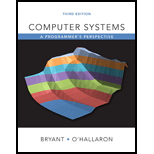
A.
Explanation of Solution
C code:
//Include Header file
#include <stdio.h>
#include "csapp.h"
//Define the maximum entry for blocked list
#define MAXIMUMSIZE 100
//Function declaration
int divideURI(char *URIName, char *hostName, char *portNumber, char *pName);
void parseBlockList(char *fName, char list[MAXIMUMSIZE][MAXLINE], int limit);
int blockedURIList(char *URIName, char list[MAXIMUMSIZE][MAXLINE]);
//Main function
int main(int argc, char **argv)
{
//Declare required variable
int i, listenfd, connfd;
int clientfd;
//Create socket
socklen_t clientlen;
//Create structure for client address
struct sockaddr_storage clientaddr;
//Create rio function
rio_t cRio, sRio;
char cbuffer[MAXLINE], sbuffer[MAXLINE];
ssize_t ssn, ccn;
char methodName[MAXLINE], URIName[MAXLINE], versionNo[MAXLINE];
char hostName[MAXLINE], portNumber[MAXLINE], pName[MAXLINE];
char block_list[MAXIMUMSIZE][MAXLINE];
int logFileDes;
char logFileBuffer[MAXLINE];
//Check command line arguments
if (argc != 2)
{
//Display message
fprintf(stderr, "usage: %s <port>\n", argv[0]);
fprintf(stderr, "use default portNumber 5000\n");
listenfd = Open_listenfd("5000");
}
else
{
listenfd = Open_listenfd(argv[1]);
}
//Open log files
logFileDes = Open("log.list", O_WRONLY | O_APPEND, 0);
//Set memory for block list
memset(block_list, '\0', MAXLINE * MAXIMUMSIZE);
//Call function for parse file
parseBlockList("block.list", block_list, MAXIMUMSIZE);
//Check condition
while (1)
{
/* wait for connection as a server */
clientlen = sizeof(struct sockaddr_storage);
//Call accept method
connfd = Accept(listenfd, (SA *) &clientaddr, &clientlen);
Rio_readinitb(&sRio, connfd);
/* Check URI Name full path */
if (!Rio_readlineb(&sRio, sbuffer, MAXLINE))
{
Close(connfd);
continue;
}
//Display the uri path
sscanf(sbuffer, "%s %s %s", methodName, URIName, versionNo);
/* Check blocked uri */
if (blockedURIList(URIName, block_list))
{
printf("%s is blocked\n", URIName);
Close(connfd);
continue;
}
//Display the visit uri
sprintf(logFileBuffer, "visit url: %s\n", URIName);
Write(logFileDes, logFileBuffer, strlen(logFileBuffer));
memset(hostName, '\0', MAXLINE);
memset(portNumber, '\0', MAXLINE);
memset(pName, '\0', MAXLINE);
//Declare variable
int res;
/* Check if the given uri is separate */
if ((res = divideURI(URIName, hostName, portNumber, pName)) == -1)
{
fprintf(stderr, "is not http protocol\n");
Close(connfd);
continue;
}
else if (res == 0)
{
fprintf(stderr, "is not a abslute request path\n");
Close(connfd);
continue;
}
//Connect server as a client
clientfd = Open_clientfd(hostName, portNumber);
Rio_readinitb(&cRio, clientfd);
//Send the first request
sprintf(sbuffer, "%s %s %s\n", methodName, pName, versionNo);
Rio_writen(clientfd, sbuffer, strlen(sbuffer));
printf("%s", sbuffer);
do
{
/* Call next http requests */
ssn = Rio_readlineb(&sRio, sbuffer, MAXLINE);
printf("%s", sbuffer);
Rio_writen(clientfd, sbuffer, ssn);
}
while(strcmp(sbuffer, "\r\n"));
//For server send reply back
while ((ccn = Rio_readlineb(&cRio, cbuffer, MAXLINE)) != 0)
Rio_writen(connfd, cbu...
B.
Explanation of Solution
C code:
#include <stdio.h>
#include "csapp.h"
//Define the maximum entry for blocked list
#define MAXIMUMSIZE 100
//Function declaration
int divideURI(char *URIName, char *hostName, char *portName, char *pathName);
void parseBlockList(char *fName, char list[MAXIMUMSIZE][MAXLINE], int limit);
int blockedURIList(char *URIName, char list[MAXIMUMSIZE][MAXLINE]);
void *proxyThreadFunction(void *vargp);
//Declare variable for blocked url list
static char bList[MAXIMUMSIZE][MAXLINE];
//Declare variable for log file fd
static int logFileDesc;
//Main function
int main(int argc, char **argv)
{
//Declare required variable
int listenfd;
//Create socket
socklen_t clientlen;
//Create structure for client address
struct sockaddr_storage clientaddr;
int *connfdp;
pthread_t tid;
//Check command line arguments
if (argc != 2)
{
//Display message
fprintf(stderr, "usage: %s <port>\n", argv[0]);
fprintf(stderr, "use default port 5000\n");
listenfd = Open_listenfd("5000");
}
else
{
listenfd = Open_listenfd(argv[1]);
}
//Open log files
logFileDesc = Open("log.list", O_WRONLY | O_APPEND, 0);
//Set memory for block list
memset(bList, '\0', MAXLINE * MAXIMUMSIZE);
//Call function for parse file
parseBlockList("block.list", bList, MAXIMUMSIZE);
//Check condition
while (1)
{
/* wait for connection as a server */
clientlen = sizeof(struct sockaddr_storage);
connfdp = Malloc(sizeof(int));
//Call accept method
*connfdp = Accept(listenfd, (SA *) &clientaddr, &clientlen);
//Generate new thread
Pthread_create(&tid, NULL, proxyThreadFunction, connfdp);
}
//Close log file descriptor
Close(logFileDesc);
}
//Function definition for proxy thread
void *proxyThreadFunction(void *vargp)
{
//Create thread id
pthread_t tid = Pthread_self();
Pthread_detach(tid);
int connfd = *(int*)vargp;
Free(vargp);
//Create rio function
rio_t cRio, sRio;
/* Declare required variable for client and server buffer */
char cBuffer[MAXLINE], sBuffer[MAXLINE];
ssize_t ssn, ccn;
/* Declare variable for method name, uri, version number, host name, port number and path name*/
char methodName[MAXLINE], URIName[MAXLINE], versionNumber[MAXLINE];
char hostName[MAXLINE], portName[MAXLINE], pathName[MAXLINE];
char logFileBuffer[MAXLINE];
int clientfd;
Rio_readinitb(&sRio, connfd);
/* Check URI Name full path */
if (!Rio_readlineb(&sRio, sBuffer, MAXLINE))
{
Close(connfd);
return NULL;
}
//Display the uri path
sscanf(sBuffer, "%s %s %s", methodName, URIName, versionNumber);
/* Check blocked uri */
if (blockedURIList(URIName, bList))
{
printf("Thread %ld: %s is blocked\n", tid, URIName);
Close(connfd);
return NULL;
}
//Print the log visit
sprintf(logFileBuffer, "Thread %ld: visit url: %s\n", tid, URIName);
Write(logFileDesc, logFileBuffer, strlen(logFileBuffer));
memset(hostName, '\0', MAXLINE);
memset(portName, '\0', MAXLINE);
memset(pathName, '\0', MAXLINE);
int res;
/* Check if the given uri is separate */
if ((res = divideURI(URIName, hostName, portName, pathName)) == -1)
{
fprintf(stderr, "tid %ld: not http protocol\n", tid);
Close(connfd);
return NULL;
}
else if (res == 0)
{
fprintf(stderr, "tid %ld: not a abslute request path\n", tid);
Close(connfd);
return NULL;
}
//Connect server as a client
clientfd = Open_clientfd(hostName, portName);
Rio_readinitb(&cRio, clientfd);
//Send the first request
sprintf(sBuffer, "%s %s %s\n", methodName, pathName, versionNumber);
Rio_writen(clientfd, sBuffer, strlen(sBuffer));
printf("tid %ld: %s", tid, sBuffer);
do
{
/* Call next http requests */
ssn = Rio_readlineb(&sRio, sBuffer, MAXLINE);
printf("Tid %ld: %s", tid, sBuffer);
Rio_writen(clientfd, sBuffer, ssn);
} while(strcmp(sBuffer, "\r\n"));
//For server send reply back
while ((ccn = Rio_readlineb(&cRio, cBuffer, MAXLINE)) != 0)
Rio_writen(connfd, cBuffer, ccn);
�...

Trending nowThis is a popular solution!

Chapter 12 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- USING JAVA: Implement a simple e-mail messaging system. A message has a recipient, a sender, and a message text. A mailbox can store messages. Supply a number of mailboxes for different users and a user interface for the user to login, send messages to other users, read their own messages, and log out.arrow_forwardWrite a Java Servlets program for calculating the total number of hits on a web page designed by you. The web page should contain at least one title, one label and one output tag which displays the result of the calculation i.e. the number of hits on that page. The number of hits should be counted only for the one lifecycle of the servlet. Note that the program should have the comments embedded in it to explicitly explain why that code is being used.arrow_forwardThe execution of the session design pattern in an online application could look something like this: The Session class in this example is built as a singleton and has methods for logging in and out, verifying the state of verification, and obtaining the user.The class has a static way for locating the sole instance as well as a private function Object() { [native code] } that makes sure no more than one instance of the class can be made.In this manner, the user's login state can be checked, their user object can be retrieved, and their session can be maintained throughout the application by accessing the session object from anywhere in the application.arrow_forward
- Write a JavaFX program that let the user to select a signal major or multiple majors from a list of majors and display the selected majors in the text area as shown below. The program should let the user to choose a Selection Mode from: SINGLE or MULTIPLE. When the program starts no items should be displayed in the text area. The list of Majors are IT Majors given below: Cyber Security Choose Selection Mode: SINGLE Selected items are: Cyber Forensics No items selected Cyber Security Networks Security Cyber Forensics Computer Networks Data Management Networks Security Computer Networks Data Analytics Software Development Data Management Web Design Data Analytics Applications Development Software Develoomentarrow_forwardDevelop a server program in Java that waits for clients to connect. The server responds to the client to choose one of the following options available withserver and then deliver the requested object to client:SumMultiplyDivisionBye Server Program is first started. Then the Client program is started. Ask three questions from Server Get the answer from client Finally send the resutls.Execute the following steps:Step 1: Run the Java program of ServerStep 2: Run the Java program of Client and Sample output of Client Window:Sample output 1(Client):Connected to serverMessage from Server: Select the option:SumMultiplyDivisionByeEnter the message to the server: 1Reply from Server: What is the sum of 2 + 3 ?Enter the message to the server: 4Reply from Server: Your Answer not correctReply from Server: What is the sum of 4 + 3 ?Enter the message to the server: 7Reply from Server: Your Answer correct, CongratsReply from Server: What is the sum of 10 + 11 ?Enter the message to the server:…arrow_forwardCreate a java program that establishes a connection between a client and a server socket. The client should send a request to the server and the server should accept the request. The client should then send a number to the server; the server will receive the number and square it. For example, if the client sends the number 8, the server should respond with 64; if the client sends 10, server should respond with 100. The client should then display the squared number. You are required to use the InetAddress class to get the localhost address and use 2415 as the port number for the server socket. Make sure that you close the socket connectionarrow_forward
- Using java swing coding, you have to 1. Display the list (table) in descending order of points. 2. Display the list (table) in ascending order. 3. Add a button which every time it is pressed it generates one random page. 4. Add a button which every time it is pressed it generates the previous page. 5. Add a button and a textbox which can be used to search for all details.arrow_forwardIn javascript: Page #1: Greets User 1 (The Word Chooser) and asks for their Name, a word, and the number of wrong guesses they would like to give User 2(The Guesser).User 1 then clicks the "Go" button, which leads to page 2. Page #2: Greets User 2 by telling them the word choosers name and how many guesses they have been given for the word, they initially see a "-" for each unguessed letter, and a counter with remaining bad guesses. They must also be shown previously guessed letters. There is a place for them to enter their name and to guess letters. After all guesses are done, win or lose, the page goes to: Page #3: Congratulates the user, or informs them of their failure to guess the word. This page must show: User 1 Name User 2 Name Word they were trying to guessarrow_forwardWrite a GUI Java program that creates the following interface:• In the Text Filed enter a floating number and select the conversion metrics from the two drop-down lists. • When the Button “Calculate” is clicked, convert the input number based on the selected metrics and display the result in the Text Area.• When “Clear” Button is clicked, the Text Filed and the Text Area should be blank.arrow_forward
- Help with JavaFX barchart: I have a program that allows a user to deposit and withdraw money while updating their balance based on the month the transaction occured, but I am having trouble with the programs class and am trying to make a bar chart that will have the total balance of that month, so as an example in May the bar would show $70, while the bar for June would show $100, even if the deposits were $20 and $50, then $30, $40 and $30.arrow_forwardYou are supposed to make a system for a bakery. The bakery owner plans to reduce the staff and implement a system that can display the items available in the bakery with their price for the ease of customer. Different items are available in the bakery and are categorize under different options such as: Fried Items: Chicken Samosa Vegetable Roll Baked Items: Chicken Patty Potato Patty Munch Stuff: Chips Biscuits Beverages: Cold drink Juice Water The customer can view items from different list by selecting the number associated with each list. If the customer wishes to exit the menu, he/she will be redirected to the beginning of the system where about the bakery and menu option is available.arrow_forwardScenario: You are tasked with the development of an E-Scooter ride-sharesystem. It allows registered commuters to approach an idle E-Scooter andreserve it, following which they use the E-Scooter to commute a certaindistance (that is not known prior to use). Finally, after the commuter reachestheir destination, they end the ride, which prompts an automaticcomputation of the ride fees. which is automatically debited using thecommuters registered payment details. Task(s):1. Create a CPN model based on the AOM Goal and Behavioural InterfaceModels using the mapping heuristics discussed in the lecture.2. The initial tokens (initial state) of your system should reflect at least 2scooters and 2 commuters. More generally → Please ensure a level ofcomplexity similar to the CPN model example given in the lecture.3. You can use the CPN model example used in the lecture and modify itto suit your needs.4. You are welcome to use hierarchical CPNs to follow the Goal hierchy ifyou like, but it is not…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
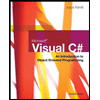