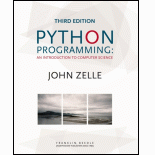
Concept explainers
Three Button Monte
Program Plan:
- Import the required packages.
- Declare a gameplay function. Inside the function,
- Create the application window using the “button.py” module.
- Activate the doors.
- Get the random number.
- Assign the values to “false”.
- Check the condition.
- Assign “point1” to “true”.
- Check the condition.
- Assign the “point2” to “true”.
- Otherwise, Assign the “point3” to “true”
- Activate all the three doors.
- Get the action mouse clicked.
- Check the condition using “while” loop.
- Check the condition for selecting the door 1 to be clicked.
- Check the condition for selecting the door 2 to be clicked.
- Check the condition for selecting the door 3 to be clicked.
- Definition of “printSummary()”.
- Print the result.
- Definition of main function.
- Creating the application window by setting title, cords and background.
- Define “Quit” button and set to active.
- Assign the text to the interface.
- Call the method draw.
- Initialize variables to enter loop
- Check the condition for “Quit” not clicked.
- Call the method “gameplay()”
- Call the method “printSummary()”
- Call the main function.

Explanation of Solution
Program:
Refer the program “button.py” given in the “Chapter 10” from the text book. Add the method “update()” along with the given code.
#Define the method update
def update(self, win, label):
#Call the method undraw()
self.label.undraw()
#Assign the position to centre
center = self.center
#Assign the label
self.label = Text(center, label)
#Set active to false
self.active = False
#Call the method draw()
self.label.draw(win)
Main.py:
#Import required packages
from button import Button
from graphics import GraphWin, Point, Text
from random import random
from time import sleep
#Definition of gameplay method
def gameplay(click, win, wins, losses):
#use button.py module to create doors and activate them
door1 = Button(win, Point(-7.5, -3), 5, 6, "Door 1")
door2 = Button(win, Point(0, -3), 5, 6, "Door 2")
door3 = Button(win, Point(7.5, -3), 5, 6, "Door 3")
#Activate the doors
door1.activate()
door2.activate()
door3.activate()
#Get the random number
x = random() * 3
#Assign the values to false
point1 = point2 = point3 = False
#check the condition
if 0 <= x <1:
#Assign point1 to True
point1 = True
#Check the condition
elif 1 <= x < 2:
#Assign the point2 to True
point2 = True
#Otherwise
else:
#Assign the point2 to True
point3 = True
#Get the action mouse click
click = win.getMouse()
#Check the condition using "while" loop
while door1.clicked(click) or door2.clicked(click) or door3.clicked(click):
#If door1 is clicked
if door1.clicked(click):
#Assign point1 to true
if point1 == True:
#Update the interface
door1.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door1.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the losses
losses = losses + 1
break
#Check the door2 is clicked
elif door2.clicked(click):
#Assign point2 to true
if point2 == True:
#Update the interface
door2.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door2.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the value of losses
losses = losses + 1
break
else:
if point3 == True:
door3.update(win,"Victory!")
#Call the method sleep
sleep(1)
#Increment the value of wins
wins = wins + 1
#Otherwise
else:
#Update the interface
door3.update(win,"Try Again")
#Call the method sleep
sleep(1)
#Increment the value of losses
losses = losses + 1
break
#Return the values
return click, wins, losses
#Definition of printSummary
def printSummary(wins, losses):
#Print the result
print("Wins: {0:5} Losses: {1:5}".format(wins, losses))
#Definition of main
def main():
#Creating the application window by setting title, cords and background
win = GraphWin("Three Button Monte", 500, 300)
win.setCoords(-12, -12, 12, 12)
win.setBackground("green3")
#define Quit button and set to active
gameover = Button(win, Point(9, 10), 3, 3, "Quit")
gameover.activate()
#Assign the text to the interface
direction = Text(Point(0, 10), "Pick a Door")
#Call the method draw
direction.draw(win)
#initialize variables to enter loop
click = Point(0,0)
wins = losses = 0
#Check the condition
while not gameover.clicked(click):
#Call the method
click, wins, losses = gameplay(click, win, wins, losses)
#Call the method printSummary
printSummary(wins, losses)
#Call the main function
main()
Output:
Screenshot of output
Clicking the “Exit” button:
Clicking the “Door1”, “Door2”, “Door3” will displays the opened door leads to “Victory!” or “Try Again”. After clicking the “Exit” button it prints the count of the corresponding result.
Wins: 1 Losses: 2
Want to see more full solutions like this?
Chapter 10 Solutions
Python Programming: An Introduction to Computer Science
- Do as directed. There is an application in which user may check subject check box and enter subject marks in relevant text field. In case of checking check box relevant text field state is changed to editable and by unchecking check box relevant text field state is changed to read only, you have to Write a code for “Total and Average Quality Points” button in which you have to calculate total points, average point and show in relevant fields. Also write code for click event of check boxes. Average Points = Total Points/Number of Coursesarrow_forwardWrong answer would be downvoted. Attach output screen with code Don't copyarrow_forwardPlease add reset button or a button that will clear all things on textfield i cant do it i am receiving an errorarrow_forward
- When the player clicks on the “Start Game” button, in every 1 seconds, three random alphabets will be generated and flashing on the screen (e.g. A X W) at the position as shown above. The three alphabets must not repeat at the same time (i.e. can’t have repeating alphabets – e.g. A X A). After starting a game, you can’t change the chosen alphabet.arrow_forwardUse method which take a string parameter. Method must be named hasRepeat and string parameter. Don't reject please And give me write code to fill. Attach screenshot as wellarrow_forwardNote: Code with comments and output screenshot is must else answer will be downvoted. Thank youarrow_forward
- The goal of Snake is to create a snake as long as possible. This is achieved by guiding the snake to an apple on the game board. The snake cannot stop moving, and dies whenever it hits something (excluding apples). Because the snake is growing longer and longer as the game progresses, it gets increasingly difficult to avoid collisions with the snake itself. The player can change the direction of the head of the snake by using the arrow keys. At step in the game, there is always an apple somewhere on the board. If the snake eats an apple, the snake becomes one cell longer. A new apple is placed on a random location, excluding all places covered by the snake. When the snake reaches a side of the game board, it re-emerges at the opposite end.arrow_forwardWrite a MessageBox.Show method that displays “You win!” as the message, “Game Over” in the title bar, an OK button, and an Information icon.arrow_forwardYou are creating a simple RPG video game, and you need to work out some details for your crafting menu. Your characters can craft two potions with simple ingredients: Minor Mana, and Minor Healing. The two potions have similar ingredients with some slight differences shown below. You want to add a "Craft All Potions" option, which will ask the user if they would like to prioritize Healing or Mana potions. It will then tell you how many you can produce of your priority potion, and then with the leftovers it will craft the other type of potion if it is possible. First ask for the priority potion, and then the quantities of the ingredients as an input. Then output how many of the priority potion you can make, and how many of the other potion. If the user provides an invalid input, ask them again.arrow_forward
- Write an application that counts by five from 5 through 500 inclusive and that starts a new line after every multiple of 50 (50,100,150 and so on).Save the file as CountByAnything.javaarrow_forwardModify the Dice Poker program from this chapter to include any or all of the following features: 1- Splash Screen. When the program first fires up, have it print a short introductory message about the program and buttons for "Let's Play" and "Exit." The main interface shouldn't appear unless the user se- lects "Let's Play." 2-Add a "Help" button that pops up another window displaying the rules of the game (the payoffs table is the most important part). 3-Add a high score feature. The program should keep track of the 10 best scores. When a user quits with a good enough score, he/she is invited to type in a name for the list. The list should be printed in the splash screen when the program first runs. The high-scores list will have to be stored in a file so that it persists between program invocations.arrow_forwardPLEASE READ-THIS IS NOT FOR A GRADE!⚠️⚠️⚠️ Using the code below how would you code a Program that should display the average for the first semester and the second semester.Also posted an example Code: import tkinter # Let's create the Tkinter window. window = tkinter.Tk() window.title("GUI") # You will first create a division with the help of Frame class and align them on TOP and BOTTOM with pack() method. top_frame = tkinter.Frame(window).pack() bottom_frame = tkinter.Frame(window).pack(side = "bottom") # Once the frames are created then you are all set to add widgets in both the frames. btn1 = tkinter.Button(top_frame, text = "Button1", fg = "red").pack() #'fg or foreground' is for coloring the contents (buttons) btn2 = tkinter.Button(top_frame, text = "Button2", fg = "green").pack() btn3 = tkinter.Button(bottom_frame, text = "Button3", fg = "purple").pack(side = "left") #'side' is used to left or right align the widgets btn4 = tkinter.Button(bottom_frame, text =…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage