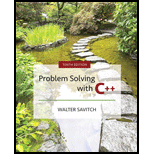
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 11.1, Problem 7STE
The Pitfall section entitled “Leading Zeros in Number Constants” suggests that you write a short
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Explian thiS C program
#include<stdio.h>
int countSetBits(int n) { int count = 0; while (n) { count += n & 1; n >>= 1; } return count;}
int main() { int num; printf("Enter a number: "); scanf("%d", &num); printf("Output: %d units\n", countSetBits(num)); return 0;}
Please provide the Mathematica code
Explian this C program code.
#include <stdio.h>
void binary(unsigned int n) {
if (n /2!=0) {
binary(n /2);
}
printf("%d", n %2);
}
int main() {
unsignedint number =33777;
unsignedchar character ='X';
printf("Number: %u\n", number);
printf("Binary: ");
binary(number);
printf("\nDecimal: %u\nHexadecimal: 0x%X\n\n", number, number);
printf("Character: %c\n", character);
printf("ASCII Binary: ");
binary(character);
printf("\nASCII Decimal: %u\nASCII Hexadecimal: 0x%X\n", character, character);
return0;
}
Chapter 11 Solutions
Problem Solving with C++ (10th Edition)
Ch. 11.1 - Write a function definition for a function called...Ch. 11.1 - What is the difference between a friend function...Ch. 11.1 - Suppose you wish to add a friend function to the...Ch. 11.1 - Prob. 4STECh. 11.1 - Notice the member function output in the class...Ch. 11.1 - Notice the definition of the member function input...Ch. 11.1 - The Pitfall section entitled Leading Zeros in...Ch. 11.1 - Give the complete definition of the member...Ch. 11.1 - Why would it be incorrect to add the modifier...Ch. 11.1 - What are the differences and the similarities...
Ch. 11.1 - Given the following definitions: const int x = 17;...Ch. 11.2 - What is the difference between a (binary) operator...Ch. 11.2 - Prob. 13STECh. 11.2 - Suppose you wish to overload the operator = so...Ch. 11.2 - Prob. 15STECh. 11.2 - Give the definition for the constructor discussed...Ch. 11.2 - Here is a definition of a class called Pairs....Ch. 11.2 - Following is the definition for a class called...Ch. 11.3 - Give a type definition for a structure called...Ch. 11.3 - Write a program that reads in five amounts of...Ch. 11.3 - Change the class TemperatureList given in Display...Ch. 11.3 - Prob. 22STECh. 11.3 - If a class is named MyClass and it has a...Ch. 11.4 - Prob. 24STECh. 11.4 - The following is the first line of the copy...Ch. 11.4 - Answer these questions about destructors. a. What...Ch. 11.4 - a. Explain carefully why no overloaded assignment...Ch. 11 - Modify the definition of the class Money shown in...Ch. 11 - Self-Test Exercise 17 asked you to overload the...Ch. 11 - Self-Test Exercise 18 asked you to overload the...Ch. 11 - Prob. 1PPCh. 11 - Define a class for rational numbers. A rational...Ch. 11 - Define a class for complex numbers. A complex...Ch. 11 - Enhance the definition of the class StringVar...Ch. 11 - Define a class called List that can hold a list of...Ch. 11 - Define a class called StringSet that will be used...Ch. 11 - This programming project requires you to complete...Ch. 11 - Redo Programming Project 6 from Chapter 9 (or do...Ch. 11 - Solution to Programming Project 11.12 To combat...Ch. 11 - Repeat Programming Project 11 from Chapter 10 but...Ch. 11 - Do Programming Project 19 from Chapter 8 except...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Tuition Increase At one college, the tuition for a full-time student is 8,000 per semester. It has been announc...
Starting Out with Python (4th Edition)
What is an object? What is a control?
Starting Out With Visual Basic (8th Edition)
Write a complete Java program that reads a line of keyboard input containing two values of type int separated b...
Java: An Introduction to Problem Solving and Programming (8th Edition)
What is the disadvantage of having too many features in a language?
Concepts Of Programming Languages
In Exercises 55 through 60, find the value of the given function where a and b are numeric variables of type Do...
Introduction To Programming Using Visual Basic (11th Edition)
Write Python statements that print the following: a. The words Computer Science Rocks, followed by an exclamati...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Design a dynamic programming algorithm for the Longest Alternating Subsequence problem described below: Input: A sequence of n integers Output: The length of the longest subsequence where the numbers alternate between being larger and smaller than their predecessor The algorithm must take O(n²) time. You must also write and explain the recurrence. Example 1: Input: [3, 5, 4, 1, 3, 6, 5, 7, 3, 4] Output: 8 ([3, 5, 4, 6, 5, 7, 3, 4]) Example 2: Input: [4,7,2,5,8, 3, 8, 0, 4, 7, 8] Output: 8 ([4, 7, 2, 5, 3, 8, 0,4]) (Take your time with this for the subproblem for this one)arrow_forwardDesign a dynamic programming algorithm for the Coin-change problem described below: Input: An amount of money C and a set of n possible coin values with an unlimited supply of each kind of coin. Output: The smallest number of coins that add up to C exactly, or output that no such set exists. The algorithm must take O(n C) time. You must also write and explain the recurrence. Example 1: Input: C24, Coin values = = [1, 5, 10, 25, 50] Output: 6 (since 24 = 10+ 10+1+1 +1 + 1) Example 2: Input: C = 86, Coin values = [1, 5, 6, 23, 35, 46, 50] Output: 2 (since 86 = 46+35+5)arrow_forwardDesign a dynamic programming algorithm for the Longest Common Subsequence problem de- scribed below Input: Two strings x = x1x2 xm and y = Y1Y2... Yn Output: The length of the longest subsequence that is common to both x and y. . The algorithm must take O(m n) time. You must also write and explain the recurrence. (I want the largest k such that there are 1 ≤ i₁ < ... < ik ≤ m and 1 ≤ j₁ < ... < jk ≤ n such that Xi₁ Xi2 Xik = Yj1Yj2 ··· Yjk) Example 1: Input: x = 'abcdefghijklmnopqrst' and y = 'ygrhnodsh ftw' Output: 6 ('ghnost' is the longest common subsequence to both strings) Example 2: Input: x = 'ahshku' and y = ‘asu' Output: 3 ('asu' is the longest common subsequence to both strings)arrow_forward
- Design a dynamic programming algorithm for the problem described below Input: A list of numbers A = = [a1,..., an]. Output: A contiguous subsequence of numbers with the maximum sum. The algorithm must take O(n) time. You must also write and explain the recurrence. (I am looking for an i ≥ 1 and k ≥ 0 such that a + ai+1 + ···ai+k has the largest possible sum among all possible values for i and k.) Example 1: Input: A[5, 15, -30, 10, -5, 40, 10]. Output: [10, 5, 40, 10] Example 2: Input: A = [7, 5, 7, 4, -20, 6, 9, 3, -4, -8, 4] Output: [6,9,3]arrow_forwardDesign a dynamic programming algorithm for the Longest Increasing Subsequence problem described below: Input: A sequence of n integers Output: The length of the longest increasing subsequence among these integers. The algorithm must take O(n²) time. You must also write and explain the recurrence. Example 1: Input: [5, 3, 6, 8, 4, 6, 2, 7, 9, 5] Output: 5 ([3, 4, 6, 7, 9]) Example 2: Input: [12, 42, 66, 73, 234, 7, 543, 16] Output: 6 ([42, 66, 73, 234, 543])arrow_forwardDesign a dynamic programming algorithm for the Subset Sum problem described below: Input: A set of n integers A and an integer s Output: A subset of A whose numbers add up to s, or that no such set exists. The algorithm must take O(n·s) time. You must also write and explain the recurrence. Example 1: Input: A = {4, 7, 5, 2, 3}, s = 12 Output: {7,2,3} Example 2: Input: A{4, 7, 5,3}, s = 6 Output: 'no such subset'arrow_forward
- TECNOLOGIE DEL WEB 2023/2023 (VER 1.1) Prof. Alfonso Pierantonio 1. Project Requirements The project consists in designing and implementing a Web application according to the methodology and the technologies illustrated and developed during the course. This document describe cross-cutting requirements the application must satisfy. The application must be realized with a combination of the following technologies: PHP MySQL HTML/CSS JavaScript, jQuery, etc templating The requirements are 2. Project size The application must have at least 18 SQL tables The number of SQL tables refers to the overall number of tables (including relation normalizations). 3. Methodology The application must be realized by adopting separation of logics, session management, and generic user management (authentication/permissions). Missing one of the above might correspond to a non sufficient score for the project. More in details: 3.1 Separation of Logics The separation of logics has to be realizse by using…arrow_forwardWrite a C program to calculate the function sin(x) or cos(x) using a Taylor series expansion around the point 0. In other words, you will program the sine or cosine function yourself, without using any existing solution. You can enter the angles in degrees or radians. The program must work for any input, e.g. -4500° or +8649°. The function will have two arguments: float sinus(float radians, float epsilon); For your own implementation, use one of the following relations (you only need to program either sine or cosine, you don't need both): Tip 1: Of course, you cannot calculate the sum of an infinite series indefinitely. You can see (if not, look in the program) that the terms keep getting smaller, so there will definitely be a situation where adding another term will not change the result in any way (see problem 1.3 – machine epsilon). However, you can end the calculation even earlier – when the result changes by less than epsilon (a pre-specified, sufficiently small number, e.g.…arrow_forwardWrite a C program that counts the number of ones (set bits) in the binary representation of a given number. Example:Input: 13 (binary 1101)Output: 3 unitsarrow_forward
- I need help to resolve or draw the diagrams. thank youarrow_forwardYou were requested to design IP addresses for the following network using the addressblock 166.118.10.0/8, connected to Internet with interface 168.118.40.17 served by the serviceprovider with router 168.118.40.1/20.a) Specify an address and net mask for each network and router interface in the table provided. b) Give the routing table at Router 1.c) How will Router 1 route the packets with destinationi) 168.118.10.5ii) 168.118.10.103iii) 168.119.10.31iii) 168.118.10.153arrow_forwardI would like to get help to draw an object relationship diagram for a typical library system.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
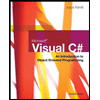
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
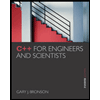
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
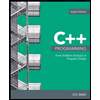
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
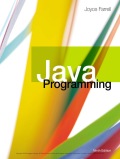
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
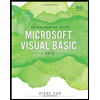
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Introduction to Operators in C; Author: Neso Academy;https://www.youtube.com/watch?v=50Pb27JoUrw;License: Standard YouTube License, CC-BY