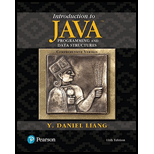
Factorial
- Import required packages
- Declare the main class named “Main”.
- Give the main method “public static void main ()”
- Create an object “sc” for the scanner class.
- Get a number from the user and store it in a variable “numberString”.
- Create an object “bigNum” for the static method “BigInteger”.
- Print the output.
- Give function definition for the static method “factorial ()”.
- Check if the value is equal to zero.
- Return 1.
- Else,
- Call the function “factorial ()” recursively until the result is found.
- Check if the value is equal to zero.
- Give the main method “public static void main ()”

The below program is used to find the factorial for the given number using “BigInteger” class.
Explanation of Solution
Program:
//Import required packages
import java.math.*;
import java.util.*;
//Class name
public class Main
{
//Main method
public static void main(String[] args)
{
//Create an object for Scanner
Scanner sc = new Scanner(System.in);
//Print the message
System.out.print("Enter an integer of any size: ");
//Get the string from the user
String numberString = sc.nextLine();
//Create an object for the static method
BigInteger bigNum = new BigInteger(numberString);
//Print the output
System.out.println("Factorial of " + bigNum + " is " + factorial(bigNum));
}
//Static method "factorial"
public static BigInteger factorial(BigInteger value)
{
//Check if the value is equal to zero
if (value.equals(BigInteger.ZERO))
//Return 1
return BigInteger.ONE;
//Else
else
/*Call the function recursively to till the result is found*/
return value.multiply(factorial(value.subtract(BigInteger.ONE)));
}
}
Enter an integer of any size: 50
Factorial of 50 is 30414093201713378043612608166064768844377641568960512000000000000
Want to see more full solutions like this?
Chapter 18 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
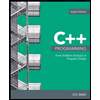