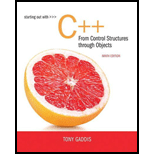
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 12PC
Program Plan Intro
Implementation of specialized templates
Program plan:
- Include the required header files in the program.
- Define the template class “SimpleVector”:
- Declare the required class data members and exception function under “private” access specifier.
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the specialized template “SimpleVector()” function:
- Get the array size and allocate the memory for corresponding array size
- Store the c-string values as the array elements.
- Define the specialized template copy constructor of the “SimpleVector()” function:
- Copy the array size and allocate the memory for corresponding array size
- copy the array elements.
- Define the specialized template “~SimpleVector” class destructor:
- Delete the allocated memory for the array.
- Define the specialized template “subError()” function:
- Display an error message.
- Exit from the program
- Define the specialized template function for operator overload for “[]”:
- Get the array subscript and check it is in the array range.
- If not then call the “subError()” exception function.
- Return the subscript.
- Define the template class “SearchableVector”, which is derived from the above class template “SimpleVector”:
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the specialized template copy constructor of the “SearchableVector()” function:
- Call the base class copy constructor for copying the array size and allocate the memory for corresponding array size.
- Copy the array elements.
- Define the specialized template function “findItem()”
- Declare the required variables in the function.
- Get the element from the function call.
- Using while loop
- Find the middle element of the array.
- Check the middle element is the searching element.
- If so, then return the array subscript.
- Else, check the middle element is greater than the searching element.
- If so, decrease the last index value.
- Else, increase the starting index value.
- If the element is not found, then return -1 as the array subscript.
- Define the main() function:
- Create an object for the class “SearchableVector” for c-string type.
- Using for loop, display the array on the output screen.
- Call the function “findItem()” using c-string element.
- Compare the result of the function and display the message according to the condition.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below.
Implement the following methods in arrayListType.h:
isEmpty
isFull
listSize
maxListSize
clearList
Copy constructor
Implement the following method in unorderedArrayListType.h
insertAt
Also, write a program (in main.cpp) to test your function.
In C++
The base class Pet has private data members petName, and petAge. The derived class Dog extends the Pet class and includes a private data member for dogBreed. Complete main() to:
create a generic pet and print information using PrintInfo().
create a Dog pet, use PrintInfo() to print information, and add a statement to print the dog's breed using the GetBreed() function.
Ex. If the input is:
Dobby2Kreacher3German Schnauzer
the output is:
Pet Information: Name: Dobby Age: 2Pet Information: Name: Kreacher Age: 3 Breed: German Schnauzer
Task 4. Utility classes
Implement a Menu-Driven program of ArrayList class using the above scenario and perform the
following operations.
a. Create an object of ArrayList class based on the scenario with explanations of the method used.
b. Create a method that will allow inserting an element to ArrayList Class based on the scenario. The
input field must be validated as necessary.
C.
Create a method to delete specific elements in the ArrayList with explanations of the method used.
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Classes, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forwardc++ student instruction1. SimpleVector Generic Template2. SearchableVector Generic Template3. SimpleVectorInterface Generic Template4. Soccer Class implemented with MyString 5. Implementation of operators.6. Use three files in your implementation.Description of the problem:1. Use the Soccer class described in Gaddis chapter 11 number 6 page 6522. Implement the operators in your code, and make the code modifications discussed in class, to create a one-dimensional array composed of instances of type Soccer and show your results in solving the following problems:to. (Gaddis) Programming Challenger 9. SearchableVectorModification page 1023 Chapter 16b. (Gaddis) Programming Challenger 10. SortableVector ClassTemplate Modification page 1023 Chap16. order with himSelection Sort algorithm p. 474 Chap. 8=============================================================== Soccer Scores class infoWrite a program that stores the following data about a soccer player in a structure: Player’s Name…arrow_forwardData Structure & Algorithum java program Do the following: 1) Add a constructor to the class "LList" that creates a list from a given array of objects.2) Add a method "addAll" to the "LList" class that adds an array of items to the end of the list. The header of the method is as follows, where "T" is the generic type of the objects in the list. 3) Write a Test/Driver program that thoroughly tests all the methods in the class "LList".arrow_forward
- Unordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forwardUsing C++ Without Using linked lists: Create a class AccessPoint with the following: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overLap that checks if two access points overlap their coverage and returns true if they do. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Represent this with bars like, IIIII. Each bar can represent 20% Test your class by writing a main function that…arrow_forwardAlert dont submit AI generated answer.arrow_forward
- Procedure: Develop an extension of structure.Vector, called MyVector, that includes a new method, sort. Here are some steps toward implementing this new class: 1. Create a new class, MyVector, which is declared to be an extension of the structure.Vector class. You should write a default constructor for this class that simply calls super();. This will force the structure.Vector constructor to be called. This, in turn, will initialize the protected fields of the Vector class. 2. Construct a new Vector method called sort. It should have the following declaration: public void sort(Comparator c) // pre: c is a valid comparator // post: sorts this vector in order determined by c This method uses a Comparator type object to actually perform a sort of the values in MyVector. You may use any sort that you like. 3. Write an application that reads in a data file with several fields, and, dependingarrow_forwardGiven a template class named indexList, the prototype of one of its member function is as follows: bool replace (int index, const T &item); When implementing, what is the prototype (the first two lines only) of the replace function? The body of the function is not needed. A B I EE % $5 3arrow_forwardThis is in c++ The base class Pet has private data members petName, and petAge. The derived class Dog extends the Pet class and includes a private data member for dogBreed. Complete main() to: create a generic pet and print information using PrintInfo(). create a Dog pet, use PrintInfo() to print information, and add a statement to print the dog's breed using the GetBreed() function. main.cpp #include <iostream> #include<string> #include "Dog.h" using namespace std; int main() { string petName, dogName, dogBreed; int petAge, dogAge; Pet myPet; Dog myDog; getline(cin, petName); cin >> petAge; cin.ignore(); getline(cin, dogName); cin >> dogAge; cin.ignore(); getline(cin, dogBreed); // TODO: Create generic pet (using petName, petAge) and then call PrintInfo // TODO: Create dog pet (using dogName, dogAge, dogBreed) and then call PrintInfo // TODO: Use GetBreed(), to output the breed of the dog } Dog.cpp #include "Dog.h" #include…arrow_forward
- THIS QUESTION IS RELATED TO SUBJECT OBJECT ORIENTED PROGRAMMING IN C++ . SOLVE this question in dev c++. please send it in 30 minutes and solve step by step. THANKS Q#5 Start with the safearay class from the ARROVER3 program in Chapter 8 of Object Orient programming by Rober Lafore. Make this class into a template, so the safe array can store any kind of data. Include following member functions in Safe array class. The minimum function finds the minimum value in array. The maximum function find the maximum value in array. The average function find average of all the elements of an array. The total function finds the running total of all elements of an array. In main(), create safe arrays of at least two different types int and double and store some data in them. Then display minimum, maximum, average and total of array elements. Note: use subscript ([]) operator in sasfearay class.arrow_forwardPlease Solve the Question in C++ as quickly as you can in 40 minutes. And do the same as asked in the question. Don't use extra things, please. Q1: Start with the safearay class from the ARROVER3 program in Chapter 8 of Object Orient programming by Rober Lafore. Make this class into a template, so the safe array can store any kind of data. Include following member functions in Safe array class. The minimum function finds the minimum value in array. The maximum function find the maximum value in array. The average function find average of all the elements of an array. The total function finds the running total of all elements of an array. In main(), create safe arrays of at least two different types int and double and store some data in them. Then display minimum, maximum, average and total of array elements. Note: use subscript ([]) operator in sasfearay class.arrow_forwardJAVA CODEarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
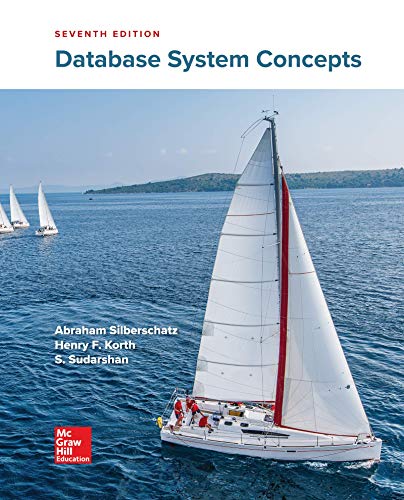
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
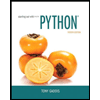
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
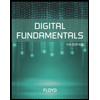
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
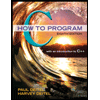
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
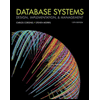
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
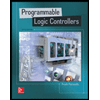
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education