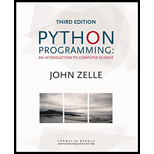
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 11, Problem 10PE
Program Plan Intro
Implementation of sieve
Program plan:
- Define the “sieve_era()” function,
- Create empty list.
- Create “while” loop,
- Append the first element to the new list using “append()” method.
- Make a “try” statement for exception.
- Create “for” loop,
- Check whether the list element is divided by the first element.
- If it is “True” remove the element.
- Create “for” loop,
- If any exception occurs, handle the exception,
- Keep the new list.
- Return new list.
- Define the “main()” function,
- Get the input from the user.
- Create empty list.
- Create “for” loop,
- Fill the new list using “append()” method.
- Assign the list return from “sieve_era()” function.
- Remove the element “4” from the list.
- Print the list.
- Call the “main()” function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given a list of integers, you want to know whether it is possible to divide the integers into two sets, so that the sum of one set is odd, and the sum of the other
set is a multiple of 10.
Every integer must be in one set or the other.
You can write a recursive helper method that takes any number of arguments and then call it inside the method, but you cannot use any loops.
Test cases:
oddAndTen([5, 5, 3])
true
oddAndTen ([5, 5, 4])
oddAndTen ([5, 5, 4, 1])
false
true
A prime number is an integer greater than 1 whose only positive divisors are 1 and the integer itself. The Greek mathematician Eratosthenes developed an algorithm, known as the Sieve of Eratosthenes, for finding all prime numbers less than or equal to a given number n—that is, all primes in the range 2 through n. Consider the list of numbers from 2 through n. Two is the first prime number, but the multiples of 2 (4, 6, 8,...) are not, and so they are crossed out in the list. Hie first number after 2 that was not crossed out is 3, the next prime. We then cross out from the list all higher multiples of 3 (6, 9, 12,…). The next number not crossed out is 5, the next prime, and so we cross out all higher multiples of 5 (10, 15, 20,…). We repeat this procedure until we reach the first number in the list that has not been crossed out and whose square is greater than n. All the numbers that remain in the list are the primes from 2 through n. Write a program that uses this sieve method and an…
Given a list of integers, we want to know whether it is possible to choose a subset of some of the integers, such that the integers in the subset adds up to the
given sum recursively.
We also want that if an integer is chosen to be in the sum, the integer next to it in the list must be skipped and not chosen to be in the sum.
Do not use any loops or regular expressions.
Test cases:
skipSum([2, 5, 10, 6], 12)
true
skipSum([2, 5, 10, 6], 7)
false
skipSum([2, 5, 10, 6], 16)
false
Given code:
public static boolean skipSum (List list, int sum) {
// call your recursive helper method
return skipSumHelper (list, e, sum);
1.
2.
3.
4.
Chapter 11 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 11 - Prob. 1TFCh. 11 - Prob. 2TFCh. 11 - Prob. 3TFCh. 11 - Prob. 4TFCh. 11 - Prob. 5TFCh. 11 - Prob. 6TFCh. 11 - Prob. 7TFCh. 11 - Prob. 8TFCh. 11 - Prob. 9TFCh. 11 - Prob. 1MC
Ch. 11 - Prob. 2MCCh. 11 - Prob. 3MCCh. 11 - Prob. 4MCCh. 11 - Prob. 5MCCh. 11 - Prob. 6MCCh. 11 - Prob. 7MCCh. 11 - Prob. 8MCCh. 11 - Prob. 9MCCh. 11 - Prob. 10MCCh. 11 - Prob. 1DCh. 11 - Prob. 2DCh. 11 - Prob. 1PECh. 11 - Prob. 2PECh. 11 - Prob. 3PECh. 11 - Prob. 5PECh. 11 - Prob. 6PECh. 11 - Prob. 7PECh. 11 - Prob. 8PECh. 11 - Prob. 9PECh. 11 - Prob. 10PECh. 11 - Prob. 11PECh. 11 - Prob. 12PECh. 11 - Prob. 15PECh. 11 - Prob. 16PECh. 11 - Prob. 18PECh. 11 - Prob. 19PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Suppose you have n two-digit numbers. For example, if n = 5, youmay have the following 5 two-digits number: 24 11 57 17 46You want to arrange the list in ascending order. Write an algorithm to perform the above task, ensuring the algorithm eventually stops and works with unusual data such as repeating numbers. Number each step of the algorithm, and explain briefly what its role isin achieving your goal. Pick a list of numbers, and show by hand how the algorithm will achieve the sorting required.arrow_forwardArtificial Intelligence (Part - 1) ==================== The Towers of Hanoi is a famous problem for studying recursion in computer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. (1) Propose a state representation for the problem?arrow_forwardArtificial Intelligence (Part - 2) ==================== The Towers of Hanoi is a famous problem for studying recursion incomputer science and searching in artificial intelligence. We start with N discs of varying sizes on a peg (stacked in order according to size), and two empty pegs. We are allowed to move a disc from one peg to another, but we are never allowed to move a larger disc on top of a smaller disc. The goal is to move all the discs to the rightmost peg (see figure). To solve the problem by using search methods, we need first formulate the problem. Supposing there are K pegs and N disk. (2) What is the size of the state space?arrow_forward
- The word ladder game was invented by Lewis Carroll in 1877. The idea is to begin with a start word and then change one letter at a time until you arrive at an end word. Each word along the way must be an English word. For example, starting from FISH, you can arrive at MAST through the following word ladder:FISH, WISH, WASH, MASH, MAST Write a program that uses recursion to find the word ladder given a start word and an end word, or that determines no word ladder exists. Use the file words.txt that is available online with the source code for the book as your dictionary of valid words. This file contains 87,314 words. Your program does not need to find the shortest word ladder between words; any word ladder will do if one exists. list aalii aardvark aardvarks aardwolf aba abaca abaci abacist aback abacus abacuses abaft abalone abalones abamp abampere abandon abandoned abandonee abandoner abandonersarrow_forwardIN JAVA Alice and Bob are playing a board game with a deck of nine cards. For each digit between 1 to 9, there is one card with that digit on it. Alice and Bob each draw two cards after shuffling the cards, and see the digits on their own cards without revealing the digits to each other. Then Alice gives her two cards to Bob. Bob sees the digits on Alice’s cards and lays all the four cards on the table in increasing order by the digits. Cards are laid facing down. Bob tells Alice the positions of her two cards. The goal of Alice is to guess the digits on Bob’s two cards. Can Alice uniquely determine these two digits and guess them correctly? Input The input has two integers p,q (1≤p<q≤9) on the first line, giving the digits on Alice’s cards. The next line has a string containing two ‘A’s and two ‘B’s, giving the positions of Alice’s and Bob’s cards on the table. It is guaranteed that Bob correctly sorts the cards and gives the correct positions of Alice’s cards. Output If Alice can…arrow_forwardWrite an algorithm that finds the m smallest numbers in a list of n numbers.arrow_forward
- Suppose N books of different sizes are placed on a table. You are asked to sort the books so that the books do not fall over easily as illustrated in the figure below. The only thing you are allowed to do is to pull out one book from anywhere in the way as the figure below and put it to place it again. Remember that you have quick eyes, you can immediately determine, which book is the smallest or largest to pull it out. a) Design an algorithm (a set of steps) to sort the books. b) This sorting problem is similar to a famous sorting algorithm. What is the name of this algorithm?arrow_forwardThe Fibonacci algorithm is a famous mathematical function that allows us to create a sequence of numbers by adding together the two previous values. For example, we have the sequence:1, 1, 2, 3, 5, 8, 13, 21…Write your own recursive code to calculate the nth term in the sequence. You should accept a positive integer as an input, and output the nth term of the sequence.Once you have created your code, add comments describing how the code works, and the complexity of any code you have created.arrow_forwardGiven a singly linked list, reverse the list. This means you have to reverse every node. For example if there are 4 nodes, the node at position 0 will move to position 3, the node at 1 will move to position 2 and so on. You do not have to write the Node class just write what you have been asked to. You are NOT allowed to create a new list. In python languagearrow_forward
- For the following problem please write an algorithm in plain English .i.e give details as to how you will solve the problem.A deck of 52 playing cards (as used for playing bridge) has to be sorted. At the end of the attempt, the sorted deck of cards should be on the table with the backside up.The order within a suite is Ace - King - Queen - Jack- 10 -9 - 8 - 7- 6- 5- 4- 3- 2. The very first card in the sorted deck is the Ace of Clubs, the next ones are the King of Clubs, the Queen of Clubs, the Jack of Clubs, the 10 of Clubs ... down to the 2 of Clubs. The next card is Ace of Spades, followed by the King of Spades etc. The hearts and diamonds cards follow in the same order.The deck of cards must be well-shuffled immediately prior to the challenge.Please write the algorithm in steps like you write a recipe for a dish.If any steps need to be repeated try to use a loop.Please try to not use any programming language.(IT's one question just with a lot of instructions to be understood well)arrow_forwardCorrect answer will be upvoted else downvoted. Computer science. section grouping is a string containing just characters "(" and ")". A standard section succession is a section grouping that can be changed into a right number juggling articulation by embedding characters "1" and "+" between the first characters of the arrangement. For instance, section successions "()()" and "(())" are normal (the subsequent articulations are: "(1)+(1)" and "((1+1)+1)"), and ")(", "(" and ")" are not. You need to find a string b that comprises of n characters to such an extent that: b is a standard section grouping; in the event that for some I and j (1≤i,j≤n) ai=aj, bi=bj. As such, you need to supplant all events of 'A' with a similar kind of section, then, at that point, all events of 'B' with a similar sort of section and all events of 'C' with a similar sort of section. Your undertaking is to decide whether such a string b exists. Input The primary line contains a solitary…arrow_forwardEach year, the government releases a list of the 10,000 most common baby namesand their frequencies (the number of babies with that name). The only problem with this is that some names have multiple spellings. For example, "John" and ''.Jon" are essentially the same name but would be listed separately in the list. Given two lists, one of names/frequencies and the other of pairs of equivalent names, write an algorithm to print a new list of the true frequency of each name. Note that if John and Jon are synonyms, and Jon and Johnny are synonyms, then John andJohnny are synonyms. (It is both transitive and symmetric.) In the final list, any name can be usedas the "real" name.EXAMPLEInput:Names: John (15), Jon (12), Chris (13), Kris (4), Christopher (19)Synonyms: (Jon, John), (John, Johnny), (Chris, Kris), (Chris, Christopher)Output: John (27), Kris (36)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
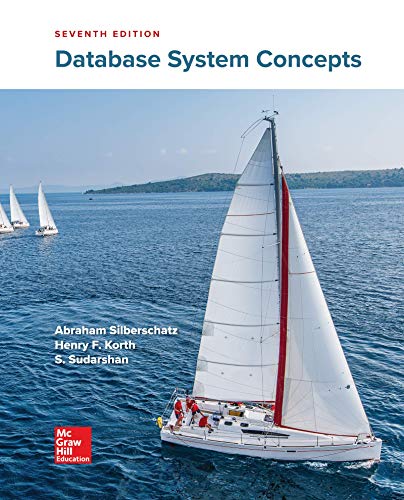
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
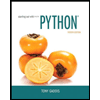
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
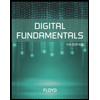
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
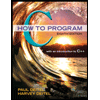
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
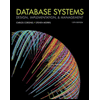
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
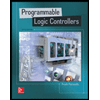
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7); Author: CS Dojo;https://www.youtube.com/watch?v=D6xkbGLQesk;License: Standard YouTube License, CC-BY