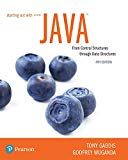
Explanation of Solution
Event handler:
- The event handler is nothing but an object that responds to the events.
- A particular method in the event handler is called if an event source is connected to the event handler. The object of the event is passed as the argument to that method.
- This process is known as event firing.
- The event handler class should implement the “EventHandler” interface.
- This interface is in the package “javafx.event”.
Step 1: Register the event handler for the button using “setOnAction ()” method.
Step 2: Inside this, set the text for the label as “Hello World”.
Statement to register an instance of the class with “myButton” control using lambda expression:
//Register the event handler
myButton.setOnAction(event ->
{
//Set the text
outputLabel.setText("Hello World");
});
Example program:
The statement to register the event handler for “myButton” is highlighted.
//Import required packages
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.scene.control.Label;
import javafx.scene.control.Button;
//Declare the main class
public class Example extends Application
{
//Declare the main method
public static void main(String[] args)
{
// Launch the application...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- In C#. Please make an interface that looks as descibes on the imagearrow_forwardWhat is a Handler used for in Android? Write a snippet of code to create a Handler and to call its post method. Note that post() must create a new of a certain type of object in its parameter. You may leave the core of the function empty.arrow_forwardCreate a Ferret class with properties name and weight passed to the constructor.arrow_forward
- Please do it in JavaScript or C# 1) Design and implement the class structure for a drawing application. A drawing is made up of a canvas that contains a collection of three different shapes: circles, lines, and boxes. Each shape can be drawn by calling a Draw() method for that shape. The canvas class should also have a draw method that draws all of the shapes it contains. You don’t have to actually draw anything on a screen; you can simulate drawing by writing a message to the console.For example, the code snippet: canvas.Draw();Drawing a circle at...Drawing a line at...etc. (For each shape on the canvas) 2)(Bonus) Consider re-writing the above answer (#1) differently where the canvas does not contain the shapes, but is able to draw any of the three shapes passed to it. Re-design canvas.Draw() if you need to. Your main() program should ask for user input on what shape to draw. Ask once, draw, then exit.could produce something like the following output to the console:arrow_forwardDescriptionIn this assignment, you are required to implement an electronic programming quiz system. User can createquestions and preview the quiz.Your TaskYou are asked to write a Java program for the programming quiz system. There are two types of questions:Multiple Choice Question and Ture/False Question. User can create questions using the system; andpreview the quiz, which display all questions in the system one by one. During the preview, the user canattempt the quiz by entering his/her answers to questions. The system will then immediately check theanswer and calculate. After attempting all questions, the total score will be displayed. A sample run of theprogram is shown as below (Green text refers to user input): Please choose (c)reate a question, (p)review or (e)xit >> cEnter the type of question (MC or TF) >> MCEnter the question text >> Each primitive type in Java has a correspondingclass contained in the java.lang package. These classes are called…arrow_forwardThe next sections will provide you with the definitions of the classes MonthCalender and DateTimerPicker respectively.arrow_forward
- You are designing a program that will keep track of the boxes in a doctor’s office. Each box will have three attributes: date, contents, and location. Write a class that will consist of box objects. Continuing with the program in problem #1, create instances of the class for these boxes: Box #23 contains medical records from 2016 and is in the storage closet. Box #21 contains lotion samples manufactured in 2018 and is in the waiting room. Box #07 contains flyers about the flu shot for 2020 and is in the receptionist’s desk. Continuing with the program in problem #2, write statements that will print out the date of box #23, the contents of box #07, and the location of box #21. Continuing with the program in problem #3, write a method that could be used to tell if the attributes of two boxes are identical. Continuing with the program in problem #4: Box #10 has identical attributes to Box #07. Create box #10. Then, write two statements that will print out: one will compare the contents of…arrow_forwardComplete MyProgram such that you can test all conditions In the Theater constructor you need to instantiate each seat object based upon the information passed to the constructor, making sure they are available. In the reassign method, make sure the from and to seat assignments are within range and return false if not. There is test cases included with this exercise, so when you submit your screenshot, it needs to show the test cases passed.arrow_forwardCreate the VisualCounter class, which supports both increment and decrement operations. Take the constructor's two arguments N and max, where N indicates the maximum operation number and max specifies the counter's maximum absolute value. Make a plot that displays the value of the counter each time its tally changes as a byproduct.arrow_forward
- Please help me solve this with java..I posted it many times and got rejected I don't ? Game class instructions:• Game class has three attributes: points (which represent the number of points awarded when winning the game), status (false if the game has not been played and true if the game has been played), and description (which is a text description of the game). Note that description attribute is a read-only variable. • In the constructor, initialize points to zero, status to false, and description to the given parameter.• isPlayed method returns true if the game has been played, and false otherwise.• getPoints is a getter method for points attribute.• play method is an abstract method.• Override toString method to return the game description.• Override equals method so that two games are the same if their descriptions are the same. :instructions class ) HangMan• HangMan is a game in which a player tries to guess a word based on a given hint. For example, if the given hint is…arrow_forwardCarly's Catering provides meals for parties and special events. In Chapter 3, you created an Event class for the company. The Event class contains two public final static fields that hold the price per guest ($35) and the cutoff value for a large event (50 guests), and three private fields that hold an event number, number of guests for the event, and the price. It also contains two public set methods and three public get methods. Now, modify the Event class to contain two overloaded constructors. One constructor accepts an event number and number of guests as parameters. Pass these values to the setEventNumber() and setGuests() methods, respectively. The setGuests() method will automatically calculate the event price. The other constructor is a default constructor that passes "A000" and 0 to the two-parameter constructor. Save the file as Event.java. b. In Chapter 3, you also created an EventDemo class to demonstrate using two Event objects. Now, modify that class to instantiate…arrow_forward2. Write an interface for Tossable. We will say that every Tossable item has a way to toss it and we want to be able to give its color. A Tossable item by default is to throw an assist toss to another player.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
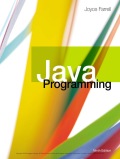
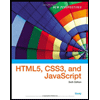