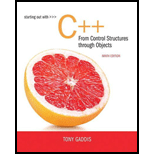
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 10PC
Program Plan Intro
MaxNode function
Program Plan:
“Main.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the main () function.
- Declare the necessary variables.
- Insert the values into the list.
- Displays to user that the values are inserted.
- Call the method “maximumnode()” to find the largest element of the list.
- The largest valued node gets displayed.
“NumberList.h”:
- Define the template function necessary.
- Define the class “NumberList”.
- Declare the necessary structure variables.
- Define the constructor and destructor.
- Declare the necessary function prototype.
“NumberList.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the method named “nodeappend()”,
- Declare the necessary variables
- Validate the list using if statement to find the status of the list.
- Use loop to iterate for the contents present in the list and add node to the last of the list.
- Define the method named “viewlist()”,
- Declare the necessary variables.
- Use loop that iterates to display the elements present in the list.
- Define the method named “insertval()”,
- Declare the necessary variables.
- Validate the content of the list using if statement.
- After evaluation of the list the contents the values are inserted at the desired location.
- Loop is used to iterate for the values that are present, to validate whether the particular element is greater or lesser than the elements present in the list.
- After evaluation the value is inserted at the desired location.
- Define the method named “removenode()”,
- Declare the necessary variables.
- Validate the list using if statement in order to position the list based on the elements that is deleted from the list.
- Define the method named “~NumberList()”,
- Declare the necessary variables.
- Loop that iterates to delete the memory allocates.
- Define the method named “sortlist()”,
- Declare the necessary variables.
- Use loop to iterate for the value of the list.
- A condition statement to compare the elements of the list and return the sorted list.
- Define the method named “mergeval()”,
- Loop that is used to iterate to merge the values of the list based on the list values.
- Call the function “sortlist()” to sort the value after being merged.
- Define the method named “maximumnode()”,
- Return the head node to be the maximum node.
- Defined the method named “maxNode()”,
- Use a condition statement to validate the elements of the list to identify the maximum value that is present in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Exercise, maxCylinderVolume
F# system function such as min or methods in the list module such as List.map are not allowed
Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0.
Examples:
> maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304
7. Dynamic MathStack The MathStack class shown in this chapter has only two member functions: add and sub. Write the following additional member functions: Function Description mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack. div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack. addAll Pops all values off the stack, adds them, and pushes their sum onto the stack. multA11 Pops all values off the stack, multiplies them, and pushes their prod- uct onto the stack. Demonstrate the class with a driver program.
here is the extention file please use it.
// Specification file for the IntStack class
#ifndef INTSTACK_H
#define INTSTACK_H
class IntStack
{
private:
int *stackArray; // Pointer to the stack array
int stackSize; // The stack size
int top; // Indicates the top of the stack
public:
// Constructor
IntStack(int);
// Copy constructor…
Count dominators
def count_dominators(items):
An element of items is said to be a dominator if every element to its right (not just the one element that is immediately to its right) is strictly smaller than it. By this definition, the last item of the list is automatically a dominator. This function should count how many elements in items are dominators, and return that count. For example, dominators of [42, 7, 12, 9, 13, 5] would be the elements 42, 13 and 5.
Before starting to write code for this function, you should consult the parable of "Shlemiel the painter" and think how this seemingly silly tale from a simpler time relates to today's computational problems performed on lists, strings and other sequences. This problem will be the first of many that you will encounter during and after this course to illustrate the important principle of using only one loop to achieve in a tiny fraction of time the same end result that Shlemiel achieves with two nested loops. Your workload…
Chapter 20 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 20.2 - What happens if a recursive function never...Ch. 20.2 - What is a recursive functions base case?Ch. 20.2 - Prob. 20.3CPCh. 20.2 - What is the difference between direct and indirect...Ch. 20 - What is the base case of each of the recursive...Ch. 20 - What type of recursive function do you think would...Ch. 20 - Which repetition approach is less efficient, a...Ch. 20 - When should you choose a recursive algorithm over...Ch. 20 - Explain what is likely to happen when a recursive...Ch. 20 - The _____________ of recursion is the number of...
Ch. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Write a recursive function to return the number of...Ch. 20 - Write a recursive function to return the largest...Ch. 20 - #include iostream using namespace std; int...Ch. 20 - Prob. 13RQECh. 20 - #include iostream #include string using namespace...Ch. 20 - Iterative Factorial Write an iterative version...Ch. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Recursive Array Sum Write a function that accepts...Ch. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PCCh. 20 - Prob. 9PCCh. 20 - Prob. 10PCCh. 20 - Prob. 11PCCh. 20 - Ackermanns Function Ackermanns Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Data structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects.2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject)3) Write a program that thoroughly tests all the methods in the class "AList".4) Include comments to all source code.arrow_forwardWrite JAVA code General Problem Description: It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below. Requirements: Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.arrow_forwardC++ ProgrammingActivity: Deque Linked List Explain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { DLLDeque* deque = new DLLDeque(); int test; cin >> test; //Declarations int tempnum; int num; int sum; int count; bool addfirst = false; bool addlast = false; bool remfirst = false; bool remlast = false; switch (test) { case 0: //Implementation do{ cin >> num; if(num == 0){ break; } //Reset tempnum = num; count = 0; sum = 0; addfirst = false; addlast = false; remfirst = false; remlast =…arrow_forward
- True or False The objects of a class can be stored in an array, but not in a List.arrow_forwardWhen the following program is executed, it terminates with an error. Which option best describes the cause of this error? Question options: The function will terminate the program due to invalid syntax. The function will terminate the program due to it accessing an invalid list index. The function will terminate the program due to the recursive limit being reached. The function will terminate the program due to a type mismatch when evaluating an operator. The function will terminate the program due to an invalid type conversion. None of these optionsarrow_forwardTerm by CodeChum Admin (JAVA CODE) Construct a class called Term. It is going to represent a term in polynomial expression. It has an integer coefficient and an exponent. In this case, there is only 1 independent variable that is 'x'. There should be two operations for the Term: public Term times(Term t) - multiplies the term with another term and returns the result public String toString() - prints the coefficient followed by "x^" and appended by the exponent. But with the following additional rules: if the coefficient is 1, then it is not printed. if the exponent is 1, then it is not printed ( the caret is not printed as well) if the exponent is 0, then only the coefficient is printed. Input The first line contains the coefficient and the exponent of the first term. The second line contains the coefficient and the exponent of the second term. 1·1 4·3 Output Display the resulting product for each of the test case. 4x^4arrow_forward
- Use the template below: def createList(n): #Base Case/s #ToDo: Add conditions here for base case/s #if <condition> : #return <value> #Recursive Case/s #ToDo: Add conditions here for your recursive case/s #else: #return <operation and recursive call> #remove the line after this once all ToDo is completed return [] def removeMultiples(x, arr): #Base Case/s #TODO: Add conditions here for your base case/s #if <condition> : #return <value> #Recursive Case/s #TODO: Add conditions here for your recursive case/s #else: #return <operation and recursive call> #remove the line after this once you've completed all ToDo return [] def Sieve_of_Eratosthenes(list): #Base Case/s if len(list) < 1 : return list #Recursive Case/s else: return [list[0]] + Sieve_of_Eratosthenes(removeMultiples(list[0], list[1:])) if __name__ == "__main__": n = int(input("Enter n: "))…arrow_forwardSalesforce Assignment: You are working in company as a junior developer and the manger assign you a task to create the test class of trigger code of the following code will cover all the use case like insert, update, delete. The code is as follows: public class TriggerBasicAssignmentTriggerHelper ( // This method is used to update the vlaue of field C public static void getFiledvalue(List listofValues, Map mapofCoustomobject ){ for (Trigger_Basic_Assignments_ct :listofvalues){ if(mapofCoustomobject == null || t.Field_A_c != mapofCoustomobject.get(t.Id). Field_A_C || t.Field_8_c != mapofCoustomobject.get(t.I if(t.Operator_ '+') t.Field C_c = t.Field_A_c + t.Field_B_c; else if(t.Operator_c *') t. Field C_c = t.Field A_* t.Field_8_c; else if(t.Operator_c == /' && t.Field B_c != 0) t.Field C_c = t.Field A_c / t.Field_B_c; else t.Field C_c = t.Field_A_c - t.Field B_c; I need the test class only.arrow_forwardC++ function Linked list Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.arrow_forward
- Reference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forwarddetemine if the following statement are true or false The iterator operation is required by the Iterable interface. A list allows retrieval of information based on the contents of the information.arrow_forward5. List Member Deletion Modify the list class you created in the previous programming challenges by adding a function to remove an item from the ist and by adding a destructor: void zemove (double x) : Linkedtist (02 Test the class by adding a sequence of instructions that mixes operations for adding items, removing items, and printing the list.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
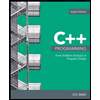
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
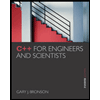
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr