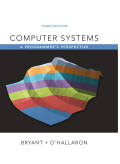
Readers-Writers problem:
- The readers-writers interactions would happen frequently in real systems.
- It has some variations; each is centered on priority of writers and readers.
- The details for “first readers-writers problem” is displayed below:
- This problem favors readers.
- It needs that no reader must be kept waiting lest a writer has already been granted approval to use object.
- There should be no reader waiting due to waiting of writer.
- The details for “second readers-writers problem” is displayed below:
- This problem favors writers.
- It requires that after a writer is set to write, it performs write as fast as possible.
- A reader arriving after writer should wait, even if writer is also waiting.
- The “w” semaphore controls access to critical sections that access shared object.
- The “mutex” semaphore would protect admittance to shared variable “readcnt”.
- It counts number of readers currently in critical section.
- A writer locks “w” mutex each time it would enter critical section and unlocks it each time it leaves.
- This guarantees that there exists at most one writer in critical section at any time point.
- The first reader who enter critical section locks “w” and last reader to leave critical section unlocks it.
- The “w” mutex is ignored by readers who enter and leave while other readers are present.
- A correct solution to either of readers-writers problem could result in starvation.
- A thread is been blocked indefinitely and is failed from making progress.

Explanation of Solution
C code for readers-writers problem:
//Include libraries
#include <stdio.h>
#include "csapp.h"
//Define constants
#define WrteLmt 100000
#define Pple 20
#define N 5
//Declare variable
static int readtms;
//Declare variable
static int writetms;
//Declare semaphore variable
sem_t mtx;
//Declare semaphore variable
sem_t rdrcnt;
//Declare reader method
void *reader(void *vargp)
{
//Loop
while (1)
{
//P operation
P(&rdrcnt);
//P operation
P(&mtx);
//Increment variable
readtms++;
//V operation
V(&mtx);
//V operation
V(&rdrcnt);
}
}
//Declare writer method
void *writer(void *vargp)
{
//Loop
while (1)
{
//P operation
P(&mtx);
//Increment value
writetms++;
//If condition satisfies
if (writetms == WrteLmt)
{
//Display
printf("read/write: %d/%d\n", readtms, writetms);
//Exit
exit(0);
}
//V operation
V(&mtx);
}
}
//Declare init method
void init(void)
{
//Declare variables
readtms = 0;
//Declare variables
writetms = 0;
//Call method
Sem_init(&mtx, 0, 1);
//Call method
Sem_init(&rdrcnt, 0, N);
}
//Define main
int main(int argc, char* argv[])
{
//Declare variable
int li;
//Declare thread variable
pthread_t lTd;
//Call method
init();
//Loop
for (li = 0; li < Pple; li++)
{
//If condition satisfies
if (li%2 == 0)
//Call method
Pthread_create(&lTd, NULL, reader, NULL);
//If condition does not satisfies
else
//Call method
Pthread_create(&lTd, NULL, writer, NULL);
}
//Call method
Pthread_exit(NULL);
//Exit
exit(0);
}
Explanation:
- The reader method decrements the reader count and semaphore initially.
- The reading operation is performed after that.
- The reader count and semaphore values are incremented after the operation.
- The writer method decrements the semaphore variable initially.
- The write operation is then performed.
- If count reaches limit of writers, then display count.
- The semaphore values are incremented after the operation.
read/write: 142746/100000
Want to see more full solutions like this?
Chapter 12 Solutions
EBK COMPUTER SYSTEMS
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
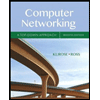
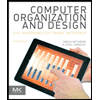
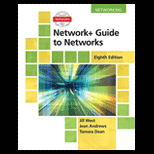
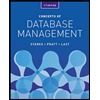
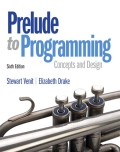
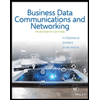