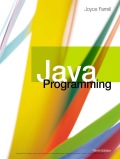
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 6PE
Program Plan Intro
Base ball game
Program plan:
Filename: “DemoBaseballGame.java”
- Include the required header files
- Define the class “DemoBaseballGame”
- Define the main method
- Create an object for “BaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the winning name
- Otherwise, check “total [0]” is less than “total [1]”
- Display the winning name
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Filename: “BaseballGame.java”
- Define the “BaseballGame” class
- Declare the required variables
- Define the constructor
- Declare an array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores [t]” length
- Set the value
- Iterate “i” until it reaches “scores [t]” length
- Define the “setNames” method
- Set the values
- Define the “setScore” method
- Declare the required variables
- Check “team” is less than 0 or greater than “scores” length minus 1
- Display the team is valid
- Check “inning” is less than 0 or greater than “scores” length minus 1
- Display the team is not valid
- Otherwise,
- Iterate “x” until it reaches “inning”
- Check the condition
-
- Set the value
- Check the condition
- Display a score can’t yet be set for inning
- Otherwise, set the value
- Iterate “x” until it reaches “inning”
- Define the “setNames” method
- Return the names
- Define the “getNames” method
- Return the position of the name
- Define the “getScores” method
- Declare the variable
- Check “team” is less than 0 or greater than “scores” length minus 1
- Display invalid team number
- Check “inning” is less than 0 or greater than “scores[0]” length minus 1
- Display invalid inning
- Otherwise, set the value
- Define “getInnings” method
- Return innings
Filename: “HighSchoolBaseballGame.java”
- Declare the variable and assign the value
- Define the constructor
- Declare the array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores[t]” length
- Set the value
- Iterate “i” until it reaches “scores[t]” length
- Define “getInnings” method
- Return innings
Filename: “LittleLeagueBaseballGame.java”
- Declare the variable and assign the value
- Define the constructor
- Declare the array variable
- Iterate “t” until it reaches “scores” length
- Iterate “i” until it reaches “scores[t]” length
- Set the value
- Iterate “i” until it reaches “scores[t]” length
- Define “getInnings” method
- Return innings
Filename: “DemoLLBaseballGame.java”
- Include the required header files
- Define the class “DemoLLBaseballGame”
- Define the main method
- Create an object for “LittleLeagueBaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Otherwise, set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the team 1 is win
- Otherwise, check “total [0]” is less than “total [1]”
- Display the team 2 is win
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Filename: “DemoHSBaseballGame.java”
- Include the required header files
- Define the class “DemoHSBaseballGame”
- Define the main method
- Create an object for “HighSchoolBaseballGame” class
- Call the method “SetNames” for setting the names
- Call the method “display”
- Call the “setScore” method for setting the score
- After setting the score, call the “display” method
- Call the “setScore” method in fifth inning
- Call the “setScore” method after setting second inning
- Call the “setScore” method for invalid inning
- Call the “setScore” method for invalid team
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “setScore” method
- Iterate the “i” value until it reaches “getInnings”
- Call the “display” method
- Define the “display” method
- Declare the required variables
- Display the teams
- Iterate the “t” value until it reaches “2”
- Iterate the “i” value until it reaches “getInnings”
- Call the “getScore” method
- Check “score” is not equal to “BaseballGame.UNPLAYED”
-
- Check the “i” is equal to 0
- Display the team
- Display the score
- Calculate the total score
-
- Otherwise, set the value
-
- Check the “i” is equal to 0
- Iterate the “i” value until it reaches “getInnings”
- Check the condition
- Display no innings played yet
- Check the condition
- Check “total[0]” is greater than “total[1]”
- Display the team 1 is win
- Otherwise, check “total [0]” is less than “total [1]”
- Display the team 2 is win
- Otherwise, display game is tie.
- Check “total[0]” is greater than “total[1]”
- Define the main method
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
A cylinder of diameter 10 cm rotates concentrically inside another hollow
cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their
axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to
rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poise
Make the following game user friendly with GUI, with some simple graphics
The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units.
Cell Class:
public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…
Please original work
What are four of the goals of information lifecycle management think they are most important to data warehousing,
Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each.
Please cite in text references and add weblinks
Chapter 10 Solutions
EBK JAVA PROGRAMMING
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forward
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
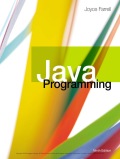
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
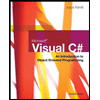
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY