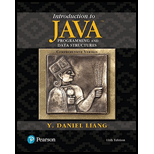
Concept explainers
(a)
Exception handling:
In Java, the exception is an object used to signal the unexpected error occurred during a program execution.
- Exception is the error, which arises at run time and such errors can be handled in Java by exception handling.
Runtime exception:
- The runtime exceptions are indicated in RuntimeException class that defines the errors in programs such as out of bounds array, numeric errors, and so on.
- Some of runtime exceptions such as,
- ArithmeticException
- NullPointerException
- IndexOutOfBoundsException
- IllegalArgumentException
(b)
Explanation of Solution
Output of code:
The given code is,
//Class definition
class Test
{
// Main function
public static void main(String args[])
{
//Declare the array variable
int[] list = new int[5];
//Display the result
System.out.println(list[5]);
}
}
Explanation:
In the above code,
- In t...
(c)
Explanation of Solution
Output of code:
The given code is,
//Class definition
class Test
{
// Main function
public static void main(String args[])
{
//Declare the string variable
String s = "abc";
//Display the result
System.out.println(s.charAt(3));
}
}
Explanation:
In the above code,
- In the main function,
- Declare and i...
(d)
Explanation of Solution
Output of code:
The given code is,
//Class definition
class Test
{
// Main function
public static void main(String args[])
{
//Create the object for Object class
Object o = new Object();
//Initialize the object to string variable
String d = (String)o;
}
}
Explanation:
In the above ...
(e)
Explanation of Solution
Output of code:
The given code is,
//Class definition
class Test
{
// Main function
public static void main(String args[])
{
//Create the object for Object class
Object o = null;
//Convert "o" into string and then display it
System.out.println(o...
(f)
Explanation of Solution
Output of code:
The given code is,
//Class definition
class Test
{
// Main function
public static void main(String args[])
{
//Divide the 1.0 by 0 and then display the result
System.out.println(1.0/0);
}
}
Explanation:
In the above...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- INSTRUCTIONS: Write a C++ script/code to do the given problem. Write a class called profileID, which has data members firstName,lastName, age, birthday and ageCompany (the age when you entered in the company). Remember to use set and get methods to access the data members this time. Also, write another class called bonusAward that has a method getBonus()with arguments yos and etype which are both integer data types. This method calculates the bonus received by an employee using this formula: yos*1000 + etype*1000. Write two derived classes, ASE and SE, using multiple inheritance usingprofileID and bonusAward as parent classes. Both classes must have amethod named getyos()that returns the yos of an employee using the formula age–ageCompany; and a method named getetype() which returns a constant based on the employee type. For ASE, the constant is 10 while for SE that constant is 20. Last, write a main program where you instantiate ASE as employee1 and SE as employee2. Get their…arrow_forwardIn this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forwardPLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forward
- with python do whis: Edit or delete a user profileWhen the user chooses 2, the first thing that it should do is to check whether the user information is loaded in the program (i.e., check if the user information is passed to the function that generates recipe recommendations). If the user information is passed to the function (i.e., the user chose option 1 before choosing option 2), the program should show the user the following menu:Hello (user name)You can perform one of the following operations:1) Delete your profile2) Edit your profilea. If the user chooses 1, perform the following subtasks to delete a user profile:1- Search for the user profile in the file userInformation.txt using the user name in read mode; once you find the user profile (i.e., the line that contains all the user information), pass it to a function that deletes the user information.2- The function should create a temporary file called temp.txt in write mode and search the file userInformation.txt in read mode…arrow_forwardBasic Java Help Each year, the Social Security Administration provides a list of baby names for boy and girls by state. You will write a program to read a file containing a list of baby names and display the top names. To start, implement the following class: baby names util = printTopNames(File, int): void printTopNames Will be a static method. It will accept two parameters, one is a File containing the list of names. The other is an integer that indicates how many names to display (ie, top 10 names, or top 50 names, etc). The integer argument could be any valid integer. You do not have to worry about an integer value large than 5 for the boy/girl names. This method should open the file and parse the file and print the names as shown below: Your program will use the Scanner class to read the data from the file (see your text for examples). You will also need to use a delimiter with the scanner so that the scanner will break the line apart based on the commas. For this file, set…arrow_forwardWhich statements correctly describes libraries? For each of the following statements, select Yes if the statement is true. Otherwise, select No. -A game library could include common operations for scoring lives, a leaderboard, and physics, engine to use in coding a game. -A disadvantage of libraries is that code cannot be reused in more than one script. -Libraries restrict the sharing of code by limiting the number of events in a program.arrow_forward
- If an exception is raised and the program does not handle it with a try/exceptstatement, what happens?arrow_forwardSuppose an exception is thrown in a function. What are the three things the function can do?arrow_forwardWhen is it preferable to use Exception over if-else when dealing with error/invalid situations?in the language Javaarrow_forward
- Friend functions increase Programming Bugs?arrow_forwardCreate a flowchart and modify the code. INSTRUCTION: Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another.Example: 20 mod 3 equals 2Because 20/3 = 6 with a remainder of 2 CODE TO COPY: #include <iostream> using namespace std; class Calculator{public:Calculator(){printf("Welcome to my Calculator\n"); } int addition(int a, int b);int subtraction(int a, int b);int multiplication(int a, int b);float division(int a, int b);}; int Calculator::addition(int a, int b){return (a+b);} int Calculator::subtraction(int a, int b){return (a-b);} int…arrow_forward*CODE SHOULD BE IN JAVA* A personal phone directory contains room for first names and phone numbers for 30 people. Assign names and phone numbers for the first 10 people. Prompt the user for a name, and if the name is found in the list, display the corresponding phone number. If the name is not found in the list, prompt the user for a phone number, and add the new name and phone number to the list. Continue to prompt the user for names until the user enters quit. After the arrays are full (containing 30 names), do not allow the user to add new entries. Use the following names and phone numbers: Name Phone # Gina (847) 341-0912 Marcia (847) 341-2392 Rita (847) 354-0654 Jennifer (414) 234-0912 Fred (414) 435-6567 Neil (608) 123-0904 Judy (608) 435-0434 Arlene (608) 123-0312 LaWanda (920) 787-9813 Deepak (930) 412-0991arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
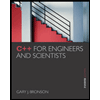