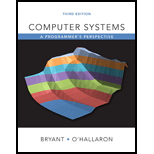
Concept explainers
Explanation of Solution
Implementation of a concurrent prethreaded version of the TINY web server:
Modified code for “sbuf.h” file:
The modified code for “sbuf.h” from section 12.5.4 in book is given below:
#ifndef SBUF_HEADER
#define SBUF_HEADER
#include "csapp.h"
typedef struct
{
int *buf; /* Buffer array */
int n; /* Maximum number of slots */
int front; /* buf[(front+1)%n] is first item */
int rear; /* buf[rear%n] is last item */
sem_t mutex; /* Protects accesses to buf */
sem_t slots; /* Counts available slots */
sem_t items; /* Counts available items */
} sbuf_t;
//Function declaration
void sbuf_init(sbuf_t *sp, int n);
void sbuf_deinit(sbuf_t *sp);
void sbuf_insert(sbuf_t *sp, int item);
int sbuf_remove(sbuf_t *sp);
//Function declaration for sbuf_empty
int sbuf_empty(sbuf_t *sp);
//Function declaration for sbuf_full
int sbuf_full(sbuf_t *sp);
#endif
Modified code for “sbuf.c” file:
The modified code for “sbuf.c” from section 12.5.4 in book is given below:
#include "csapp.h"
#include "sbuf.h"
/* Create an empty, bounded, shared FIFO buffer with n slots */
void sbuf_init(sbuf_t *sp, int n)
{
sp->buf = Calloc(n, sizeof(int));
sp->n = n; /* Buffer holds max of n items */
sp->front = sp->rear = 0; /* Empty buffer if front == rear */
Sem_init(&sp->mutex, 0, 1); /* Binary semaphore for locking */
Sem_init(&sp->slots, 0, n); /* Initially, buf has n empty slots */
Sem_init(&sp->items, 0, 0); /* Initially, buf has zero data items */
}
/* Clean up buffer sp */
void sbuf_deinit(sbuf_t *sp)
{
Free(sp->buf);
}
/* Insert item onto the rear of shared buffer sp */
void sbuf_insert(sbuf_t *sp, int item)
{
P(&sp->slots); /* Wait for available slot */
P(&sp->mutex); /* Lock the buffer */
sp->buf[(++sp->rear)%(sp->n)] = item; /* Insert the item */
V(&sp->mutex); /* Unlock the buffer */
V(&sp->items); /* Announce available item */
}
/* Remove and return the first item from buffer sp */
int sbuf_remove(sbuf_t *sp)
{
int item;
P(&sp->items); /* Wait for available item */
P(&sp->mutex); /* Lock the buffer */
item = sp->buf[(++sp->front)%(sp->n)]; /* Remove the item */
V(&sp->mutex); /* Unlock the buffer */
V(&sp->slots); /* Announce available slot */
return item;
}
//Function definition for empty buffer
int sbuf_empty(sbuf_t *sp)
{
//Declare variable
int ne;
//For lock the buffer
P(&sp->mutex);
ne = sp->front == sp->rear;
//For lock the buffer
V(&sp->mutex);
return ne;
}
//Function definition for full buffer
int sbuf_full(sbuf_t *sp)
{
//Declare variable
int fn;
//For lock the buffer
P(&sp->mutex);
fn = (sp->rear - sp->front) == sp->n;
//For lock the buffer
V(&sp->mutex);
return fn;
}
For code “tiny.c” and “tiny.h”:
Same code as section 11.6 in book.
sample.html:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Home</title>
</head>
<body>
Tiny server Example
</body>
</html>
main.c:
#include <stdio.h>
#include "csapp.h"
#include "tiny.h"
#include "sbuf...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- Write a program using pthreads, which calculates the sum of elements in a hard-coded integer array in parallel using 4 threads. The program must divide the work between 4 threads which run simultaneously. For simplicity, you can assume that the size of the array is 100. Note that the integer array must be declared as a global data structure. Initially code your solution so that the sum of elements is maintained in a global shared variable. Each thread modifies the same shared variable as it sums up elements from the array. Use a suitable synchronization primitive (mutex) to ensure safe access to the global variable. (A sample code of Mutex is attached for your reference)...also put screenshot of output.arrow_forwardWrite a MultiThreaded ServerSocket Program in C# that can handle multiple clients at the same time. a C# Server Socket use TcpListener Class and listen to PORT 9393. When the C# Server Socket gets a request from Client side, the Server passes the instance of the client request to a separate class handleClient. For each Client request, there is a new thread instant is created in C# Server for separate communication with Client.arrow_forwardHello, the code task below has to be written in python 3 and the HTTP must show the html file from the command line. Please help. Currently, the web server handles only one HTTP request at a time. Implement a multithreaded server that is capable of serving multiple requests simultaneously. Using threading, first create a main thread in which your modified server listens for clients at a fixed port. When it receives a TCP connection request from a client, it will set up the TCP connection through another port and services the client request in a separate thread. There will be a separate TCP connection in a separate thread for each request/response pair.arrow_forward
- Write a class that extends thread, called clientEng, to implement the following part of client protocol 1- The client thread sends an integer number, let us assume it is N, to the server. 2- The server iterates from 1 to 10 to send 10 values that results from multiplying N by the iteration index. 2- The client gets into a loop that iterates 10 times to receive the 10 values. 3- In each iteration the client prints on the screen a message as "N * M =value ", where M is the number of iteration from 0 to 10 and value is the received value. 4- after the last iteration the client should sends a 'done message from the server. 5- The client receives "OK message from the server and closes the socket. Do not write any other code, other than the thread class, but Add a line that allows for creating the running the code of clientEng.arrow_forwardWrite a program that launches 26 threads. Each thread represents an English alphabet, say a, b, c, etc. Each thread should concatenate the string "+ ch " to a string variable that is initially defined as the string " " (i.e. empty string). "ch" represents the character of the current thread being executed (e.g. a, b, c,..) . Print your string variable after all threads have been executed. The targeted correct output may look like "a+b+c+d+e+…..". Run the program with and without synchronization to see its effect. Comment on the non-synchronized output.arrow_forwardWrite complete Java code to define a thread for printing all the Odd Nos from 1 to 5000 which are divisible by 9. Thread must sleep for 5 seconds. Implement the threading using the Runnable java provided Interface. Finally, create three different threads with names ThreadOne, ThreadTwo and ThreadThree in main(). Execute the code in the file where threading is implemented. Apply the Java multithreading exception handling mechanism properlyarrow_forward
- Write a program that simulates a paging system using the aging algorithm. The number of page frames is a parameter. The sequence of page references should be read from a file. For a given input file, plot the number of page faults per 1000 memory references as a function of the number of page frames available. Code Solution: https://github.com/preethikandhalu/C/blob/master/aging.c Task to be done based on the above program: Describe what the code does, explaining how it accomplishes its task of implementing the aging page replacement algorithm. You must create an input file for simulating a sequence of page references. Run the program. (Fix any bugs that it may contain, first). Vary the number of page frames, keeping the sequence of page references constant. What can you infer from the results? Plot the output in an Excel graph. Explain your findings. Adjust your inputs to get meaningful outputs.arrow_forwardPython using Mpy4py. 1. Load a txt file 2. count the total number of all repeated words and export it to a txt file 3. report the word count time using multiple threads from 1 to 8. Please make it simple without importing complex modules since it doesn't work on my mac. like: import multipyparallel as ipp please write comments on the description on each lines of code.arrow_forwardImplement the producer consumer problem (also known as bounded buffer problem) in java. create two threads i.e. the producer thread and the consumer thread. Create a shared buffer object using a LinkedList or Queue. The producer adds data to the buffer and the consumer removes data from the buffer. The producer cannot add data if the buffer is full and the consumer cannot remove data from the buffer if it is empty. Make sure there are no race conditions and deadlocks.arrow_forward
- 10. Write a multi-threading JAVA program having four threads:- The “Generator” thread: it takes as argument (in the constructor) aninteger N and generates an array of N integers- The “Even” thread: it takes as argument (in the constructor) an array ofintegers and counts the number of even numbers in the array- The “Odd” thread: it takes as argument (in the constructor) an array ofintegers and count the number of odd numbers in the array- The main thread:o it asks the user to enter an integer No Then, it runs the “Generator” thread to create an array of integersand waits the end of “Generator”o Then, it run “Even” and “Odd” to count the number of even andodd numbers in the generated arrayo Finally, it prints if there is more even or odd numbersNote: the main thread should wait the end of “Even” and “Odd”threads before printing the final result.arrow_forwardWrite a Java program using threads to compute the first 15 natural numbers, and to compute the first 50 Fibonacci numbers. Set the priority of thread that computes Fibonacci number to 9 and the other to 5. After calculating 30 Fibonacci numbers, make that thread to sleep and take up natural number computation. After computing the 15 natural numbers continue the Fibonacci number computing.arrow_forwardWrite a program that creates a certain number of sellerthreads thatattempt to sell all the available tickets. There is a global variablenumTickets which tracks the number of tickets remaining to sell.We will create many threads that all will attempt to sell tickets untilthey are all gone. Each thread will exit after all the tickets havebeen sold.E.g. if we have 4 tickets and 2 sellerthreads then output should be likeSeller #1 sold one (3 left)Seller #0 sold one (2 left)Seller #1 sold one (1 left)Seller #0 sold one (0 left)Seller #1 noticed all tickets sold! (I sold 2myself)Seller #0 noticed all tickets sold! (I sold 2myself)Done use c++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
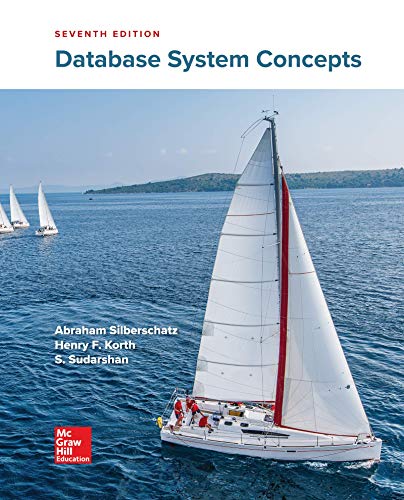
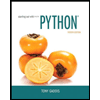
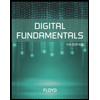
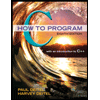
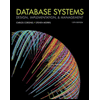
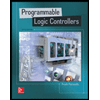