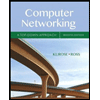
Concept explainers
Your task for this assignment is to identify a spanning tree in one connected undirected
weighted graph using C++.
1. Implement a spanning tree
subgraph of the of a connected undirected weighted graph. The program is interactive.
Graph edges with respective weights (i.e., v1 v2 w) are entered at the command line and
results are displayed on the console.
2. Each input transaction represents an undirected edge of a connected weighted graph. The
edge consists of two unequal non-negative integers in the range 0 to 9 representing graph
vertices that the edge connects. Each edge has an assigned weight. The edge weight is a
positive integer in the range 1 to 99. The three integers on each input transaction are
separated by space. An input transaction containing the string “end-of-file” signalsthe end
of the graph edge input. After the edge information is read, the process begins. Use an
adjacency matrix for recording input edges and show all code used to determine the
spanning tree. The input data can be assumed to be valid, so is no need to perform data
validation on the input data.
3. After the edges of the spanning tree are determined, the tree edges are displayed on the
console, one edge per output line, following the message: “Spanning tree:”. Each output
line representing a tree edge contains two integers separated by space. These integers are
the two vertices representing the edge.
Sample input transactions are as follows:
1 3 81
3 2 62
1 2 73
end-of-file
Sample output expected after processing the above input will be as follows:
Spanning tree:
1 3
2 3
2
4. The program will be run at the command prompt by navigating to the directory containing
the executable version of the program after the program is compiled.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- I need help coding this in java language.arrow_forwardPlease help with the program below. Need to write a program called dfs-stack.py in python that uses the algorithm below without an agency list but instead uses an adjacency matrix. The program should prompt the user for the number of vertices V, in the graph.Please read the directions below in the image I will post a picture of the instructions and the algorithm.arrow_forwardpublic interface StackInterface void push (T element) throws StackoverfloWException; void pop () throws StackUnderflowException; T top () throws StackUnderflowException; boolean isFul1 (); boolean isEmpty(); Show what is written by the following segments of code (see printın on the code), given that iteml, item2, and item3 are int variables, and ali is an object that fits the abstract description of a stack as shown above StackInterface. Assume that you can store and retrieve variables of type int on ali. iteml = 2; item2 = 0; item3 = 6; ali.push (item2); ali.push (item1); ali.push ( iteml+item3); item2 = ali.top ( ); ali.push (item3*item3); ali.push (item2); ali.push (3); iteml = ali.top ( ); ali.pop( ); System.out.println (iteml + " " + item2 + while (!ali.isEmpty( )) + item3); iteml = ali.top( ) ; ali.pop () ; System.out.println (item1) ;arrow_forward
- You are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forwardThis is in c++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. Don't print the dummy head node. Example input : 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 output: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forwardJava Program ASAP Please modify Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.HashMap;import java.util.Map;import java.util.Scanner;public class MorseCodeConverter { public static void main(String[] args) { Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text =…arrow_forward
- the number of edges in a complete 2 of 2 undirected graph of 80 vertices is.? Select one: a. exactly equals 3160 b. None c. less than or equals 3160 d. less than or equals 6320 e. exactly equals 6320 Assume that you have a doubly link list. Pointer x is pointing to the last node in the link list and pointer y is pointing to before the last node. which of the following will remove the node pointed by x from the list? Select one: O a. delete x; b. y->prev->next = y->next; X->prev= y->prev; delete x; O C. X->prev->next = x->next; y->prev= x->prev; delete x; O d. x->next = y; y->prev = x->prev; delete x;arrow_forwardIn this problem you will implement a function called triangle_countwhich will take as input a graph object G, representing an undirectedgraph G, and will return the number of triangles in G. Do not use anyimports for this problem. To simplify the problem you may assume thateach edge is stored twice in G. That is if an edge goes from u to v then vwill be in u’s collection and u will be in v’s collection. If you would prefer,you may assume that an edge is only stored once. In either case G willneed to be regarded as undirected.arrow_forwardThe Task This assignment requires you to write a non-verbose input-driven java program for m aint aining a binary search tree (BST) of integer elements (negative, zero or positive). The speciality of your BST will be that it will record the frequency of occurrence for each integer element in it. Actions provisioned on the BST. When your program is running, a user should be able to select one of the following actions to be performed on the BST. 1. Insert an element into the BST 2. Sear ch for an element in the BST 3. Find the maximum element from the BST 4. Find the minimum element from the BST 5. Print the elements in the BST in preorder 6. Print the elements in the BST in postorder 7. Print the elements in the BST in inorder 8. Delete an element Anything else to exit the program When the progr am is executed, the user will provide their choice of action on the BST, by entering one of the numbers between 1 to 8 that corre spond to their choice. For example, if the user wishes to insert…arrow_forward
- Write a program in c++ and make sure it works, that reads a list of students (first names only) from a file. It is possible for the names to be in unsorted order in the file but they have to be placed in sorted order within the linked list.The program should use a doubly linked list.Each node in the doubly linked list should have the student’s name, a pointer to the next student, and a pointer to the previous student. Here is a sample visual. The head points to the beginning of the list. The tail points to the end of the list. When inserting consider all the following conditions:if(!head){ //no other nodes}else if (strcmp(data, head->name)<0){ //smaller than head}else if (strcmp(data, tail->name)>0){ //larger than tail}else{ //somewhere in the middle} When deleting a student consider all the following conditions:student may be at the head, the tail or in the middleBelow, you will find a sample of what the file looks like. Notice the names are in…arrow_forwardJava programming homework please helparrow_forwardPlease use the alphabet shown in the screenshot below to code a Depth-first search (DFS) algorithm in C++.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
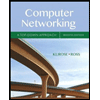
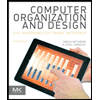
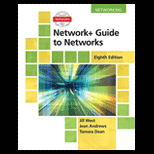
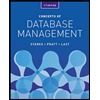
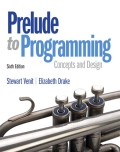
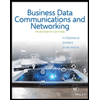