5
23.33 and 45.5 are Not Equal
Input two character values: a A
a and A are Not Equal
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
What is the definition of operator and function overloading? Is there anything beneficial about it?
What do you mean by function overloading? Can you explain it with code?
Kindly give proper explanation and output I will rate your answer
Write a program in C++ that uses member functions like get data and set data and make a parameterized constructor,,, default constructor also to find tha area of a box(like cube) (.h&.pp)
Chapter 23 Solutions
C How to Program (8th Edition)
Ch. 23 - Prob. 23.3ECh. 23 - (Array Class Template) Reimplement class Array...Ch. 23 - Prob. 23.5ECh. 23 - Explain which is more like a stencil—a class...Ch. 23 - Prob. 23.7ECh. 23 - The compiler performs a matching process to...Ch. 23 - Prob. 23.9ECh. 23 - Prob. 23.10ECh. 23 - Review your answer to Exercise 23.10. Explain why...Ch. 23 - Prob. 23.12E
Knowledge Booster
Similar questions
- Can you please help me with these questions? 1) When the left operand of a function that overloads an operator is NOT an object of the class, or a reference to such an object, the function must be declared as a ___ because it is a ___ function. friend, non-member member, void member, overloaded friend, member 2) To overload the greater than operator, you define an operator method whose name is ___. > operator> >operator greaterThan 3) A(n) ___ ADT is used to store a homogeneous, one-dimensional, sequential, set of data items with a specific ordering. The ordering can be changed, as needed. list stack queue treearrow_forwardOperator and function overloading - what does it entail? In what ways may you benefit from itarrow_forwardWhat does operator and function overloading entail? In what ways might it be beneficial to you?arrow_forward
- Does a friend function violate the data hiding? Explain briefly. Write a program to swap variables of two classes using friend function. C++arrow_forwardUse Clojure to complete these questions: Please note that this is the beginning of the course so please use simple methods possible, thanks a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true. c. See attached picture.arrow_forwardHow we can pass the function pointer as a parameter give example.arrow_forward
- Write a definition for a void-function that has two int value parameters and outputs to the screen the product of these arguments. Write a main function that asks the user for these two numbers, reads them in, calls your function, then terminates. c++arrow_forwardPlease fill in the blanks for the following statements: The class function with no return type that is used to initialize data members is called the __________________________.arrow_forwardWrite a simple function template for predicate function isEqualTo that compares its two arguments of the same type with the equality operator (==) and returns true if they are equal and false otherwise. Use this function template in a program that calls isEqualTo only with a variety of fundamental types. Now write a separate version of the program that calls isEqualTo with a user-defined class type, but does not overload the equality operator. What happens when you attempt to run this program? Now overload the equality operator (with the operator function) operator==. Now what happens when you attempt to run this program?arrow_forward
- Use the friend function to rewrite the operator overloading of operator +arrow_forward- in this exercise, please do not include and use string class. The function is using only array notation and manipulation.- string functions such as strlen is not allowed.- it should not have multiple return statements in the same function- there should be no global variable.- the function should not traverse the arrays more than once (e.g. looping through the array once only) A C++ PROGRAM named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become{'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forwardUse Clojure: a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
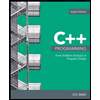
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning