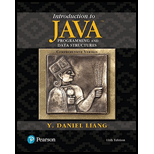
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 19.2PE
Program Plan Intro
Implement “GenericStack” using inheritance
Program Plan:
- Include appropriate package “import java.util.Scanner” into program.
- Define the class named “Exercise19_02”.
- Define main method.
- Define the object “obj” for “GenericStack<String>” class.
- Assign the object for “Scanner” class.
- Prompt the user and get the strings from user and push the values into stack using “for” loop.
- Using “for” loop, print array elements in reverse order.
- Define the class “GenericStack<E>” which extends the “ArrayList<E>” class.
- Define the Boolean method “isEmpty()” which returns empty value.
- Define the Integer method “getSize()” which returns size of the stack.
- Define the method “peek()” which returns top element from the stack.
- Define the method “pop()” which returns stack elements.
- Define the method “push()” which inserts the values into stack.
- Define the method “search()” which searches the index position of the stack.
- Define the method “toString()” which prints the stack elements.
- Define main method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please answer the following question in Python code:
Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!)
Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top.
push() – take an item as input and push it on the top of the stack
pop() – remove and return the item at the top of the stack
isEmpty() – returns True if the stack is empty, False otherwise
[] – return the item at a given…
(Please Help. My professor did not teach us any of this and will not answer my emails)
Design a class named Queue for storing integers. Like a stack, a queue holds elements. In a stack, the elements are retreived in a last-in-first-out fashion. In a queue, the elements are retrieved in a first-in-first-out fashion. The class contains:
An int[] data field named elements that stores the int values in the queue
A data field named size that stores the number of elements in the queue
A constructor that creates a Queue object with defult capacity 8
The method enqueue(int v) that adds v into the queue
The method empty () that returns true if the queue is empty
The method getSize() that returns the size of the queue
(Sort ArrayList)
Write the following method that sorts an ArrayList:
public static <E extends Comparable<E>> void sort(ArrayList<E> list)
Write a test program that prompts the user to enter 10 integers, invokes this method to sort the numbers, and displays the numbers in increasing order.
Sample Run
Enter 10 integers: 3 4 12 7 3 4 5 6 4 7
The sorted numbers are 3 3 4 4 4 5 6 7 7 12
Class Name: Exercise19_09
Chapter 19 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 19.2 - Are there any compile errors in (a) and (b)?Ch. 19.2 - Prob. 19.2.2CPCh. 19.2 - Prob. 19.2.3CPCh. 19.3 - Prob. 19.3.1CPCh. 19.3 - Prob. 19.3.2CPCh. 19.3 - Prob. 19.3.3CPCh. 19.3 - Prob. 19.3.4CPCh. 19.4 - Prob. 19.4.1CPCh. 19.4 - Prob. 19.4.2CPCh. 19.5 - Prob. 19.5.1CP
Ch. 19.5 - Prob. 19.5.2CPCh. 19.6 - What is a raw type? Why is a raw type unsafe? Why...Ch. 19.6 - Prob. 19.6.2CPCh. 19.7 - Prob. 19.7.1CPCh. 19.7 - Prob. 19.7.2CPCh. 19.7 - Prob. 19.7.3CPCh. 19.7 - Prob. 19.7.4CPCh. 19.8 - Prob. 19.8.1CPCh. 19.8 - Prob. 19.8.2CPCh. 19.8 - Prob. 19.8.3CPCh. 19.8 - Prob. 19.8.4CPCh. 19.8 - Prob. 19.8.5CPCh. 19.9 - Prob. 19.9.1CPCh. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - How are the add, multiple, and zero methods...Ch. 19.9 - What would be wrong if the printResult method is...Ch. 19 - (Revising Listing 19.1) Revise the GenericStack...Ch. 19 - Prob. 19.2PECh. 19 - (Distinct elements in ArrayList) Write the...Ch. 19 - Prob. 19.4PECh. 19 - (Maximum element in an array) Implement the...Ch. 19 - (Maximum element in a two-dimensional array) Write...Ch. 19 - Prob. 19.7PECh. 19 - (Shuffle ArrayList) Write the following method...Ch. 19 - (Sort ArrayList) Write the following method that...Ch. 19 - (Largest element in an ArrayList) Write the...Ch. 19 - Prob. 19.11PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Find the nonleaves) Java Define a new class named BSTWithNumberOfNonLeaves that extends BST with the following methods: /** Return the number of nonleaf nodes */public int getNumberofNonLeaves() // BEGIN REVEL SUBMISSION class BSTWithNumberOfNonLeaves<E> extends BST<E> { /** Create a default BST with a natural order comparator */ public BSTWithNumberOfNonLeaves() { super(); } /** Create a BST with a specified comparator */ public BSTWithNumberOfNonLeaves(java.util.Comparator<E> c) { super(c); } /** Create a binary tree from an array of objects */ public BSTWithNumberOfNonLeaves(E[] objects) { super(objects); } public int getNumberOfNonLeaves() { return getNumberOfNonLeaves(root); } /** Returns the number of non-leaf nodes */ private int getNumberOfNonLeaves(TreeNode<E> root) { // WRITE YOUR CODE HERE } } // END REVEL SUBMISSIONarrow_forwardPlease use Python for this question: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty, False otherwise [] – return the item at a given location, [0] is at…arrow_forwardPlease use Python for this question: 1. Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using inheritance. Note that this means you may be able to inherit some of the methods below: a. Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. b. push() – take an item as input and push it on the top of the stack c. pop() – remove and return the item at the top of the stack d. isEmpty() – returns True if the stack is empty, False otherwise e. [] – return the item at a given location, [0] is at the bottom of the stack f.…arrow_forward
- (YOU ARE NOT ALLOWED TO USE ARRAYLIST IN THIS PROJECT)Write a Java program to simulate a blackjack game of cards. The computer will play the role of the dealer. The program will randomly generate the cards dealt to the player and dealer during the game. Cards in this game will be represented by numbers 1 to 13 with Ace being represented by a 1. Remember, that face cards (i.e. Jack, Queen, and King) are worth 10 points to a hand while an Ace can be worth 1 or 11 points depending on the user’s choice. The numbered cards are worth their number value to the hand.arrow_forward(see image for code) Consider the following code. Replace the tags _??1_ and _??2_, respectively, by filling in the text fields below, such that running printGenericCollection(c) prints the contents of the Collection object c the console:arrow_forward(True/False): Arrays are passed by reference to avoid copying them onto the stackarrow_forward
- (Implement set operations in MyList)(JAVA) Please use class name: Exercise_01 The implementations of the methods addAll, removeAll, retainAll, toArray(), and toArray(T[]) are omitted in the MyList interface. Implement these methods. Test your new MyList class using the code at https://liveexample.pearsoncmg.com/test/Exercise24_01.txt. Sample Output: Enter five strings for array name1 separated by space: TomGeorgePeterJeanJaneEnter five strings for array name2 separated by space: TomGeorgeMichaelMichelleDanielEnter two strings for array name3 separated by space: TomPeterlist1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After addAll:[Tom, George, Peter, Jean, Jane, Tom, George, Michael, Michelle, Daniel] list1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After removeAll:[Peter, Jean, Jane] list1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After retainAll:[Tom, George] list1:[Tom, George,…arrow_forward5. Now examine the for-loops in the above Java program. (assume that we have the have replaced the array with an ArrayList) a) Which ArrayList method should you use to control the for-loop iterations? b) Explain why the first for-loop cannot be controlled by that ArrayList method (but the other two for-loops can). Here is a quick review of how array elements are modified and accessed: 1. public class BasicArray{ 2. public static void main(String[] args) int[] basic = new int[4]; for (int i=0; iarrow_forwardC# (Generic Method IsEqualTo) Write a simple generic version of method IsEqualTo that compares its two arguments with the Equals method, and returns true if they’re equal and false otherwise. Use this generic method in a program that calls IsEqualTo with a variety of simple types, such as object or int. What result do you get when you attempt to run this program?arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
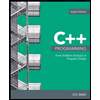
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning