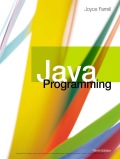
Explanation of Solution
Program code:
MadLib.java
//import the required packages
import java.io.*;
//define a class MadLib
class MadLib
{
//define a method intro()
private static void intro()
{
//create a string variable and initialize it
String msg = "This is a Mad-Lib game. I will prompt " +
"you for five words.\n";
//print the string
System.out.print(msg);
}
//define a method getInput()
private static String getInput(String msg) throws IOException
{
//create the object of BufferReader
BufferedReader stdin;
//initialize the object
stdin = new BufferedReader(new InputStreamReader(System.in));
//print the string msg
System.out.print(msg);
//return the line readed
return stdin.readLine();
}
//define a method printOutput
private static void printOutput (String noun1, String adverb, String noun2, String pronoun, String adj)
{
//create a string output and set the value
String output = "\nThree blind " + noun1 + ", \nThree blind " + noun1 + ", \nsee " + adverb +
" they run!" + "\nsee " + adverb + " they run!\nThey all ran after the farmer's " + noun2 +
"\nWho cut off their tails,\nWith a carving knife.\nDid you ever see " +
pronoun + " a thing in your life,\nAs three " + adj + " mice.";
//print output
System.out.println(output);
}
//define a main() method
public static void main(String[] args) throws IOException
{
//call the method intro()
intro();
//get the value for noun1
String noun1 = getInput("Enter a noun : ");
//get the value for adverb
String adverb = getInput("Enter a adverb : ");
//get the value for noun2
String noun2 = getInput("Enter a another noun : ");
//get the value for pronoun
String pronoun = getInput("Enter a pronoun : ");
//get the value for adj
String adj = getInput("Enter an adjective : ");
//print the accepted values
printOutput(noun1, adverb, noun2, pronoun, adj);
}
}
Explanation:
The above snippet of code is used get the noun, adverb, pronoun and adjective from the user and creates the sentences using it...

Trending nowThis is a popular solution!

Chapter 2 Solutions
EBK JAVA PROGRAMMING
- A "mad-lib" is a fill-in-the blank game. One player writes a short story in which some words are replacedby blanks. For each word that is removed, the appropriate part of speech is noted: e.g. noun (person/-place/thing), adjective (word that describes a noun), verb (an action, e.g. eat), adverb (modifies a verb, e.g.quickly). Then, before reading the story, the story-writer asks the other player to write down a word of theappropriate part of speech for each blank without knowing the context in which it will be used. In this way,a humorous (sometimes) or non-sensical (usually) story is created a)arrow_forwardAGU Computer Engineering Department created a new mathematical game that can be played with two people. In this game, you are given a list of random integers. At each round, the first player takes two numbers from the list and calculates the sum of these numbers. Then, the second player takes two numbers and makes the same calculation. The higher one is going to take the round and increment the score by 1. At the end of the game, who has the better score will win the game. Here are the rules for the game: There are always an even number of items on the list There are two colors for the players: Green and Red Green starts the game At each round, green plays and then red plays The player takes the first and the last numbers The game can end Tie when the scores are the same. The game will end when there is no element to take from the list Your program will simulate the rounds and find out the winner, Green or Red or Tie. The input will be two lines. Input Format…arrow_forwardAGU Computer Engineering Department created a new mathematical game that can be played with two people. In this game, you are given a list of random integers. At each round, the first player takes two numbers from the list and calculates the sum of these numbers. Then, the second player takes two numbers and makes the same calculation. The higher one is going to take the round and increment the score by 1. At the end of the game, who has the better score will win the game. Here are the rules for the game: There are always an even number of items on the list There are two colors for the players: Green and Red Green starts the game At each round, green plays and then red plays The player takes the first and the last numbers The game can end Tie when the scores are the same. The game will end when there is no element to take from the list Your program on java will simulate the rounds and find out the winner, Green or Red or Tie. The input will be two lines. Input…arrow_forward
- In c Hangman gameWrite a terminal based on the game of Hangman. In a hangman game, the player is given a wordthat they need to guess, with each letter of the word represented by an underscore/blank. Theplayer tries to guess a letter of the word by entering it into the terminal. If the letter is correct, theblank corresponding to that letter is filled in. If the letter is incorrect, a part of a stick figure isdrawn. The player has a limited number of incorrect guesses before the stick figure is fully drawnand the game is lost. The player wins the game if they guess all the letters of the word before thestick figure is fully drawn.The program should have an array of possible words to choose from. A different word should berandomly chosen from the array every time we run the program.arrow_forwardIn this game, one flyand three frogs are placed randomly on a board with the size 7x7.In each iteration, they move randomlywithin the board, and the simulation stops when one of the frogs eats the fly.Fly and frogs are essentially creatures that can move, make noise, and eat. Frogs can move up to 2squares in any direction, and flies can move up to 1. Frogs make the "Croak! Croak!" sound, and fliesmake "ZzzZZz!". Since frogs should not eat their kind, a function of "isEatable" should also beimplemented in decision making. A variable or function to check if the fly is alive is also required as aterminate condition for simulation.In each iteration, an 'f' character represents frogs' position on board, and an '*' character is used torepresent the position of fly.arrow_forwardIn this game, one flyand three frogs are placed randomly on a board with the size 7x7.In each iteration, they move randomlywithin the board, and the simulation stops when one of the frogs eats the fly.Fly and frogs are essentially creatures that can move, make noise, and eat. Frogs can move up to 2squares in any direction, and flies can move up to 1. Frogs make the "Croak! Croak!" sound, and fliesmake "ZzzZZz!". Since frogs should not eat their kind, a function of "isEatable" should also beimplemented in decision making. A variable or function to check if the fly is alive is also required as aterminate condition for simulation.In each iteration, an 'f' character represents frogs' position on board, and an '*' character is used torepresent the position of fly. Java language please I dont want the survival game starting with the code below I want in Java language and like in photos please #functions def GenerateRandomScene(): scenes = ['Riverside','Top of the Mountain','Middle of…arrow_forward
- In this game, one flyand three frogs are placed randomly on a board with the size 7x7.In each iteration, they move randomlywithin the board, and the simulation stops when one of the frogs eats the fly.Fly and frogs are essentially creatures that can move, make noise, and eat. Frogs can move up to 2squares in any direction, and flies can move up to 1. Frogs make the "Croak! Croak!" sound, and fliesmake "ZzzZZz!". Since frogs should not eat their kind, a function of "isEatable" should also beimplemented in decision making. A variable or function to check if the fly is alive is also required as aterminate condition for simulation.In each iteration, an 'f' character represents frogs' position on board, and an '*' character is used torepresent the position of fly. Java Language THIS QUESTION HAS BEEN ANSWERED BUT THE ANSWERS GIVEN ARE IRRELEVANTarrow_forwardIn this game, one flyand three frogs are placed randomly on a board with the size 7x7.In each iteration, they move randomlywithin the board, and the simulation stops when one of the frogs eats the fly.Fly and frogs are essentially creatures that can move, make noise, and eat. Frogs can move up to 2squares in any direction, and flies can move up to 1. Frogs make the "Croak! Croak!" sound, and fliesmake "ZzzZZz!". Since frogs should not eat their kind, a function of "isEatable" should also beimplemented in decision making. A variable or function to check if the fly is alive is also required as aterminate condition for simulation.In each iteration, an 'f' character represents frogs' position on board, and an '*' character is used torepresent the position of fly. I dont want the survival game starting with the code below I want in Java language and like in photos please #functions def GenerateRandomScene(): scenes = ['Riverside','Top of the Mountain','Middle of Forest','Mountain…arrow_forwardIn this game, one flyand three frogs are placed randomly on a board with the size 7x7.In each iteration, they move randomlywithin the board, and the simulation stops when one of the frogs eats the fly.Fly and frogs are essentially creatures that can move, make noise, and eat. Frogs can move up to 2squares in any direction, and flies can move up to 1. Frogs make the "Croak! Croak!" sound, and fliesmake "ZzzZZz!". Since frogs should not eat their kind, a function of "isEatable" should also beimplemented in decision making. A variable or function to check if the fly is alive is also required as aterminate condition for simulation.In each iteration, an 'f' character represents frogs' position on board, and an '*' character is used torepresent the position of fly. Java Language I dont want the survival game that answer of my last two questions I want the above question and example output like in the photosarrow_forward
- Java program help Simulate a slot machine. The customer will add a fixed amount of money to the machine and play the slots. There should be 4 images for the game: "Apple", "Orange", "Banana", and "Cherry". If three of the images match, the customer wins $2 and is added to their balance. If all four match, the customer wins $10 and that is added to their balance. If less then four match there is no prize money, and the customer is charged $1 for the try. The customer can quit after each try, and the program will print out the customer's cash balance. Coding Details: Use an array of strings to store the 4 strings listed above. Use a do-while loop for the program. The program starts once the user enters how much money they have. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for the program is shown below: Determine the fruits to display (step 3 below) and print them Determine if there…arrow_forwardNim is a two-player game played with several piles of stones. You can use as many piles and as many stones in each pile as you want, but in order to better understand the game, we'll start off with just a few small piles of stones (see figure 1 below). Pile 1 Pile 1 Pile 2 The two players take turns removing stones from the game. On each turn, the player removing stones can only take stones from one pile, but they can remove as many stones from that pile as they want (please note, a player must remove atleast 1 stone from a pile during his/her turn). If they want, they can even remove the entire pile from the game! The winner is the player who removes the final stone (avoid taking the last stone - see figure 2 below). Pile 2 Pile 3 Pile 3 Let's say its Max (player 1) turn to play. Then Max can win by simply removing a stone from Pile 2 or Pile 3 Draw a game tree (upto depth level 2) for the given version of the Nim game. Please consider figure 1 as your initial game configuration/state…arrow_forwardA Memory Matching Game in java code with a 4x4 grid of Squares that when you click on a square it shows a number. It would have to be 2 of each number 1-8 and if the two squares that are clicked match then the squares stay on the grid. If they do not match after picking 2 squares they flip back overarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
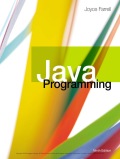
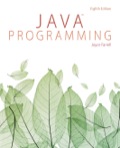