ISSUE NEEDED TO BE SOLVED: I attached the error codes that keep presenting themselves with the code I created and do not know what they mean or how to fix them to get the program to run.
ASSIGNMENT: You are working for a lumber company, and your employer would like a program that calculates the cost of lumber for an order. The company sells pine, fir, cedar, maple, and oak lumber. Lumber is priced by board feet. One board foot equals one square foot that is one inch thick. The price per board foot is given in the following table:
Pine 0.89
Fir 1.09
Cedar 2.26
Maple 4.50
Oak 3.10
The lumber is sold in different dimensions (specified in inches of width and height, and feet of
length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). An entry from the user will be in the form of a letter and four
integer numbers. The integers are the number of pieces, width, height, and length. The letter will be one of P, F, C, M, O (corresponding to the five kinds of wood) or T, meaning total. When the letter is T, there are no integers following it on the line. The program should print out the price
for each entry, and print the total after T is entered. Here is an example run:
Enter item: P 10 2 4 8
10 2x4x8 Pine, Cost:$47.47
Enter item: M 1 1 12 8
1 1x12x8 Maple, cost:$36.00
Develop the program using functional decomposition, and use proper style and documentation in your code. Your program must make appropriate use of value-returning functions in solving this problem. Make sure that the user prompts are clear and that the output is labeled appropriately.
Code Created:
//<File name> -- brief statement as to the file’s purpose
//CSIS 111-<Section Number> ADD YOUR SECTION NUMBER
//<Sources if necessary>
//Include statements
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
//function prototypes
float calculateFeet(int w, int h, int l);
//Global declarations: Constants and type definitions only -- no variables
int main()
{
//In cout statement below SUBSTITUTE your name and lab number
cout << "Your name -- Lab Number" << endl << endl;
//Variable declarations
char ch;
float total = 0, cost = 0;
cout << setprecision(2) << fixed;
while (true)
{
cout << "Enter item: ";
cin >> ch;
if (ch != 'T')
{
int piece, width, height, length;
cin >> piece;
cin >> width;
cin >> height;
cin >> length;
switch (ch)
{
case'P':
cost = (0.89 * piece * calculateFeet(width, height, length));
cout << piece << "" << width << "x" << height << "x" << length << "" << "Pine," << " cost: " << "$" << cost << "\n";
break;
case 'F':
cost = (1.09 * piece * calculateFeet(width, height, length));
cout << piece << "" << width << "x" << height << "x" << length << "" << "Fir," << " cost: " << "$" << cost << "\n";
break;
case 'C':
cost = (2.26 * piece * calculateFeet(width, height, length));
cout << piece << "" << width << "x" << height << "x" << length << "" << "Cedar," << " cost: " << "$" << cost << "\n";
break;
case 'M':
cost = (4.50 * piece * calculateFeet(width, height, length));
cout << piece << "" << width << "x" << height << "x" << length << "" << "Maple," << " cost: " << "$" << cost << "\n";
break;
case 'O':
cost = (3.10 * piece * calculateFeet(width, height, length));
cout << piece << "" << width << "x" << height << "x" << length << "" << "Oak," << " cost: " << "$" << cost << "\n";
break;
}
total += cost;
}
else
{
cout << "Total cost: $" << total << "\n";
break;
}
}
//Program logic
//Closing program statements
system("pause");
return 0;
}
//Function definitions
float calculateFeet(int w, int h, int l)
{
float feet = ((w*h*l)/12.0);
return feet;
}
ISSUE NEEDED TO BE SOLVED: I attached the error codes that keep presenting themselves with the code I created and do not know what they mean or how to fix them to get the program to run. My assignment is due tonight and am desparate for help. The program is through Visual Studio and is C++ related


Step by step
Solved in 2 steps with 1 images

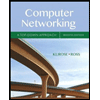
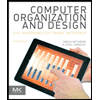
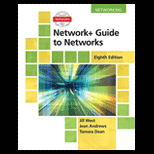
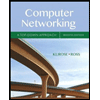
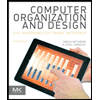
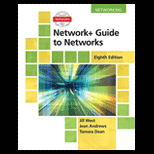
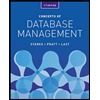
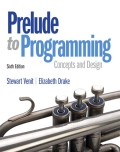
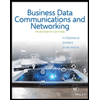