Your program should ask for the input from the user. The input ends with 999 to indicate that there are no more classes to be scheduled. Please enter class details. 3203 100 1500 1103 100 1500 2201 300 800 2203 100 1500 3401 200 800 1201 100 1500 999 The output should be the following: 3401 starts at 800 ends at 1000 2201 starts at 1000 ends at 1300 3203 starts at 1500 ends at 1600 2203 starts at 1600 ends at 1700 1201 starts at 1700 ends at 1800 1103 starts at 1800 ends at 1900 To help you get started, you are given with a code snippet to write your code. #include using namespace std; struct Course { int ccode; // course code int duration; // class duration in military hours, so 2 hours is 200 an so on int lecture_arrive_time; //prefered start time 800 for 8am and 1200 for 12pm }; void print_sched(Course c[],int); void class_sort(Course c[],int); int main() { int input=0,i=0; Course classes[100]; cout << "Please enter class details:" << endl; cin >> input; while (input != 999){ classes[i].ccode = input; cin >> classes[i].duration; cin >> classes[i].lecture_arrive_time; i++; cin >> input; } class_sort(classes, i); print_sched(classes, i); }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Your program should ask for the input from the user. The input ends with 999 to indicate that there are no more classes to be scheduled.
Please enter class details.
3203 100 1500
1103 100 1500
2201 300 800
2203 100 1500
3401 200 800
1201 100 1500
999
The output should be the following:
3401 starts at 800 ends at 1000
2201 starts at 1000 ends at 1300
3203 starts at 1500 ends at 1600
2203 starts at 1600 ends at 1700
1201 starts at 1700 ends at 1800
1103 starts at 1800 ends at 1900
To help you get started, you are given with a code snippet to write your code.
#include <iostream>
using namespace std;
struct Course
{
int ccode; // course code
int duration; // class duration in military hours, so 2 hours is 200 an so on
int lecture_arrive_time; //prefered start time 800 for 8am and 1200 for 12pm
};
void print_sched(Course c[],int);
void class_sort(Course c[],int);
int main()
{
int input=0,i=0;
Course classes[100];
cout << "Please enter class details:" << endl;
cin >> input;
while (input != 999){
classes[i].ccode = input;
cin >> classes[i].duration;
cin >> classes[i].lecture_arrive_time;
i++;
cin >> input;
}
class_sort(classes, i);
print_sched(classes, i);
}
** you will need to implement the functions **

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

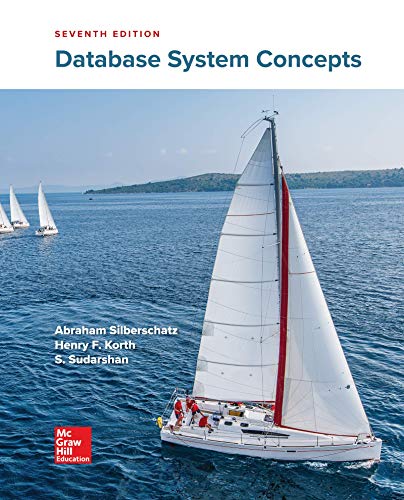
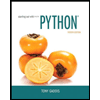
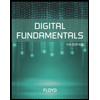
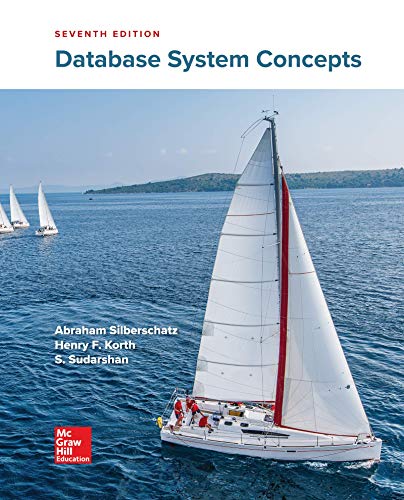
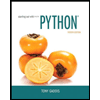
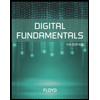
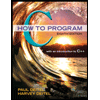
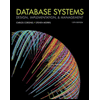
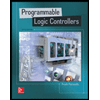