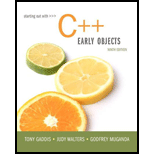
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 32RQE
Program Plan Intro
Shared pointers:
- A “shared_ptr” is a smart pointer which maintains shared ownership of an object using pointer.
- Shared pointers are used in managing the ownership of the dynamically allocated object.
- Shared pointers are used by more than one owner available for the objects that are allocated dynamically.
Example:
The following code illustrates the use and creation of shared pointer:
//creating a shared pointer of integer data type
shared_ptr<int> sDPtr1 (new int);
//creating an array of shared pointer
shared_ptr<int[]> sDPtr2 (new int[5]);
Explanation:
In the above code snippet, a smart pointer of integer data type is assigned directly.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A cylinder of diameter 10 cm rotates concentrically inside another hollow
cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their
axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to
rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poise
Make the following game user friendly with GUI, with some simple graphics
The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units.
Cell Class:
public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…
Please original work
What are four of the goals of information lifecycle management think they are most important to data warehousing,
Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each.
Please cite in text references and add weblinks
Chapter 10 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 10.5 - Prob. 10.1CPCh. 10.5 - Write a statement defining a variable dPtr. The...Ch. 10.5 - List three uses of the symbol in C++.Ch. 10.5 - What is the output of the following program?...Ch. 10.5 - Rewrite the following loop so it uses pointer...Ch. 10.5 - Prob. 10.6CPCh. 10.5 - Assume pint is a pointer variable. For each of the...Ch. 10.5 - For each of the following variable definitions,...Ch. 10.10 - Assuming array is an array of ints, which of the...Ch. 10.10 - Give an example of the proper way to call the...
Ch. 10.10 - Complete the following program skeleton. When...Ch. 10.10 - Look at the following array definition: const int...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Assume ip is a pointer to an int. Write a...Ch. 10.10 - Prob. 10.15CPCh. 10.10 - Prob. 10.16CPCh. 10.10 - Prob. 10.17CPCh. 10.12 - Prob. 10.18CPCh. 10.12 - Assume the following structure declaration exists...Ch. 10.12 - Prob. 10.20CPCh. 10 - Each byte in memory is assigned a unique _____Ch. 10 - The _____ operator can be used to determine a...Ch. 10 - Prob. 3RQECh. 10 - The _____ operator can be used to work with the...Ch. 10 - Prob. 5RQECh. 10 - Creating variables while a program is running is...Ch. 10 - Prob. 7RQECh. 10 - If the new operator cannot allocate the amount of...Ch. 10 - Prob. 9RQECh. 10 - When a program is finished with a chunk of...Ch. 10 - You should only use the delete operator to...Ch. 10 - What does the indirection operator do?Ch. 10 - Look at the following code. int X = 7; int ptr =...Ch. 10 - Name two different uses for the C++ operator.Ch. 10 - Prob. 15RQECh. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - What is the purpose of the new operator?Ch. 10 - What happens when a program uses the new operator...Ch. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - Prob. 25RQECh. 10 - Prob. 26RQECh. 10 - What happens when a unique_ptr that is managing an...Ch. 10 - What does the get ( ) method of the unique_ptr...Ch. 10 - Prob. 29RQECh. 10 - Prob. 30RQECh. 10 - Prob. 31RQECh. 10 - Prob. 32RQECh. 10 - Consider the function void change(int p) { P = 20;...Ch. 10 - Prob. 34RQECh. 10 - Write a function whose prototype is void...Ch. 10 - Write a function void switchEnds(int array, int...Ch. 10 - Given the variable initializations int a[5] = {0,...Ch. 10 - Each of the following declarations and program...Ch. 10 - Prob. 39RQECh. 10 - Test Scores #1 Write a program that dynamically...Ch. 10 - Test Scores #2 Modify the program of Programming...Ch. 10 - Indirect Sorting Through Pointers #1 Consider a...Ch. 10 - Indirect Sorting Through Pointers #2 Write a...Ch. 10 - Pie a la Mode In statistics the mode of a set of...Ch. 10 - Median Function In statistics the median of a set...Ch. 10 - Movie Statistics Write a program that can be used...Ch. 10 - Days in Current Month Write a program that can...Ch. 10 - Age Write a program that asks for the users name...Ch. 10 - Prob. 10PC
Knowledge Booster
Similar questions
- The following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forward
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
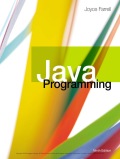
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT