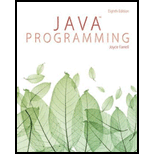
Java Programming (MindTap Course List)
8th Edition
ISBN: 9781285856919
Author: Joyce Farrell
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 12, Problem 6PE
Program Plan Intro
Display course and appropriate message
Program plan:
Filename: “CourseException.java”
- Define “CourseException” class which extends from “Exception” class
- Define the constructor
- Call the super method.
- Define the constructor
Filename: “Course.java”
- Define the “Course” class
- Declare the required variables and set the values
- Define the default constructor
- Set the values
- Define the parameterized constructor
- Set the values
- Check the length of the department
- Append the string to the “msg” variable
- Check the “num” value is less than “LOW_NUM” or greater than “HIGH_NUM”
- Append the string to the “msg” variable
- Check “msg” is not equal to empty string.
- Throw an exception.
- Define “toString” method
- Return the value
Filename: “ThrowCourseException.java”
- Define the class “ThrowCourseException”
- Define the “main” method
- Create an object for “Course” class
- Declare the variables and set the required values to the variables
- Iterate “for” loop until it reaches length of the “course”
- Set the values to the object
- Iterate “for” loop until it reaches length of the “course”
- In “try” block, set the values
- In “catch” block, display the course and error
- Display the course values
- Define the “main” method
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
In Java
Attached
Java question
Write a Thermostat class such that a user of the Thermostat class can create an objectof Thermostat and set it to the desired temperature within a pre-specified range. If theuser tries set the temperature outside this range it should throw a TemperatureTooHighor TemperatureTooLow exception. Use inheritance to create an exception superclassTemperatureOutofRange and subclasses TemperatureTooHigh andTemperatureTooLow.Sample Tester code:Thermostat t = new Thermostat(0, 100);t. setTemp(50); // Should be OK.t.setTemp(150); // Should throw TemperatureTooHigh exception.t.setTemp(-50); // Should throw TemperatureToolLow exception.Write a Tester class to demonstrate throwing and catching of exceptions. Show that thecatch specifying the superclass catches the subclass exceptions. The order of exceptionhandlers is important. If you try to catch a superclass exception type before a subclasstype, the compiler would generate errors. Also show the re-throwing of exceptions.
JAVA Problem – CycleFileInput
Revisit the Cycle class . Modify your application such that the properties will be read from a text file called “Cycle.txt”.
Directions
Examine your application for the class called Cycle.
Add an appropriate throws statement in the main method.
Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Note: Cycle.txt was created in the previous assignment, CycleFileOutput.
In your code, check that the text file does exist.
Input the values from the file to memory.
Close the file.
public class Cycle {
// Declear integer instance variable
private int numberOfWheels;
private int weight;
// Constructer declear class
public Cycle(int numberOfWheels, int weight ) {
this.numberOfWheels = numberOfWheels;
this.weight = weight;
}
// String method for output
public String toString() {
String wheel = String.valueOf(this.numberOfWheels);
String load = String.valueOf(this.weight);
return("No of wheels in cycle = " +…
Chapter 12 Solutions
Java Programming (MindTap Course List)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java Programming: Below is the lexer, shank and token files along with the shank.txt file. The shank file is the main method file. The lexer must break up the input text stream into lexemes and return a token object for each one in the shank.txt file. Make sure to fix the errors in the lexer.java file and show the complete code for lexer.java.There must be no error in the code at all. Run the whole code and show the output which the shank.txt must be printed out in the terminal. Attached is the rubric. Lexer.java package mypack; import java.util.HashMap;import java.util.List;import mypack.Token.TokenType; public class Lexer { private static final int INTEGER_STATE = 1;private static final int DECIMAL_STATE = 2;private static final int IDENTIFIER_STATE = 3;private static final int SYMBOL_STATE = 4;private static final int ERROR_STATE = 5;private static final int STRING_STATE = 6;private static final int CHAR_STATE = 7;private static final int COMMENT_STATE = 8; private static final…arrow_forwardWrite a program to create a class” Fan “to represents features of a fan as follows: “Fan “should contain following details fanType, manufacturer, model, isOn. Use constructor to initialize data members of the Fan class. Create function on() and off() to switch on and off the fan respectively. Create a function speedup() is to increase the speed of the fan by 1 if speed of the Fan greater than 5 it should throw a custom exception. Create another function speeddown() is to decrease the speed of the fan by 1 if speed of the Fan less than 0 it should throw a custom exception. Make a menu driven program.arrow_forwardProgramming Problem 3 – CycleFileInput Revisit the Cycle class in Module 3. Modify your application such that the properties will be read from a text file called “Cycle.txt”. Directions Examine your application for the class called Cycle. Add an appropriate throws statement in the main method. Create a reference to a File class with the appropriate name of a text file (Cycle.txt). Note: Cycle.txt was created in the previous assignment, CycleFileOutput. In your code, check that the text file does exist. Input the values from the file to memory. Close the file.arrow_forward
- Write an application TestStudent that prompts for and reads a student name. It then creates a Student object. It then prompts for and reads the number of quizzes n that the student has taken. It then prompts for and reads the scores of the student in n quizzes and adds each to the totalScore of the student using addQuiz() method. Finally, the application prints the student object using the toString() method, and it also prints his quiz average. The main method must handle java.util.InputMismatchException, java.lang.IllegalArgumentException and java.lang.Unsupported OperationException.arrow_forwardArgumentException is an existing class that derives from Exception; you use it when one or more of a method’s arguments do not fall within an expected range. Write the application SwimmingWaterTemperature containing a variable that can hold a temperature expressed in degrees Fahrenheit. Within the class, create a method that accepts a parameter for a water temperature and returns true or false, indicating whether the water temperature is between 70 and 85 degrees and thus comfortable for swimming. If the temperature is not between 32 and 212 (the freezing and boiling points of water), it is invalid, and the method should throw an ArgumentException. In the Main() method, continuously prompt the user for data temperature, pass it to the method, and then display the following messages indicating whether the temperature is comfortable, not comfortable, or invalid: X degrees is comfortable for swimming. X degrees is not comfortable for swimming. Value does not fall within the expected…arrow_forwardThis is the question - Create a UsedCarException class that extends Exception; its constructor receives a value for a vehicle identification number (VIN) that is passed to the parent constructor so it can be used in a getMessage() call. Create a UsedCar class with fields for VIN, make, year, mileage, and price. The UsedCar constructor throws a UsedCarException when the VIN is not four digits; when the make is not Ford, Honda, Toyota, Chrysler, or Other; when the year is not between 1997 and 2017 inclusive; or either the mileage or price is negative. Write an application that establishes an array of at least seven UsedCar objects and handles any Exceptions. Display a list of only the UsedCar objects that were constructed successfully. Here is the code I have - public class ThrowUsedCarException { public static void main(String[] args) { // Write your code here } } public class UsedCar { String vin; String make; int year; int mileage; int…arrow_forward
- Create a file with the name car.py to store information about a single Car Class. The Car object needs to include the following information: Car name Car vin number Car make Import the Car class into the main.py. Inside the main portion of the code, create a Car object, car1, that has the name "Car", vin "111", and the make "Honda". Print the car information to the commandline and make sure that your code is working correctly. Create a file with the name linkedlist.py. This file will contain the linked list class and the node class as well. The linkedlist.py file needs to import the Car class Create a class Node to represent a single node in the linked list. The class will be inside the linkedlist.py file. The Node class will include the following: A next pointer to hold the address of the next node. You need to set this variable to None A car variable to hold the car objects. Test your Node class in the main. Create a Car object car1. Set the name of the car, vin, and model Create a…arrow_forwardIn C#,arrow_forwardDescriptionIn this assignment, you are required to implement an electronic programming quiz system. User can createquestions and preview the quiz.Your TaskYou are asked to write a Java program for the programming quiz system. There are two types of questions:Multiple Choice Question and Ture/False Question. User can create questions using the system; andpreview the quiz, which display all questions in the system one by one. During the preview, the user canattempt the quiz by entering his/her answers to questions. The system will then immediately check theanswer and calculate. After attempting all questions, the total score will be displayed. A sample run of theprogram is shown as below (Green text refers to user input): Please choose (c)reate a question, (p)review or (e)xit >> cEnter the type of question (MC or TF) >> MCEnter the question text >> Each primitive type in Java has a correspondingclass contained in the java.lang package. These classes are called…arrow_forward
- I have 4 class. The first one is Customer Class and in this class there is a method nameProvide() that if id length less than 10 it throws exception. There is a field named name(str). The other class is Board Class. There are fields such as points(double), win (boolean). The third class is BoardProvide Class.A method pointProvide() that if points less than 100 it throws exception . And the last class is Total Class. In total class there are a field named boardProvide type of BoardProvide and a field ArrayList<Board>boards. I need a write a method that; 1) Firstly, must be provide name validity in the method if name is not valid it throws exception. if name pass then, need to create a Board. After creating Board, boardProvide must be check that whether points is acceptiple or not. Add boards list(with finally block). If it is greater than 100, set win to True. If its not, catch exception, set False and return. JAVAarrow_forwardIn Java. Define the class InvalidSideException, which inherits from the Exception class. Also define a Square class, which has one method variable -- an int describing the side length. The constructor of the Square class should take one argument, an int meant to initialize the side length; however, if the argument is not greater than 0, the constructor should throw an InvalidSideError. The Square class should also have a method getArea(), which returns the area of the square.Create a Driver class with a main method to test your classes. Your program should prompt the user to enter a value for the side length, and then create a Square object with that side length. If the side length is valid, the program should print the area of the square. Otherwise, it should catch the InvalidExceptionError, print "Side length must be greater than 0.", and terminate the program. The words for output is highlighted in grey and yellow in the blue box. Class should be named Driver, as shown in blue box…arrow_forwardA mountain climbing club maintains a record of the climbs that its members have made. Information about a climb includes the name of the mountain peak and the amount of time it took to reach the top. The information is contained in the ClimbInfo class as declared below. The ClimbingClub class maintains a list of the climbs made by members of the club. The declaration of the ClimbingClub class is shown below. You will write implementations of the addClimb method. import java.util.List; import java.util.ArrayList; class ClimbInfo { private String name; private int time; /** Creates a ClimbInfo object with name peakName and time climbTime. * * @param peakName the name of the mountain peak * @param climbTime the number of minutes taken to complete the climb */ public ClimbInfo(String peakName, int climbTime) { name = peakName; time = climbTime; } /** @return the name of the mountain peak */ public String getName() { return name; } /** @return the number of minutes…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
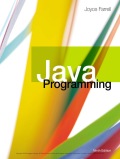
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
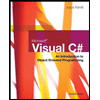
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
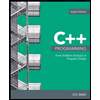
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning