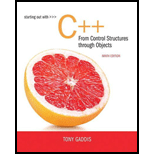
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 15, Problem 57RQE
class Tank : public Cylinder
{
private:
int fuelType;
double gallons;
public:
Tank();
~Tank();
void setContents(double);
void setContents(double);
};
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Change the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized.
The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position
public class Labyrinth { private final int size; private final Cell[][] grid;
public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); }
private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…
Change the following code so that it checks the following 3 conditions:
1. there is no space between each cells (imgs)
2. even if it is resized, the components wouldn't disappear
3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game.
Main():
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9);
JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true);
public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…
Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane.
The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units.
Cell Class:
public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…
Chapter 15 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 15.1 - Here is the first line of a class declaration....Ch. 15.1 - What is the name of the derived class in the...Ch. 15.1 - Suppose a program has the following class...Ch. 15.2 - What is the difference between private members and...Ch. 15.2 - What is the difference between member access...Ch. 15.2 - Suppose a program has the following class...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.7 - Explain the difference between overloading a...Ch. 15.7 - Prob. 15.10CP
Ch. 15.7 - Prob. 15.11CPCh. 15.7 - What will the following program display? #include...Ch. 15.7 - What will the following program display? #include...Ch. 15.7 - What will the following program display? #include...Ch. 15.7 - What will the following program display? #include...Ch. 15.8 - Does the following diagram depict multiple...Ch. 15.8 - Does the following diagram depict multiple...Ch. 15.8 - Examine the following classes. The table lists the...Ch. 15.8 - Examine the following class declarations: class...Ch. 15 - What is an is a relationship?Ch. 15 - A program uses two classes: Dog and Poodle. Which...Ch. 15 - How does base class access specification differ...Ch. 15 - What is the difference between a protected class...Ch. 15 - Can a derived class ever directly access the...Ch. 15 - Which constructor is called first, that of the...Ch. 15 - What is the difference between redefining a base...Ch. 15 - Prob. 8RQECh. 15 - What is an abstract base class?Ch. 15 - A program has a class Potato, which is derived...Ch. 15 - What base class is named in the line below?class...Ch. 15 - What derived class is named in the line below?...Ch. 15 - What is the class access specification of the base...Ch. 15 - What is the class access specification of the base...Ch. 15 - Protected members of a base class are like...Ch. 15 - Complete the table on the next page by filling in...Ch. 15 - Complete the table below by filling in private,...Ch. 15 - Complete the table below by filling in private,...Ch. 15 - A derived class inherits the ________ of its base...Ch. 15 - When both a base class and a derived class have...Ch. 15 - An overridden base class function may be called by...Ch. 15 - When a derived class redefines a function in a...Ch. 15 - A(n) __________ member function in a base class...Ch. 15 - ________ binding is when the compiler binds member...Ch. 15 - __________ binding is when a function call is...Ch. 15 - _________ is when member functions in a class...Ch. 15 - When a pointer to a base class is made to point to...Ch. 15 - A(n) __________ class cannot be instantiated.Ch. 15 - A(n) _______ function has no body, or definition,...Ch. 15 - A(n) _________ of inheritance is where one class...Ch. 15 - _______ is where a derived class has two or more...Ch. 15 - In multiple inheritance, the derived class should...Ch. 15 - Write the first line of the declaration for a...Ch. 15 - Write the first line of the declaration for a...Ch. 15 - Suppose a class named Tiger is derived from both...Ch. 15 - Write the declaration for class B. The classs...Ch. 15 - T F The base classs access specification affects...Ch. 15 - T F The base classs access specification affects...Ch. 15 - T F Private members of a private base class become...Ch. 15 - T F Public members of a private base class become...Ch. 15 - T F Protected members of a private base class...Ch. 15 - T F Public members of a protected base class...Ch. 15 - T F Private members of a protected base class...Ch. 15 - T F Protected members of a public base class...Ch. 15 - T F The base class constructor is called after the...Ch. 15 - T F The base class destructor is called after the...Ch. 15 - T F It isnt possible for a base class to have more...Ch. 15 - T F Arguments are passed to the base class...Ch. 15 - T F A member function of a derived class may not...Ch. 15 - Prob. 51RQECh. 15 - T F A base class may not be derived from another...Ch. 15 - class Car, public Vehicle { public: Car(); Car();...Ch. 15 - class Truck, public : Vehicle, protected {...Ch. 15 - class SnowMobile : Vehicle { protected: int...Ch. 15 - class Table : public Furniture { protected: int...Ch. 15 - class Tank : public Cylinder { private: int...Ch. 15 - class Three : public Two : public One { protected:...Ch. 15 - Employee and ProductionWorker Classes Design a...Ch. 15 - ShiftSupervisor Class In a particular factory, a...Ch. 15 - TeamLeader Class In a particular factory, a team...Ch. 15 - Prob. 4PCCh. 15 - Time Clock Design a class named TimeClock. The...Ch. 15 - Essay Class Design an Essay class that is derived...Ch. 15 - PersonData and CustoraerData Classes Design a...Ch. 15 - PreferredCustomer Class A retail store has a...Ch. 15 - File Filter A file filter reads an input file,...Ch. 15 - File Double-Spacer Create a derived class of the...Ch. 15 - Course Grades In a course, a teacher gives the...Ch. 15 - Ship. CruiseShip, and CargoShip Classes Design a...Ch. 15 - Pure Abstract Base Class Project Define a pure...Ch. 15 - Prob. 14PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
The Upper West View Yacht club sponsors sailboat races every weekend for its fleet of SuperGee sailboats, and w...
Starting Out With Visual Basic (8th Edition)
This will happen if you try to use an index that is out of range for a list a. A valuError exception will occur...
Starting Out with Python (4th Edition)
What will the following code output? int apples = 0, bananas = 2, pears =10; apples += 10; bananas =10; pears /...
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
In Exercises 57 through 64, identify any errors. ConstnAsInteger=5n+=1txtOutput.Text=CStrn
Introduction To Programming Using Visual Basic (11th Edition)
Determine the horizontal and vertical components of reaction at the pin A and the reaction of the rocker B on t...
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Suppose a multiprogramming operating system allocated time slices of 10 milliseconds and the machine executed a...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Discuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forwardMake the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forward
- Please original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forward
- How do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
- Operating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardArtificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
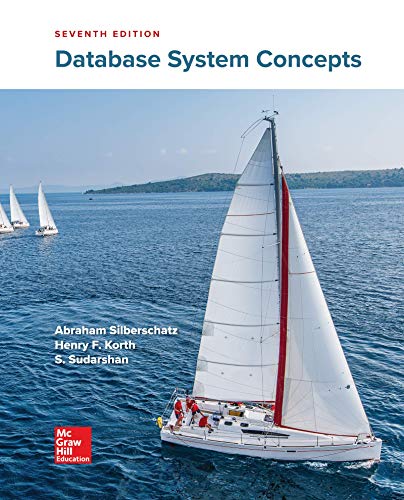
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
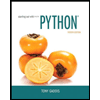
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
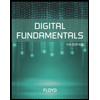
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
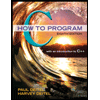
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
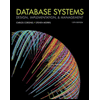
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
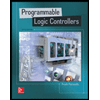
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY