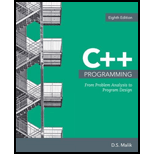
Explanation of Solution
The program has been explained in the in-lined comments:
#include <iostream>
using namespace std;
int main()
{
//delcare a list and initialize it
//with an array of 6 elements
int numList[6] = {25, 37, 62, 78, 92, 13};
//create a pointer to the list -
//the base address of the list is assigned
//to the pointer
int *listPtr = numList;
//a new pointer is declared and is assigned
//an address 2 int positions incremented i.e
//it points to the 3rd position in the array
//or numlist[2] which is the value 62
int *temp = listPtr + 2;
//declare an int variable
int num;
//assign value of the memory address pointed to
//by listPtr with the arithmetic expression on the
//RHS of the assignment operator
//RHS = *(listPtr + 1) - *listPtr =
//numList[1] - numList[0] = 37-25 = 12
//since listPtr is numList[0] so now the array elements
//are {12, 37, 62, 78, 92, 13}
*listPtr = *(listPtr + 1) - *listPtr;
//listPtr is incremented and now points to numList[1]
// i.e. 37
listPtr++;
//num is assigned the value pointed to by temp which is
//numList[2] i.e. 62
num = *temp;
//temp is incrementedand now points to numList[3]
//i...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
C++ Programming: From Problem Analysis to Program Design
- If you have the following node declaration:struct Node {int number;struct Node * next;};typedef struct Node node;node *head,*newNode;Write a C program that contains the following functions to manipulate this linked list : 3. A function deletes the element in the middle of the list (free this memory location) (if the list has 100 or 101 elements, it will delete the 50th element). The function will take a list as a parameter and return the updated list. 4. 2nd function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list. 5. 3rd function using given prototype below. This function cuts the first node of the list and adds it to the end as last node. It takes beginning address of the list as a parameter and returns the updated list.node* cutheadaddlast(node* head);arrow_forward#include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *p, *list; list = new ListNode; list->data = "New York"; p new ListNode; p->data = "Boston"; list->next = p; p->next = new ListNode; p->next->data = "Houston"; p->next->next = nullptr; // new code goes here Which of the following code correctly deletes the node with value "Boston" from the list when added at point of insertion indicated above? O list->next = p; delete p; O p = list->next; %3D list->next = p->next; delete p; p = list->next; list = p->next; delete p; O None of these O p = list->next; %3D list->next = p; %3D delete p;arrow_forwardExercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forward
- If you have the following node declaration:struct Node {int number;struct Node * next;};typedef struct Node node;node *head,*newNode;Write a C program that contains the following functions to manipulate this linked list : -----function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list.-----function using given prototype below. This function cuts the first node of the list and adds it to the end as last node. It takes beginning address of the list as a parameter and returns the updated list.node* cutheadaddlast(node* head);arrow_forwardA "generic" data structure cannot use a primitive type as its generic type. O True Falsearrow_forwardC++ Programing Create an application to keep track of a list of students and their grades in a data structures course using a linked list. You should use the C++ STL library. You need to create a class called student that contains 2 data members: name and grade and then create a linked list of objects of this class. The application should allow the user to add a student, remove a student, update the grade for a student, and search for a student. Please separate each file in your answer so it can be understood well.arrow_forward
- struct Node { int data; Node * next; }; Node • head; a. Write a function named addNode that takes in a variable of type int and inserts it at the head of the list. b. Write a function named removeNode that removes a node at the head of the list.arrow_forwardC++ Struct Pointers Help: I have a file called names.txt. Write a program that reads each line and then store the name and nickname under an individual pointer to the class Person. names.txt: Norman, Normie Justine, Jussy Richard, Dick Shelley, Shell class Person { public: string name; string nickname; Person(string name, nickname) { this->name=name; this->nickname = nickname; } }; Print out each pointer's name and nickname to verify that it has been stored.arrow_forwardPlease fill in to-do 15 #ifndef _operations_hpp_#define _operations_hpp_ #include <forward_list>#include <list>#include <map>#include <string>#include <unordered_map>#include <utility>#include <vector> #include "book.hpp" struct remove_from_bst { // Function takes a constant Book as a parameter, finds and removes from the // binary search tree the book with a matching ISBN (if any), and returns // nothing. If no Book matches the ISBN, the method does nothing. void operator()(const Book& book) { ///////////////////////// TO-DO (15) ////////////////////////////// // Write the lines of code to remove the book from "my_bst" that has an ISBN // matching "book". /////////////////////// END-TO-DO (15) //////////////////////////// }arrow_forward
- Q: Convert this to sorted array #include<iostream> #include"Student.cpp" class StudentList { private: struct ListNode { Student astudent; ListNode *next; }; ListNode *head; public: StudentList(); ~StudentList(); int IsEmpty(); void Add(Student newstudent); void Remove(); void DisplayList(); }; StudentList::StudentList() { head=NULL; }; StudentList::~StudentList() { cout <<"\nDestructing the objects..\n"; while(IsEmpty()!=0) Remove(); if(IsEmpty()==0) cout <<"All students have been deleted from a list\n"; }; int StudentList::IsEmpty() { if(head==NULL) return 0; else return 1; }; void StudentList::Add(Student newstudent) { ListNode *newPtr=new ListNode; if(newPtr==NULL) cout <<"Cannot allocate memory"; else { newPtr->astudent=newstudent; newPtr->next=head; head=newPtr; } }; void StudentList::Remove() { if(IsEmpty()==0) cout <<"List empty on remove"; else { ListNode *temp=head;…arrow_forwardcout<<"List after isolation: "; display(head);.arrow_forwardC++ Write a program that will allow a user to enter students into a database. The student information should include full name, student ID, GPA and status. The program should allow delete any student based on student ID. You may not use a vecto or an array only the list function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
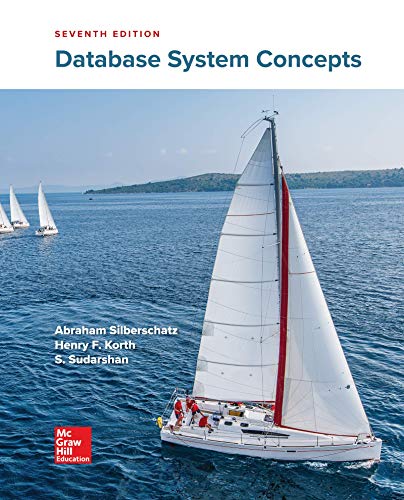
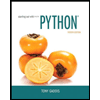
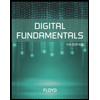
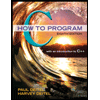
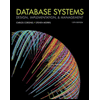
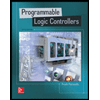