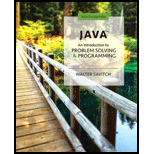
Explanation of Solution
 Modified
 The below highlighted parts are the modified codes in the server and client programs.
 Server program:
//import required header files
import java.util.Scanner;
import java.io.InputStreamReader;
import java.io.DataOutputStream;
import java.io.PrintWriter;
import java.net.Socket;
import java.net.ServerSocket;
//definition of "SocketServer" class
public class SocketServer
{
    //definition of main method
    public static void main(String[] args)
    {
        //declare the objects for the classes
        Scanner inputStream = null;
        PrintWriter outputStream = null;
        ServerSocket serverSocket = null;
        //try block
        try
        {
            // wait for connection on port 6789
System.out.println("Waiting for a connection.");
            serverSocket = new ServerSocket(6789);
            Socket socket = serverSocket.accept();
            // connection made, set up streams
inputStream = new Scanner(new InputStreamReader(socket.getInputStream()));
outputStream = new PrintWriter(new DataOutputStream(socket.getOutputStream()));
            //get the input from the client
            int i = inputStream.nextInt();
            int j = inputStream.nextInt();
            //send the output to the client
            outputStream.println(i + j);
            //flush the stream Â
            outputStream.flush();
            //display the statement
System.out.println("The connection is closed ");
            //close the connections
            inputStream.close();
            outputStream.close();
        }
        //catch block
        catch (Exception e)
        {
            // display the exception
            System.out.println("Error " + e);
        }
    }
}
 Client program:
//import required header files
import java...

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- In this task, you should: create a CustomHTTPServer class inherited from the HTTPServer class. In the constructor method, the CustomHTTPServer class sets up the server address and port received as a user input. In the constructor, your web server's RequestHandler class has been set up. Every time a client is connected, the server handles the request according to this class. The RequestHandler defines the action to handle the client's GET request. It sends an HTTPheader (code 200) with a success message Hello from server! using the write() method.arrow_forwardWrite a client program for MyTime which loops around getting strings fromthe user representing times and responding with a greeting such as “goodmorning” as appropriate. The user should enter the string “quit” to exit theprogram.  EXAMPLE run of client:USER: 2:07 pmPROGRAM: good afternoonUSER: 10:71 amPROGRAM: that’s not a valid time, please re-enterUSER: 14:30amPROGRAM: that’s not a valid time, please re-enterUSER: 10:21amPROGRAM: good morningUSER: 7:00 pmPROGRAM: good evening  the set method for MyTime should detect inputstrings which do not represent valid times. An exception should be thrown insuch a case. Clients will haveto handle (or catch) the exceptions. Thus you will have to also write your own exception class which can be used by MyTime and the client class. Here are my code: public class MyTime { private String time; public String getTime() { return time; } public void setTime() throws InvalidTime { String time = askTime(); if (time.equalsIgnoreCase("quit")) {…arrow_forwardWrite a client program for MyTime which loops around getting strings fromthe user representing times and responding with a greeting such as “goodmorning” as appropriate. The user should enter the string “quit” to exit theprogram.EXAMPLE run of client:USER: 2:07 pmPROGRAM: good afternoonUSER: 10:71 amPROGRAM: that’s not a valid time, please re-enterUSER: 14:30amPROGRAM: that’s not a valid time, please re-enterUSER: 10:21amPROGRAM: good morningUSER: 7:00 pmPROGRAM: good evening set method for MyTime should detect inputstrings which do not represent valid times. An exception should be thrown insuch a case. Clients (such as the one you are writing for question 2) will haveto handle (or catch) the exceptions. Thus you will have to also write your ownexception class which can be used by MyTime and the client class.  public class MyTime { public MyTime() { } private String time; public String getTime() { return time; } public void setTime() throws InvalidTime { String time = askTime(); if…arrow_forward
- Write a Java application for a server that listens to a client. The server should run on port 8250. Once the server is running it should output “Server is starting….”, then it should wait to receive a message from the client. Once it receives the message, it should close the socket. Create a client that sends a message to the server through port 8250. The message that should be sent tothe server should be the sum of two numbers. Once it sends the message, it should close the socket. output: run: Server is starting... Message received from client: 4+3=7arrow_forwardWrite a Java application for a server that listens to a client. The server should run on port 8250. Once the server is running it should output “Server is starting….”, then it should wait to receive a message from the client. Once it receives the message, it should close the socket. Create a client that sends a message to the server through port 8250. The message that should be sent tothe server should be the sum of two numbers. Once it sends the message, it should close the socket. Figure 2(attached) shows messages from the server:arrow_forwardWrite a Java application for a server that listens to a client. The server should run on port 8250. Once the server is running it should output “Server is starting….”, then it should wait to receive a message from the client. Once it receives the message, it should close the socket. Create a client that sends a message to the server through port 8250. The message that should be sent to the server should be the sum of two numbers. Once it sends the message, it should close the socket. Figure 2 shows messages from the server:arrow_forward
- In Java programming Write the code to print out a customer report. Your code must read each customer from a file, populate the object, place the object in a linked list, and display the data from the linked list.  Use the customer object name from Customer customer = new Customer ( “ customer name” ); Assume you have opened a file and read a line into the customer object. Write the signature line for this method Write the line of code that will write the object to a linked list Write the line of code that will print the customer object properties. Be sure to include a textual description of each property.arrow_forwardHow does a server know that one of the far-off objects he gives his clients could be collected since it is no longer in use?arrow_forwardNeed help with this code: You will write a client-server system for writing/storing notes. Each note has an ID (integer), a title (short text), and a body (long text). A client can have three kinds of requests to the server: upload a note, download a note (given its ID), and search for a note (given a keyword). In the case of search, the server will return a list of IDs and titles of notes whose titles contain the keyword. Design the database to store the notes. Design the Ul of the client. • Write code to implement the system. • You should use design patterns as in the Store Management System: 3-layer; Model-View-Controller; Singleton, Adapter. 1. StoreManager code public class StoreManager { private static StoreManager instance = null; private RemoteDataAdapter dao; private ProductView productView = null; public ProductView getProductView() { return productView; } private ProductController productController = null; public static StoreManager getlnstance() { if (instance == null)…arrow_forward
- Focus on string operations and methodsYou work for a small company that keeps the following information about its clients: • first name• last name • a 5-digit user code assigned by your company.The information is stored in a file clients.txt with the information for each client on one line(last name first), with commas between the parts. For exampleJones, Sally,00345Lin ,Nenya,00548Fule,A,00000Smythe , Mary Ann , 00012Your job is to create a program assign usernames for a login system. First: write a function named get_parts(string) that will that will receive as its arguments a string with the client data for one client, for example “Lin ,Nenya,00548”, and return the separate first name, last name, and client code. You should remove any extra whitespace from the beginning and newlines from the end of the parts.You’ll need to use some of the string methods that we covered in this lessonYou can test your function by with a main() that is just the function call with the argument typed…arrow_forwardFocus on string operations and methodsYou work for a small company that keeps the following information about its clients: • first name• last name • a 5-digit user code assigned by your company.The information is stored in a file clients.txt with the information for each client on one line(last name first), with commas between the parts. For exampleJones, Sally,00345Lin ,Nenya,00548Fule,A,00000Smythe , Mary Ann , 00012Your job is to create a program assign usernames for a login system. First: write a function named get_parts(string) that will that will receive as its arguments a string with the client data for one client, for example “Lin ,Nenya,00548”, and return the separate first name, last name, and client code. You should remove any extra whitespace from the beginning and newlines from the end of the parts.You’ll need to use some of the string methods that we covered in this lessonYou can test your function by with a main() that is just the function call with the argument typed…arrow_forwardPLEASE USE MULTITHREADING IN THIS PROGRAM SO THAT MANY CLIENTS CONNECTED TO THE SERVER AND EACH CLIENT CAN SEND MESSAGE TO SERVER AND SERVER MESSAGE SHOWN TO ALL CLIENTS AND ONE CLIENT MESSAGE SHOWN TO ALL CLIENTS.PLEASE MAKE CHANGINGS IN THIS CODE. SERVER #include <stdio.h>#include <stdlib.h>#include <string.h>#include <unistd.h>#include <sys/socket.h>#include <sys/types.h>#include <netinet/in.h>#include <arpa/inet.h>#include <pthread.h>#define PORT 5500 void func(int connfd){  char buffer[1024];  int n;  while(1) {     bzero(buffer, 1024);     read(connfd, buffer, sizeof(buffer));     printf("From client: %s\t To client : ", buffer);     bzero(buffer, 1024);     n = 0;     while ((buffer[n++] = getchar()) != '\n');     write(connfd, buffer, sizeof(buffer));     if (strncmp("exit", buffer, 4) == 0){     printf("Server Exit...\n");     break;  }    }}int main(){  int sockfd, connfd, len;  struct…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
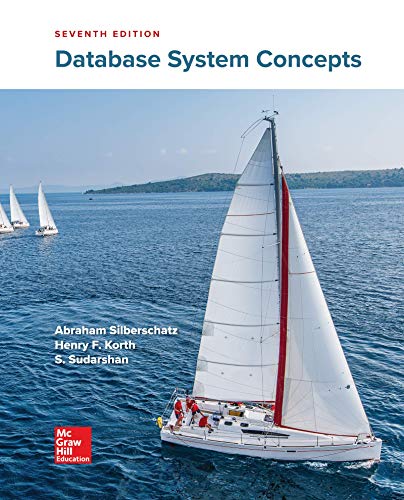
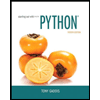
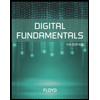
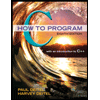
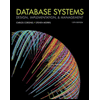
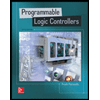