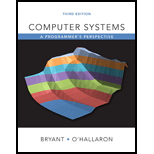
Practice Problem 2.32 (solution page 153)
You are assigned the task of writing code for a function tsub_ok, with arguments x and y, that will return 1 if computing x-y does not cause overflow. Having just written the code for Problem 2.30, you write the following:
/* Determine whether arguments can be subtracted without overflow */
/* WARNING: This code is buggy. */
int tsub_ok(int x, int y) {
return tadd_ok(x, -y)
}
For what values of x and y will this function give incorrect results? Writing a
correct version of this function.is left as an exercise (Problem 2.74).

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Software Engineering (10th Edition)
Absolute Java (6th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- splir + 1).) 7. (Numerical) Heron's formula for the area, A, of a triangle with sides of length a, b, and cie A = vIsts - aXs - b(s - c)] where (a +b+c) Write, test, and execute a function that accepts the values of a, b, and c as parameters from a calling function, and then calculates the values of s and [s(s - a)(s - b)(s - c)]. If this quantity is positive, the function calculates A. If the quantity is negative, a, b, and c do not form a triangle, and the function should set A = -1. The value of A should be returned by the function.arrow_forwardProblem 1 (Ransom Note Problem) in python A kidnapper kidnaps you and writes a ransom note. He does not write it by hand to avoid having his hand writing being recognized, so he uses a magazine to create a ransom note. We need to find out, given the ransom string and magazine string, is it possible to create a given ransom note. The kidnapper can use individual characters of words. Here is how your program should work to simulate the ransom problem: your program should prompt the user to enter a long String to represent the magazine and another short String to represent the required ransom note. your program needs to check if the magazine string contains all required characters in equal or greater number present in the ransom note. your program should print true if it is possible to create the given ransom note from the given magazine, and print false otherwise. Break up your code into a set of well-defined functions. Each function should include a comment block that briefly describes…arrow_forward(Python) Numerous engineering and scientific applications require finding solutions to a set of equations. Ex: 8x + 7y = 38 and 3x - 5y = -1 have a solution x = 3, y = 2. Given integer coefficients of two linear equations with variables x and y, use brute force to find an integer solution for x and y in the range -10 to 10. Ex: If the input is: 8 7 38 3 -5 -1 Then the output is: x = 3 , y = 2 Use this brute force approach: For every value of x from -10 to 10 For every value of y from -10 to 10 Check if the current x and y satisfy both equations. If so, output the solution, and finish. Ex: If no solution is found, output: "There is no solution" You can assume the two equations have no more than one solution. ''' Read in first equation, ax + by = c '''a = int(input())b = int(input())c = int(input()) ''' Read in second equation, dx + ey = f '''d = int(input())e = int(input())f = int(input())arrow_forward
- (a) Assume that five generation units with third order cost function (F: (P) = A: P²³ + B; P; ² + C; P; +D;) are in the circuit. Write a computer program using any arbitrary programming language (MATLAB, C++, C#, Python,...) to calculate economic. load dispatch (ELD) using first order gradient method. Note that all parameters and variables should be defined inside the program (at first lines) such that units' characteristics and demand can be changed easily. Neglect grid losses.arrow_forwardHelp me please :( Write a Python program where a non-intrinsic function solves for all the roots of a cubic polynomial given by the mathematical function ?(?)=??^3+??a^2+??+? where ?,?,? and ? are nonzero real numbers given by the user. Afterwards, the program will also solve for the sum and product of these roots. You must be able to create a function for getting the sum, and another for the product and use it in your main program. (HINT: Use Cardano’s formula).arrow_forwardProblem 6 The factorial n! of a positive number (integer) is defined by n! = n · (n – 1) · (n – 2 ) ·.….. 3. 2·1, where 0! = 1. Write a user-defined function that calculates the factorial n! of a number. For function name and arguments, use y=fact(x), where the input argument x is the number whose factorial is to be calculated and the output argument y is the value x!. The function displays an error message if a negative or non-integer number is entered when the function is called. Do not use MATLAB built-in function factorial. Use fact with the following numbers: (а) 9! (b) 8.5! (c) 0! (d) -5! *Note: Name the file B000000_P6_fact.marrow_forward
- (Wattan Corporation) is an Internet service provider that charges customers a flat rate of $7.99 for up to 10 hours of connection time. Additional hours or partial hours are charged at $1.99 each.Write a function charges that computes the total charge for a customer based on the number of hours of connection time used in a month. The function should also calculate the average cost per hour of the time used (rounded to the nearest 0.01), so use two output parameters to send back these results.You should write a second functionround_money that takes a real number as an input argument and returns as the function value the number rounded to two decimal places. Write a main function that takes data from an input file usage.txt and produces an output file charges.txt. The data file format is as follows:Line 1: current month and year as two integersOther lines: customer number (a five-digit number) and number of hours usedHere is a sample data file and the corresponding output file:Data file…arrow_forward(Wattan Corporation) is an Internet service provider that charges customers a flat rate of $7.99 for up to 10 hours of connection time. Additional hours or partial hours are charged at $1.99 each. Write a function charges that computes the total charge for a customer based on the number of hours of connection time used in a month. The function should also calculate the average cost per hour of the time used (rounded to the nearest 0.01), so use two output parameters to send back these results. You should write a second function round_money that takes a real number as an input argument and returns as the function value the number rounded to two decimal places. Write a main function that takes data from an input file usage.txt and produces an output file charges.txt. The data file format is as follows: Line 1: current month and year as two integers Other lines: customer number (a five-digit number) and number of hours used Here is a sample data file and the corresponding output file:…arrow_forwardProblem 4 (Searching a list of Student objects)Write a function called find_by_ID(students, id) that takes a list of student objects(students) and an int (id) as parameters, and returns the student object in the list withthe given id. If there is no student with the given id, it returns “NA”.See the sample output below. Looking for a student with ID = 123: Student: Alice, ID# 123 found Looking for a student with ID = 999: Student: Cathy, ID# 567 foundLooking for a student with ID = 555: NA foundarrow_forward
- The programming language: C++ The union of two ordered lists (Sequential linear list)[the solution introduction: The first video of in 3.1, 35:00-43:00] [Problem description] Give the union of two ordered lists. The maximal number of elements in an inputted set is 30. [Basic requirements] 1) Use sequential linear list. 2) The result list should also be ordered. [Example] Problem: Give the union of the ordered lists (3,4,9,100,103) and (7,9,43,53,102,105). What you need to show in the terminal(the back part is outputted by you and the blue part is inputted by the user, i.e., teacher): Please input the first ordered list: (3,4,9,100,103)Please input the second ordered list: (7,9,43,53,102,105) The union is: (3,4,7,9,9,43,53,100,102,103,105)arrow_forwardComputer Science c++ || urgent Write the code for choosing the list of items in order to get the maximum profit of fractional knapsack problem. [NOTE: number of input items should be user interest, and output should be like - firstly print the resulting profit table, and then print the all selected items (must not be all items from the inputted items - means that all inputted items should not be as the selected items) for the knapsack and then print the maximum profit.]arrow_forward7. [10] (Display Pattern) Write a C++ function called pattern that displays a pattern of stars and numbers for a given number of columns (passed as a parameter). Maximize your use of repetition (with nested for statements) and minimize the number of cout statements. Your function should then display a pattern of the appropriate size. Write a test program (main) that generates a random number in the range of 1 to 20 (inclusive) to specify the number of columns in the pattern. Use pattern to display the pattern with this random number as the number of columns. Here are two samples: With 3 as the input: With 5 as the input: 1 1 *2 *2 **3 **3 *2 ***4 1 ****5 ***4 **3 *2 1arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
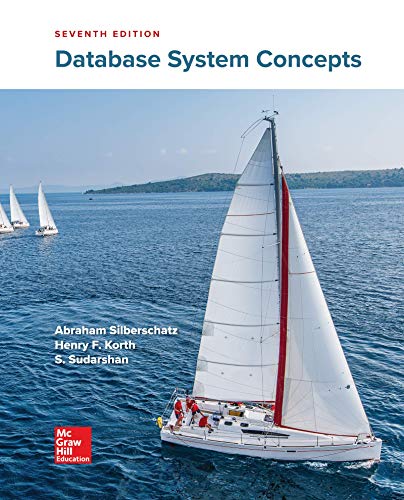
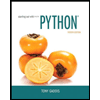
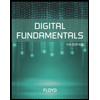
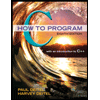
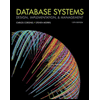
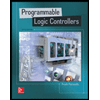