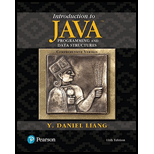
Concept explainers
a.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
}
//Finally block
finally
{
statement4;
}
statement5;
b.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
}
//Finally block
finally
{
statement4;
}
statement5;
c.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
throw ex2;
}
//Finally block
finally
{
statement4;
}
statement5;
d.
Finally block:
Finally block contains block of code and the block is executed after a try-catch block.
- If there is no errors in try block, finally statement is executed after try block executed.
- If there is error in try block, then catch block caught the exception, rest of try block is skipped, and then executes the finally statement.
- If there is error in try block, then catch block does not caught that exception, rest of try block is skipped, executes only finally statement, and then skip the rest of the code.
- Thus, finally block handle such case and it contains block of statement. Once try-catch block gets executed, finally block is executed.
Rethrowing the exception:
- An exception can be rethrown by a catch statement because; it can catch and handled by another catch statement.
- It allows many handlers to access the exception.
- However, the rethrown exception should not recaught by the same exception and should propagate to next catch statement.
In following class, first catch statement catches exception and rethrows it to another catch statement:
class Rethrow_exp
{
try
{
//... Try block statement
}
Catch statement rethrows the exception.
catch(Excep_type excep_obj)
{
//... Catch block statement
// Rethrow the exception
throw excep_obj
}
//...
}
Catch statement catches the rethrown the exception.
class rethrow_main
{
//...
catch(Excep_typeexcep_obj)
{
// Recatch block statement catches rethrown //exception
}
}
Therefore, an inner catch rethrow an exception to an outer catch.
Given code:
//Try block
try
{
statement1;
statement2;
statement3;
}
//Catch block
catch (Exception1 ex1)
{
}
//Catch block
catch (Exception2 ex2)
{
throw ex2;
}
//Finally block
finally
{
statement4;
}
statement5;

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- The following program simulates a vending machine panel. The program gets an integer that represents the user's selected item, and then outputs a dispensing message. Only 8 items are for purchase. Ex: The input 2 results in the message "Dispensing item 2". Arrange the following lines to handle two exceptions. If the user enters an item number that is not for purchase, the program throws an Exception with the message "Try again", outputs the exception message, and then tries again. If the user enters a non-integer input, the program throws an InputMismatchException, outputs "Fatal error", and then exits. Not all lines are used in the solution.arrow_forwardThis lab will exercise your understanding of some of the concepts covered in Chapter 14: exception handling 1. Create a program that uses EXCEPTION HANDLING to deal with an invalid input entry by a user. a. Write a program that prompts a user to enter a length in feet and inches. The length valuesmust be positive integers. b. Calculate and output the equivalent measurement in centimeters1. inch = 2.54 centimeters c. Write the code to handle the following exceptions: If the user enters a negative number, throw and catch an error that gives the user the appropriate message and request a valid number. Do this until the user enters a valid number If the user enters a character, throw and catch an error that indicates the input stream is invalid. Write code that resets the input stream and request a valid number. Do this until the user enters a valid number d. Output the feet-inch input values to centimeter conversion value The program may be named any name of our choice, must have a .cpp…arrow_forwardPLZ help with the following IN JAVA Suppose that statement2 causes an exception in the following try-catch block: Will statement3 be executed? If the exception is not caught, will statement4 be executed? If the exception is caught in the catch block, will statement4 be executed?arrow_forward
- Which of the following statements is false? A software developer can write his own exceptions in Java. A try statement is associated with one and only one catch statement.. A programmer written Exception implements the Exception interface. None of the above statements are falsearrow_forwardJAVA Given a main program that searches for the ID or the name of a student from a text file, complete the findID() and the findName() methods that return the corresponding information of a student. Then, insert a try/catch statement in main() to catch any exceptions thrown by findID() or findName(), and output the exception message. Each line in the text file contains the name and the ID of a student, separated by a space. Method findID() takes two parameters, a student's name and a Scanner object containing the text file's contents. Method findID() returns the ID associated with the student's name if the name is in the file, otherwise the method throws an Exception object with the message "Student ID not found for studentName", where studentName is the name of the student. Method findName() takes two parameters, a student's ID and a Scanner object containing the text file's contents. Method findName() returns the name associated with the student's ID if the ID is in the file,…arrow_forwardWhat is exception propagation? Give an example of a class that contains at least two methods, in which one method calls another. Ensure that the subordinate method will call a predefined Java method that can throw a checked exception. The subordinate method should not catch the exception. Explain how exception propagation will occur in your example.arrow_forward
- Write a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. (in Python)arrow_forwardWrite a program that reads integers user_num and div_num as input, and output the quotient (user_num divided by div_num). Use a try block to perform all the statements. Use an except block to catch any ZeroDivisionError as a variable and output "Zero Division Exception: " followed by the exception message from the variable. Use another except block to catch any ValueError caused by invalid input as a variable and output "Input Exception: " followed by the exception message from the variable. Note: ZeroDivisionError is raised when a division by zero happens. ValueError is raised when a user enters a value of different data type than what is defined in the program. Do not include code to raise any exception in the program. Ex: If the input of the program is: 15 3 the output of the program is: 5 Ex: If the input of the program is: 10 0 the output of the program is: Zero Division Exception: integer division or modulo by zero Ex: If the input of the program is: 15.5 5 the output of…arrow_forwardWrite a program that demonstrates how various exceptions are caught with catch (Exception exception) Define classes ExceptionA (which inherits from class Exception) and ExceptionB(which inherits from class ExceptionA). In your program, create try blocks that throw exceptions of types ExceptionA, ExceptionB, NullPointerException and IOException. All exceptions should be caught with catch blocks specifying type Exception.arrow_forward
- Given the following code, the output is __. try { Integer number = new Integer("1"); System.out.println("An Integer instance."); } catch (Exception e) { System.out.println("An Exception."); } Group of answer choices A- An Exception. B- 1 C- Error: Exception D- An Integer instance. E- None of the optionsarrow_forwardcould you include a try-catch statement that calls the GetYear function defined above. If a BadYear exception is thrown, print an error message and rethrow the exception to a caller; otherwise, execution should just continue as normal.arrow_forwardQ# When JUnit testing with exceptions, which of the following is true? Group of answer choices A successful test happens whenever an exception is not thrown. Exceptions and tests should not be used together. A failed test results when you catch an exception on valid input. If you give an illegal argument to a method the JUnit test will fail. Q# When you want a method to return nothing, what do you put for the return type? Group of answer choices boolean false void null Q# Which of the following statements are true? Group of answer choices All of the options are true. Methods can take multiple primitives as arguments. Methods can take objects of other classes as arguments. A method for a class can take an object of that class as an argument. Q# What does Java guarantee is part of every class? Group of answer choices AtoString() A set of Getter methods to retrieve class variables. A method to get the binary representation of the class. A set of private class…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
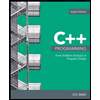
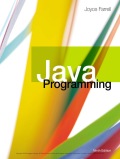
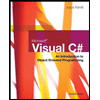