What is a template?
A template in C++ programming helps to pass data type as a parameter to make the code reusable. The goal is to write a generic code that can be reused for specific types depending upon the need.
For instance, consider a program that needs to sort an array of different types. Instead of writing the same code multiple times, it is better to write for sorting once and pass data type as a parameter.
The keywords template and typename are used in C++ to declare templatized classes.
Fixing of templates at compile-time
Like macros, templates are expanded during compilation. In the case of templates, the compiler performs type checking before expanding it. Ideally, the source code will have only a function or class, but the compiled code will have several copies of the same function or class.
Types of templates
C++ offers two types of templates namely,
- Class templates
- Function templates
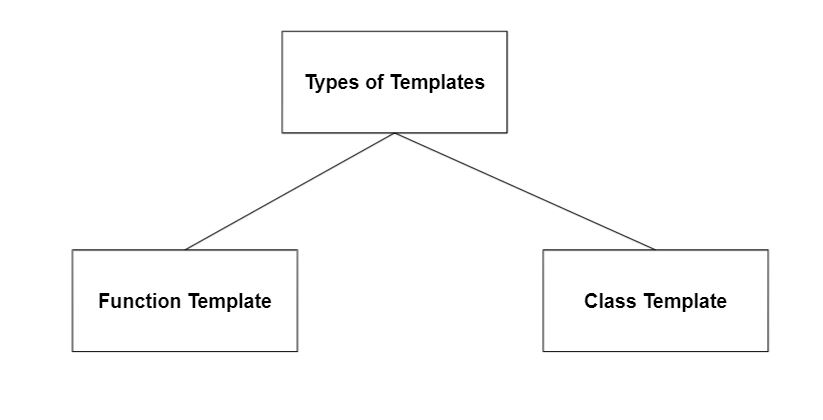
Function templates
Function templates are used like regular functions. The difference is that they are preceded with the keyword “template”. Function templates are used to perform similar operations for each data type. For instance, a programmer can write one function definition and make use of it for performing operations on parameters of different types.
Using template functions
If a template function is used in a code, the compiler will instantiate the template function when it sees it. Following is an example of how the function template program can be used:
#include<iostream>
using namespace std;
// max will return the maximum number
template<class T>
T max(T a, T b) {
return a > b ? a:b;
}
void main(){
cout<<”max(10,15) = “<< max(10,15) << endl;
cout <<”max(‘k’,’s’) = “ << max(‘k’,’s’) << endl;
cout << "max(10.1, 15.2) = " << max(10.1, 15.2) << endl;
}
Explanation
The above template function code is written to find the maximum of the two parameters passed to it. The template function is declared with a keyword template. The prototype of the max function indicates that it takes two arguments ‘a’ and ‘b’ of type T. The same function is applied to arguments of type int, char, and double inside the main function. The value returned by the function is displayed on the console.
Output
max(10,15) = 15
max(‘k’,’s’) = s
max(10.1, 15.2) = 15.2
Class templates
Class templates are similar to function templates. They are used when a class defines an independent data type. Class templates help to implement data structures such as LinkedList, BinaryTree, Stack, and Queue.
Example of class templates
Below is an example of class template implementation.
// Class template
template <class T>
class Number {
private:
// Variable of type T
T num;
public:
Number(T n) : num(n) {} // constructor
T getNum() {
return num;
}
};
int main() {
// create object with int type
Number<int> numberInt(7);
// create object with double type
Number<double> numberDouble(7.7);
cout << "int Number = " << numberInt.getNum() << endl;
cout << "double Number = " << numberDouble.getNum() << endl;
return 0;
}
Explanation
In the above program, a class template named Number has been created. It consists of a private variable num, constructor, and the function getNum(). The variable num, constructor argument, and function have type T. This shows that they can have any data type. In the main function, the template class is instantiated by specifying the actual type namely, int and double. When the code is executed, the class definition for int and double types are created accordingly.
Output
int Number = 7
double Number = 7.7
Template instantiation
When a compiler creates a class, function, or static data member from a template, it is called template instantiation.
- A generated class is a class generated from the class template.
- A generated function is a function generated from the function template.
Types of template instantiation
There are two types of template instantiation, namely,
- Explicit instantiation
- Implicit instantiation
Explicit instantiation
In explicit instantiation, the compiler is explicitly instructed to generate a definition from the template. Below is the syntax for explicit instantiation
template template_declaration
An example of explicit instantiation is:
template <class T>
class Y {
public:
Y() {};
~Y() {};
void h() {};
void m() {};
};
int main() {
template class Y<int>;
template class Y<float>;
return 0;
}
Explanation: After executing the above code, the compiler will explicitly create an instantiation for class Y<int> and class Y<float>
Implicit instantiation
If a template specialization is not instantiated explicitly or explicitly specialized, the compiler will create a template specialization only when it needs the definition. This type of instantiation is implicit instantiation.
Consider the following implicit template instantiation code for finding the maximum of two numbers:
Template <class T>
T minimum(T a, T b)
{
return a < b ? a : b ;
}
void main()
{
int n;
n = min(10, 15) ; //implicit instantiation of max(int, int)
}
Explanation: When this code is executed the compiler will create a function min(int, int) and return the minimum of the two numbers. In this case, the output will be 10.
Context and Applications
Templates are an essential concept for programmers, especially those working with C++ programming. The topic is covered in courses like:
- Web programming certification courses
- Master of Science in Software Programming
- Bachelor of Computer Science Engineering
Practice Problems
Q1) What is a template in programming?
- A template is a method for creating generic classes and functions
- It contains an introduction email, contact information, and introduction letter
- It is a free feature for creating introduction attributes
- It is a function used to introduce and start a class
Answer: Option a
Explanation: A template is a method for creating a generic class. It creates reusable source code.
Q2) Is it possible to have overloading in function templates?
- Yes
- No
- Sometimes
- Nothing can be said
Answer: Option a
Explanation: Function templates can be overloaded.
Q3) Which of the following are used for generic programming?
- Non-template compiling modules
- Start strings
- Templates
- Free constructor
Answer: Option c
Explanation: Templates are used for generic programming. They help in creating generic functions and generic classes.
Q4) Which of the following keywords are used in a template?
- class
- typename
- introduction, work, and contact information
- Both a and b
Answer: Option d
Explanation: The keywords class and typename are used in templates in the following manner:
template <class T> function declaration;
template <typename T> function declaration;
Q5) What is a template parameter?
- It is used to pass a type as an argument
- It is used to introduce or type an introduction email
- It can be an introduction letter
- It is used to introduce free type in an introduction email
Answer: Option a
Explanation: A template parameter is a special parameter used to pass a type as an argument.
Common Mistakes
Students need to remember that templates in C++ programming also accept non-type arguments. That means it is possible to pass non-type parameters to templates. However, they must be constant because the compiler has to know the value of the template parameters at the time of compilation.
Related Concepts
- Templates and static variables in C++
- The C++ standard template library (STL)
- Classes and Objects in C++
- Fundamentals of C++ programming
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Introduction to Template Homework Questions from Fellow Students
Browse our recently answered Introduction to Template homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.