What are Operators?
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
A simple example of an operator used for multiplying two numbers, say X and Y that take on numerical values is given below. The operation is performed and the result of the operation is stored onto variable Z.
Z = X * Y
In the above expression, the operator is the asterisk symbol, which instructs the compiler to multiply the numbers and get the results of the calculations.
Operator Precedence
It is important to note that in a statement, there is an order of execution of instructions that employ operators. Operator precedence ensures that a mathematical expression is evaluated correctly. It is done according to the BODMAS (Brackets, Division, Multiplication, Addition and Subtraction) rule. Operator precedence usually follows the order below which is listed from highest precedence to lowest precedence.
- Postfix operators (Like parentheses)
- Unary operators (Like ++ operator and size of)
- Multiplicative notations ( *, %, /)
- Additive notations (+, -)
- Shift Operators (<<, >>)
- Relational Operators (<, >, <==, >==)
- Bitwise operators (&, |)
- Logical operators (&&, ||)
- Conditional operator (?:)
- Assignment Operator (=, and its other forms)
Classification of Operators
The most basic types of operators used in any programming language are:
- Arithmetic Operators
- Relation Operators
- Logical Operators
- Assignment Operator
- Bitwise Operators
- Condition operator (ternary operator)
- Increment/decrement operators
- Special operators
Arithmetic Operators
Arithmetic operators are those that symbolize arithmetic calculations. They are of two major types:
- Unary
- Binary
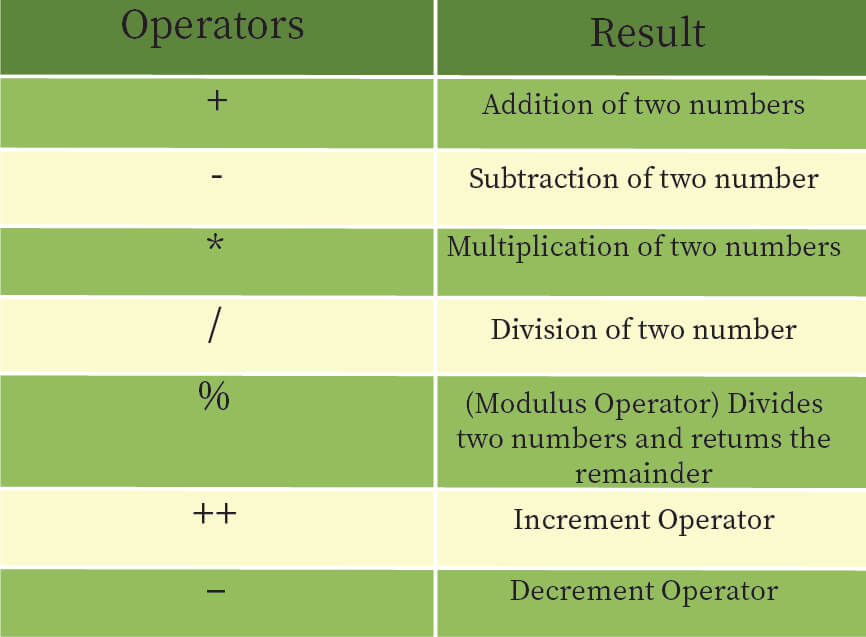
Unary Operators
They represent increment/decrement operations. These operators take as input only one operand. The increment operator is represented as ++, while the decrement operator is represented as --. Based on the position of these operators they are classified as follows.
- Pre-increment operator: When the ++ operator is placed before a variable (like ++var_name), it first increments the variable value by 1 before displaying the value.
- Post-increment operator: When the ++ operator is placed after the variable (like var_name++), it first returns the initial value stored in the variable before incrementing the value by 1.
- Pre-decrement and post-decrement operators work in the same way, but they decrement or subtract the variable value by 1.
Apart from this, the unary family can also include unary plus and minus symbols. If a value held by a variable is arithmetic, the unary plus simply gives back the same value, which the unary minus negates the value of the variable.
Binary Operators
In an equation or expression, a binary operation represents the action to be performed on two values that are present on either side of the operator. Some of the commonly used binary operators are mentioned below.
- Addition: The symbol '+' represents the addition of two operands. For instance, a+b.
- Subtraction: The symbol '-' informs the compiler to perform subtraction of the right operand from the left operand. For instance, a-b subtracts 'b' (the second operand) from 'a' (the left operand).
- Multiplication: The product of the left hand operand and the right operand is the result of using the symbol '*'. For example, a*b results in the multiplication of the value in 'a' (the left operand) and the value in 'b' (the second operand).
- Division: The symbol '/' shows the division of the left operand (the dividend) by the right operand (the divisor). The result is the quotient. For instance, a/b basically results in the division of 'a' (the left hand operand) by 'b' (the operand on the right).
- Modulus: ‘%’ returns the remainder of the dividend when the first operand is divided by the second. For eg, a%b.
- Exponentiation operator: There is no such operator to calculate exponents in C (a power function is used instead). The set of Java operators also do not contain an exponentiation symbol. However, in Python, ** is used to calculate exponents, where the first operand is raised to the power of the second operand's value. For instance, 2**3 will return the value 8.
Relational Operators
When comparing the values of different operands, a relationship can be established between them. For instance, when comparing the numbers 1 and 15, it is easy to differentiate their values by claiming that 1 is lesser than 15, and 15 is greater than 1. They are also referred to as comparison operators.
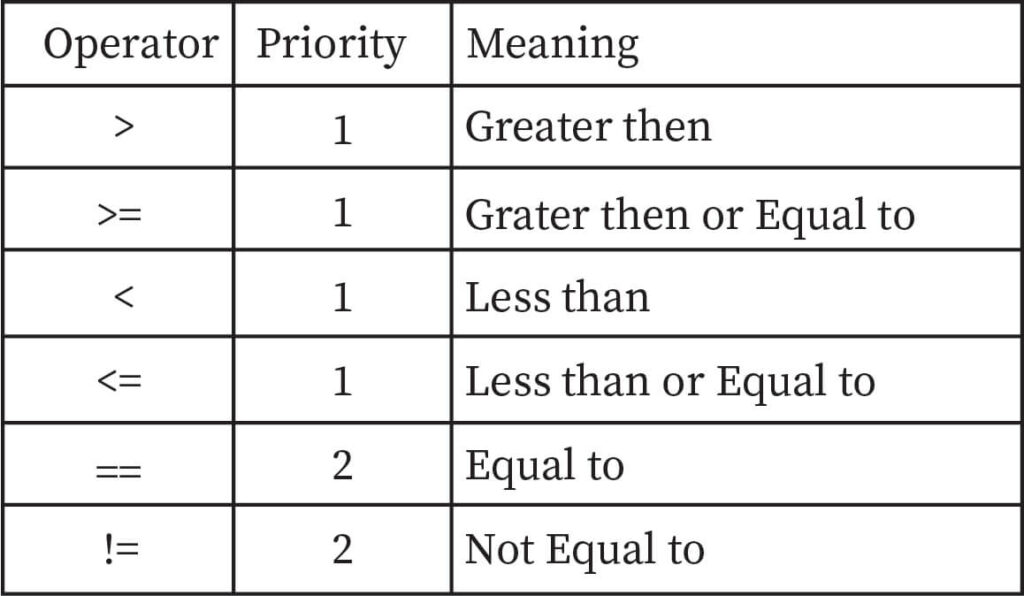
Some of the relational operators are:
- Equal to: It is represented as two consecutive equal symbols (==) used to compare if two operands are equal or not. If they are equal, it returns 'true'. If not, it returns 'false'. For instance, 6 == 6 will return the value 'true'.
- Not equal to: The Boolean complement of the equal to symbol, this operator is depicted as an exclamation mark followed by a single equal sign (!=), it is used to check if two variables are equal or not. It will similarly return true or false values accordingly. For example, 5 != 7 will result in 'true'.
- Greater than or equal to: Denoted as ‘>=’, it is used to verify if the first value is greater than or equal to the second value or not. For example, 6 >= 5 will returns the value 'true'.
- Less than or equal to: It is written as ‘<=’ and is utilized while checking whether the first value is lesser than or equal to the second value. For instance, 5 <= 6 will return the value 'true'. Both 6>=5 and 5<=6 are Boolean expressions that are equivalent.
- Greater than: Denoted as ‘>’, this comparison operator is used to check if the first value is greater than the second value or not. For example, 6 > 5 will return True.
- Less than: Represented as ‘<‘, this comparison operator is employed to check if the first value is lesser than the second value or not. For instance, 6 < 8 will present the value true.
Logical operators
This class of operators is used to combine two or more conditions, constraints, or expressions such that a new expression is produced. The new expression is solely dependent on the meaning behind the operator and the component expressions. These operators help derive results like those depicted in logical truth tables. The well-known logical operators are as follows:
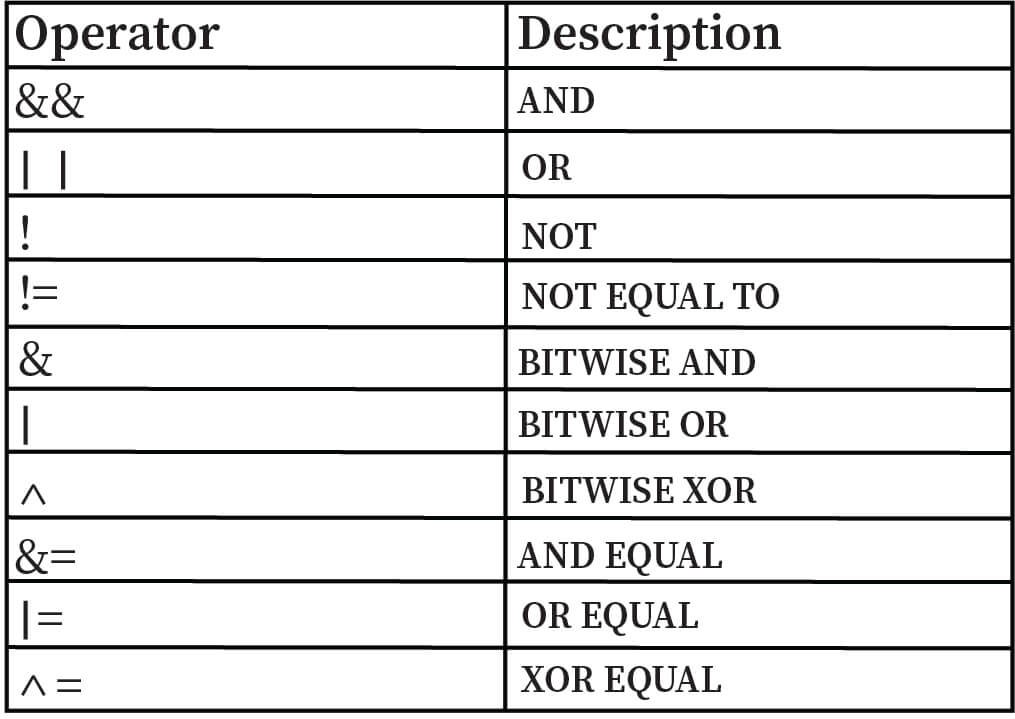
- Logical NOT: The exclamation mark (!) returns an inverted value of the input. For instance, if the input is the number 1, then the result of the NOT gate is 0 as vice versa. This can be used with Boolean values (true or false) as well.
- Logical OR: The symbol ‘||’ returns 'true' if either of the two inputs is true. Otherwise, it returns false. For instance, a || y returns true if either one or both of the variables are true (i.e. non-zero). Otherwise, it returns false.
- Logical AND: The symbol ‘&&’ returns true only if both the conditions are satisfied. In other cases, 'false' is returned. For instance, x && y returns true only when both x and y are true (i.e. non-zero).
Ternary operator
The conditional operator is a ternary operator as it performs executions on three operands. The first operand highlights a condition. The second operand is what executes if the condition proves to be true. The third operand is what executes if the condition proves to be false. The conditional operator is represented as '?:'.
For example, if the C operator for conditional statement is utilized, the statement can be written as: (x<y)? printf("true"): printf("false"). If the values of the variables are x=10 and y=12, then the first expression will be executed. So, 'true' will be printed, because the condition was satisfied. If the condition was not satisfied, the third expression would be executed and false will be displayed.
Assignment operators
Assignment operators are used for allocating values to a variable.
The left side operand is a variable while the right-side operand is the value assigned to it. It must be noted that the value assigned must match the datatype highlighted on the variable side (left side) of the equation. Otherwise, the compiler might throw an error.
- “=”: This is the assignment operator. It is primarily used to assign the value on the right to the variable on the left.
- “-=”, "+=", "/=", "*=": These operators are the combination of ‘-‘, ‘'+', "/", and "*" with the equal to operators. This notation deducts the current value of the variable from the value assigned on the right. This result is then assigned to the variable on the left. For example, if v-=10, and v currently contains the value 12, then the above operator will perform the action v=12-10=2. So, 2 will be stored in v. Similarly, v+=10 would be 22. Similar executions can be done for the multiplication and division-based operators of the same type.
Bitwise Operators
The Bitwise operators are used to execute bit-level operations on the operand.
- The operators are first changed over to bit-level and afterward, the computation is performed on the operands.
- The numerical activities like add, sub, multiply and so forth can be performed at bit level for quicker handling.
- For example, the bitwise AND is represented 'a&b' as it takes 2 numbers as operands and does AND on every bit of two numbers.
- Let's look at two examples from bitwise OR and bitwise XOR. If 'a|b', and a=4 and b=5, then they are represented as 8-bit values and added (as OR corresponds to logical addition, just as AND corresponds to logical multiplication). So, the OR operation is as follows.
00000100 (4) + 00000101 (5) = 00001001 (9)
Similarly, XOR will return 'true' value (or 1) if and only if both values are different. So, the corresponding bits of the two operands must be different.
00000100 (4) + 00000101(5) = 00000001 (1)
Special operator
One example of a special unary operator is the size of() operator used in C. It is used to denote the size of an operand's value during compilation.
A Java Program to Illustrate use of Arithmetic Operators
class ArithmeticOperations {
public static void main(String args[]){
int x=9;
int y=3;
System.out.println(x+y);//12
System.out.println(x-b);//6
System.out.println(x*y);//27
System.out.println(x/y);//3
System.out.println(x%y);//0
}
}
Context and Applications
This topic is significant in the professional exams for both undergraduate and graduate courses, especially for
- B.Sc. in Computer science
- B.C.A
- M.C.A
- B.E Computer science / Information Science
- M.Tech. Computer science / Information Science
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Operators Homework Questions from Fellow Students
Browse our recently answered Operators homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.