Given a ListItem class, complete main() using the built-in list type to create a linked list called shoppingList. The program should read items from input (ending with -1), adding each item to shoppingList, and output each item in shoppingList using the PrintNodeData() function. Ex. If the input is: milk bread eggs waffles cereal -1 the output is: milk bread eggs waffles cereal #include "ListItem.h" #include #include #include using namespace std; int main (int argc, char* argv[]) { // TODO: Declare a list called shoppingList of type ListItem string item; // TODO: Read inputs (items) and add them to the shoppingList list // Read inputs until a -1 is input // TODO: Print the shoppingList list using the PrintNodeData() function return 0; } #ifndef LISTITEMH #define LISTITEMH #include using namespace std; class ListItem { public: ListItem(); ListItem(string itemInit); // Print this node void PrintNodeData(); private: string item; }; #endif #include "ListItem.h" #include ListItem::ListItem() { item = ""; } ListItem::ListItem(string itemInit) { item = itemInit; } // Print this node void ListItem::PrintNodeData() { cout << item << endl; }
Given a ListItem class, complete main() using the built-in list type to create a linked list called shoppingList. The program should read items from input (ending with -1), adding each item to shoppingList, and output each item in shoppingList using the PrintNodeData() function.
Ex. If the input is:
milk
bread
eggs
waffles
cereal
-1
the output is:
milk
bread
eggs
waffles
cereal
#include "ListItem.h"
#include <string>
#include <list>
#include <iostream>
using namespace std;
int main (int argc, char* argv[]) {
// TODO: Declare a list called shoppingList of type ListItem
string item;
// TODO: Read inputs (items) and add them to the shoppingList list
// Read inputs until a -1 is input
// TODO: Print the shoppingList list using the PrintNodeData() function
return 0;
}
#ifndef LISTITEMH
#define LISTITEMH
#include <string>
using namespace std;
class ListItem {
public:
ListItem();
ListItem(string itemInit);
// Print this node
void PrintNodeData();
private:
string item;
};
#endif
#include "ListItem.h"
#include <iostream>
ListItem::ListItem() {
item = "";
}
ListItem::ListItem(string itemInit) {
item = itemInit;
}
// Print this node
void ListItem::PrintNodeData() {
cout << item << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

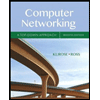
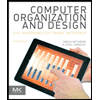
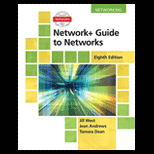
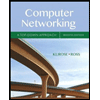
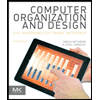
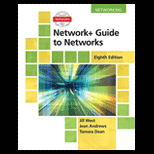
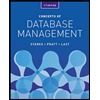
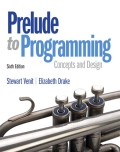
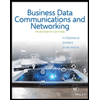