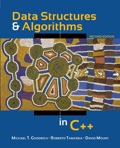
Concept explainers
Explanation of Solution
Program code:
main.cpp
//include the requird header files
#include <
#include "CreditCard.h"
//use the namespace
using namespace std;
//define the function textCard()
void testCard()
{
// vector of CC pointers
vector <CreditCard*> wallet(10);
//create the credit card details
wallet[0] = new CreditCard(5391037593875309,"JoBlo",2500);
wallet[1] = new CreditCard(3485039933951954,"JoBlo",3500);
wallet[2] = new CreditCard(6011490232942994,"JoBlo",5000);
//iterate a for loop
for (int i = 0; i < 3; i++)
{
//iterate a for loop
for (int j = 1; j <= 16; j++)
{
// explicit cast
wallet[0] -> chargeIt(double(i));
// implicit cast
wallet[1] -> chargeIt(2.0 * i);
wallet[1] -> chargeIt(2.0 * i);
}
//print the card details
cout << *wallet[i];
//iterate a while loop to get the balance
while (wallet[i]->getBalance() > 100.0)
{
//call the method makePayment
wallet[i]->makePayment(100.0);
//print the new balance
cout << "New balance = " <<
wallet[i]->getBalance() << "\n";
}
//deleting a walllet
delete wallet[i];
}
}
//define the main() method
int main()
{
//First test case
CreditCard CC = CreditCard(1234, "Bill gates", 2500);
CC.chargeIt(1000);
CC.chargeIt(-100);
CC.chargeIt(500);
CC.makePayment(-100);
CC.makePayment(300);
cout << CC << endl << endl;
//given test case
testCard();
return EXIT_SUCCESS;
}
Explanation:
In the code,
- Include the required header files.
- Use the namespace.
- Define the “testCard()” method.
- Create vector pointers
- Create array “wallet[]” to store credit card details.
- Iterate a “for” loop.
- Iterate a “for” loop.
- Explicit cast.
- Implicit cast.
- Print the card details.
- Iterate a “while” loop to get the balance.
- Call the method “makepayment()”.
- Print the new balance.
- Delete a wallet.
- Iterate a “for” loop.
- Define the “main()” function.
- Enter the credit card details.
- Call the function “chargeIt()” and “makePayment()” using “CC”.
- Call the function “testCard()”.
- Return “EXIT_SUCCESS”.
CreditCard.cpp
//Code for CreditCard.cpp
#include "CreditCard.h"
using namespace std;
//define the constructor CreditCard
CreditCard::CreditCard(long long int no, const string& nm, int lim, double bal)
{
//declare the required variables
number = no;
name = nm;
limit = lim;
balance = bal;
}
//define a function chargeIt()
bool CreditCard::chargeIt(double price)
{
if(price <= 0)
{
//print error message when price is negative
cout << "Error: Input argument must be positive.\n";
//return false
return false;
}
//if the condition is true
if (price + balance > double(limit))
{
//return false
return false;
}
//add price to balance
balance += price;
//update fraction
fraction = double(balance)*100.0/limit;
//if the condition is true
if(fraction > FRACTION_LIMIT)
{
//print warning message when fraction is more than 50%
printf("Warning: the current balance on credit card %lld is %.2f%c of your limit.\n", number, fraction, '%');
}
// the charge goes through
return true;
}
//define the function makePayment
void CreditCard::makePayment(double amount)
{
//create a variable
const double interestRate = 0.10;
//if amount less than or equal to 0
if(amount <= 0)
{
//return
return;
}
//set the value of balance
balance -= amount * (1 + interestRate);
}
ostream& operator<<(ostream& out, const CreditCard& c)
{
//print method
out << "Number = " << c.getNumber() << "\n"
<< "Name = " << c.getName() << "\n"
<< "Balance = " << c.getBalance() << "\n"
<< "Limit = " << c.getLimit() << "\n";
return out;
}
Explanation:
In the code,
- Include the required header files.
- Use the namespace.
- Define the constructor “creditCard()”.
- Declare the required variables.
- Define the function “chargeIt()”.
- If the value of the “price” is less than or equal to 0.
- Print an error message that the price must be positive.
- Return a value “false”.
- If the condition is true,
- Return false.
- Add the value of “price” to “balance”.
- Update the value of “fraction”.
- If the condition “fraction > FRACTION_LIMIT” is true.
- Print a warning message.
- Return true.
- If the condition is true,
- If the value of the “price” is less than or equal to 0.
- Define the function “makePayment()”.
- Create a variable “interestrate”.
- If the value of the “amount” is less than or equal to 0.
- Return.
- Set the value of “balance”.
- Return.
- Print the details.
- Enter the credit card details.
- Call the function “getNumber()” and “getName()”,“getBalance()” ,and “getLimit()”.
- Call the function “testCard()”.
- Return “out”.
CreditCard.h
//Code for CreditCard.h:
#ifndef CreditCard_H
#define CreditCard_H
#include<iostream>
//interest rate
#define INTEREST 5
//fraction limit
#define FRACTION_LIMIT 50.00
//define a class
class CreditCard
{
//public access specifier
public:
//class member functions
CreditCard(long long int no, const std::string& nm,
int lim, double bal = 0);
long long int getNumber() const
{
return number;
}
std::string getName() const
{
return name;
}
double getBalance() const
{
return balance;
}
int getLimit() const
{
return limit;
}
// make a charge
bool chargeIt(double price);
// make a payment
void makePayment(double amount);
//private access specifier
private:
//class members
// credit card number
long long int number;
// card owner's name
std::string name;
// the current balance
double balance;
// the credit limit
int limit;
//maintains the fraction
double fraction;
};
// print card information
std::ostream& operator<<(std::ostream& out, const CreditCard& c);
#endif
Explanation:
In the code,
- Include the required header files...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
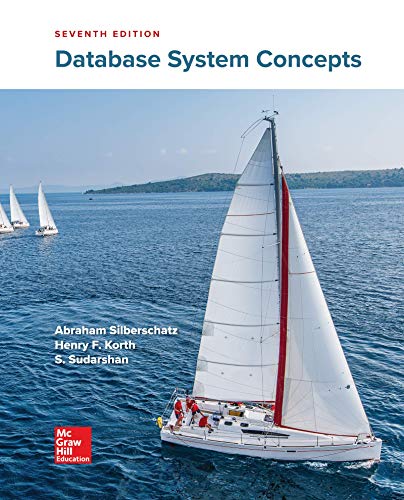
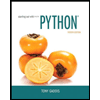
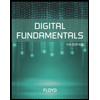
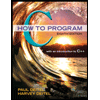
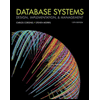
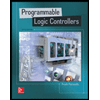