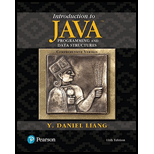
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 18, Problem 18.13PE
(Find the largest number in an array) Write a recursive method that returns the largest integer in an array. Write a test
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Recursive Power MethodWrite a method called powCal that uses recursion to raise a number to a power. The method should accept two arguments: The first argument is the exponent and the second argument is the number to be raised (example” powCal(10,2) means 210). Assume that the exponent is a nonnegative integer. Demonstrate the method in a program called Recursive
(This means that you need to write a program that has at least two methods: main and powCal. The powCal method is where you implement the requirements above and the main method is where you make a method call to demonstrate how your powCal method work).
Write a recursive function that displays the number of even and odd digits in an integer using the following header:
void evenAndOddCount(int value)
Write a test program that prompts the user to enter an integer and displays the number of even and odd digits in it.
Q1: Write a program to accomplish the following tasks:- a) Write a method to print the ArrayList in the reverse order b) Write a method to sort the ArrayList, and display the data after sorting c) Write a method to print the largest and the smallest element in the ArrayList d) In the main method ask user to enter 10 integers, store them in the ArrayList, pass the ArrayList to above methods, and then display the result
Chapter 18 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 18.2 - What is a recursive method? What is an infinite...Ch. 18.2 - Prob. 18.2.2CPCh. 18.2 - Show the output of the following programs and...Ch. 18.2 - Prob. 18.2.4CPCh. 18.2 - Prob. 18.2.5CPCh. 18.2 - Write a recursive mathematical definition for...Ch. 18.3 - Prob. 18.3.1CPCh. 18.3 - What is wrong in the following methods?Ch. 18.3 - Prob. 18.3.3CPCh. 18.4 - Describe the characteristics of recursive methods.
Ch. 18.4 - Prob. 18.4.2CPCh. 18.4 - Prob. 18.4.3CPCh. 18.5 - Prob. 18.5.1CPCh. 18.5 - Prob. 18.5.2CPCh. 18.5 - What is a recursive helper method?Ch. 18.6 - Prob. 18.6.1CPCh. 18.6 - How does the program get all files and directories...Ch. 18.6 - How many times will the getSize method be invoked...Ch. 18.6 - Will the program work if the directory is empty...Ch. 18.6 - Will the program work if line 20 is replaced by...Ch. 18.6 - Will the program work if lines 20 and 21 are...Ch. 18.7 - Prob. 18.7.1CPCh. 18.8 - Prob. 18.8.1CPCh. 18.8 - Prob. 18.8.2CPCh. 18.8 - How many times is the displayTriangles method...Ch. 18.8 - Prob. 18.8.4CPCh. 18.8 - Prob. 18.8.5CPCh. 18.9 - Which of the following statements are true? a. Any...Ch. 18.9 - Prob. 18.9.2CPCh. 18.10 - Identify tail-recursive methods in this chapter.Ch. 18.10 - Rewrite the fib method in Listing 18.2 using tail...Ch. 18 - Prob. 18.1PECh. 18 - Prob. 18.2PECh. 18 - (Compute greatest common divisor using recursion)...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Fibonacci series) Modify Listing 18.2,...Ch. 18 - Prob. 18.8PECh. 18 - (Print the characters in a string reversely) Write...Ch. 18 - (Occurrences of a specified character in a string)...Ch. 18 - Prob. 18.11PECh. 18 - (Print the characters in a string reversely)...Ch. 18 - (Find the largest number in an array) Write a...Ch. 18 - (Find the number of uppercase letters in a string)...Ch. 18 - Prob. 18.15PECh. 18 - (Find the number of uppercase letters in an array)...Ch. 18 - (Occurrences of a specified character in an array)...Ch. 18 - (Tower of Hanoi) Modify Listing 18.8,...Ch. 18 - Prob. 18.19PECh. 18 - (Display circles) Write a Java program that...Ch. 18 - (Decimal to binary) Write a recursive method that...Ch. 18 - (Decimal to hex) Write a recursive method that...Ch. 18 - (Binary to decimal) Write a recursive method that...Ch. 18 - (Hex to decimal) Write a recursive method that...Ch. 18 - Prob. 18.25PECh. 18 - (Create a maze) Write a program that will find a...Ch. 18 - (Koch snowflake fractal) The text presented the...Ch. 18 - (Nonrecursive directory size) Rewrite Listing...Ch. 18 - (Number of files in a directory) Write a program...Ch. 18 - (Game: Knights Tour) The Knights Tour is an...Ch. 18 - (Game: Knights Tour animation) Write a program for...Ch. 18 - (Game: Eight Queens) The Eight Queens problem is...Ch. 18 - Prob. 18.35PECh. 18 - (Sierpinski triangle) Write a program that lets...Ch. 18 - (Hilbert curve) The Hilbert curve, first described...Ch. 18 - (Recursive tree) Write a program to display a...Ch. 18 - Prob. 18.39PE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What is assembly language?
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Modify the temperature conversion program to print a heading above the table.
C Programming Language
The code that follows shows an example of branching on an enumerated type value in a switch statement. Recall t...
Computer Systems: A Programmer's Perspective (3rd Edition)
Look at the following string: cookiesmilkfudge:cake:ice cream a. Write code using a StringTokenizer object that...
Starting Out with Java: Early Objects (6th Edition)
Define each of the following terms: entity type entity-relationship model entity instance Attribute relationshi...
Modern Database Management
What is denormalization?
Database Concepts (7th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please solve it quickly and logical and correct solutionsarrow_forward1.Answer the following questions: 1(a) Write a method to compute and return the value of maxEven and minOdd where maxEven is the largest even element of an int array and minOdd is the smallest odd element of the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it. 1(b) Write a method to compute and return the value of countEven and countOdd where countEven is the number even elements in an int array and countOdd is the number of odd elements in the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it.arrow_forwardplease code in python Forbidden concepts: arrays/lists (data structures), recursion, custom classes You have been asked to take a small icon that appears on the screen of a smart telephone and scale it up so it looks bigger on a regular computer screen.The icon will be encoded as characters (x and *) in a 3 x 3 grid as follows: (refer image1 ) Write a program that accepts a positive integer scaling factor and outputs the scaled icon. A scaling factor of k means that each character is replaced by a k X k grid consisting only of that character. Input Specification:The input will be an integer such that 0 < k ≤ 10. Output Specification:The output will be 3k lines, which represent each individual line scaled by a factor of k and repeated k times. A line is scaled by a factor of k by replacing each character in the line with k copies of the character. [refer image2]arrow_forward
- Q1: Write a program to accomplish the following tasks:- a) Write a method to print the ArrayList in the reverse orderb) Write a method to sort the ArrayList, and display the data after sortingc) Write a method to print the largest and the smallest element in the ArrayListd) In the main method ask user to enter 10 integers, store them in the ArrayList, pass the ArrayList to above methods, and then display the result after each call.arrow_forwardJava: the user enters array 3*4 print the max and min number, and their places in the array.arrow_forward(FYI: Pseudocode is required (Not any programming language) Design a pseudocode program that loads an array with the following 7 values. Add one more word (of your own choosing) for a total of 8 words. biffcomelyfezmottleperukebedraggledquisling Be sure to use lowercase, as shown above. This will make the processing easier. Ask the user to enter a word Search through this array until you find a match with the word the user entered. Once you find a match, output "Yes, that word is in the dictionary". If you get to the end of the array and do NOT find a match, output "No, that word is not in the dictionary". The program should work with any set of words in the arrays. If I were to change the words in the arrays, it should still work. If you need help, look at the search example in your textbook.arrow_forward
- Write statements to do the following: i. Write a loop that computes the sum of all elements in the array, A ii. Write a loop that finds the minimum element in the array, A iii. Write a method to find the maximum in the array, Aarrow_forwarddef winning_card(cards, trump=None): Playing cards are again represented as tuples of (rank,suit) as in the cardproblems.pylecture example program. In trick taking games such as whist or bridge, four players each play one card from their hand to the trick, committing to their play in clockwise order starting from the player who plays first into the trick. The winner of the trick is determined by the following rules:1. If one or more cards of the trump suit have been played to the trick, the trick is won by the highest ranking trump card, regardless of the other cards played.2. If no trump cards have been played to the trick, the trick is won by the highest card of the suit of the first card played to the trick. Cards of any other suits, regardless of their rank, are powerless to win that trick.3. Ace is the highest card in each suit.Note that the order in which the cards are played to the trick greatly affects the outcome of that trick, since the first card played in the trick…arrow_forwardQuestion 3 : (Eliminate duplicates) Write a program Duplicate.java that reads in ten integers in an array T, creates a new array V by eliminating the duplicate values in the array, and displays the result. Here is the sample run of the program: Enter ten numbers: 1 2 3 2 1 6 3 4 5 2 The distinct numbers are: 1 2 3 6 4 5arrow_forward
- Indicate true or false for the following statements: Every element in an array has the same type. The array size is fixed after it is declared. The array size declarator must be a constant expression. The array elements are initialized when an array is declared.arrow_forwardWrite method body of following methods: DeleteNum(int position) // This method will delete element from array at position entered by the user arrange the data after that position to left. (Using While loop) DeleteNum(int from, int to) // This method will delete elements from array at range given by the user, as the user inputs two positions i.e start and end, the program should than delete all numbers in that range. (Use C++ to code)arrow_forwardPls please solve it quicklyarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
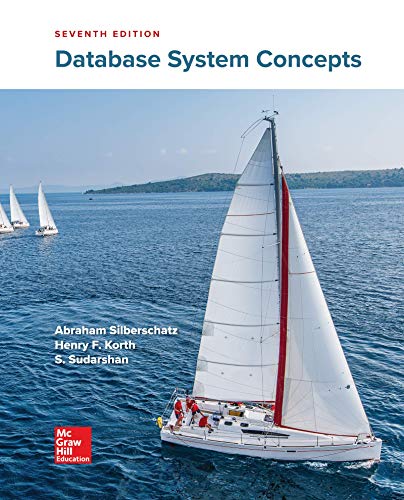
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
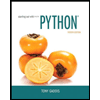
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
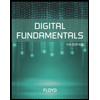
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
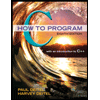
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
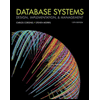
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
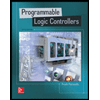
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License