Your cousin owns an automotive maintenance shop that performs routine maintenance on cars. He has asked you to write a program that will provide a list of services (and their respective prices) to users and allow them to choose any, or all, services they would like and display the final price, including a 28% labor charge, a 9% markup if the car is an import, and an 8% sales tax. Task To accomplish the above, do the following: Write the two methods outlined below. Test your program and screenshot your successful test. carMaintenance method This method should accept the make of the car as a parameter (e.g., BMW, Ford, Ferrari, etc.). It should display the services and their prices as follows: Services: Oil Change, Tune up, Brake Job, Transmission Service, respectively: 59.99, 111.99, 189.99, 149.99 These should be stored in parallel arrays. Ask the user to select a service from the list presented for his . Allow the user to request as many services as he wants. Use an accumulator to total the price for all services requested. This method should return the total price to main. Do not call the calcFinalPrice method inside of this method – the point here is for you to write a method that returns a value. calcFinalPrice method This method should accept, as a parameter, the total price that was passed to main from the carMaintenance method and whether or not the car is an import. This method should add 28% to the price for labor. Then, take on 9% if the car is an import. Then an 8% sales tax. Finally, the method should output the final price. Do not return the final price here, simply print from this method. To test your methods in main, Ask the user for the appropriate information needed to pass to your methods (hint: you will only need to ask for 2 things). Invoke (call) each method, passing the appropriate information. Hints Before invoking (calling) your methods consider asking the user for both the make and if the car is an import. Use an if statement to turn the yes or no response into a boolean. Adding 28% to the price, then 8% is not the same as adding 36%. For example, if the cost is $100, 28% + 8% is $138.24. At 36%, this price is $136.00. For your output screenshot, also include the following scenario: an Oil Change, Tune up, and Brake Job for an import would be 361.97 before taxes and labor and 545.42 after. Deliverables Submit files containing the following: 1) Java source file (.java file) 2) Screenshots of successful test run. 3) Screenshots of successful run including Tune Up, Brake Job, and Oil Change.
Please create a code that follows the directions below exactly, and have the output look just like the one in the picture provided. If possible, please refrain from using switch statements in the code.
Insturctions:
This assignment will assess your understanding of void and value returning static methods.
Your cousin owns an automotive maintenance shop that performs routine maintenance on cars. He has asked you to write a program that will provide a list of services (and their respective prices) to users and allow them to choose any, or all, services they would like and display the final price, including a 28% labor charge, a 9% markup if the car is an import, and an 8% sales tax.
Task
To accomplish the above, do the following:
- Write the two methods outlined below.
- Test your program and screenshot your successful test.
carMaintenance method
This method should accept the make of the car as a parameter (e.g., BMW, Ford, Ferrari, etc.). It should display the services and their prices as follows:
Services: Oil Change, Tune up, Brake Job, Transmission Service, respectively: 59.99, 111.99, 189.99, 149.99
These should be stored in parallel arrays. Ask the user to select a service from the list presented for his . Allow the user to request as many services as he wants. Use an accumulator to total the price for all services requested. This method should return the total price to main. Do not call the calcFinalPrice method inside of this method – the point here is for you to write a method that returns a value.
calcFinalPrice method
This method should accept, as a parameter, the total price that was passed to main from the carMaintenance method and whether or not the car is an import. This method should add 28% to the price for labor. Then, take on 9% if the car is an import. Then an 8% sales tax. Finally, the method should output the final price. Do not return the final price here, simply print from this method.
To test your methods in main, Ask the user for the appropriate information needed to pass to your methods (hint: you will only need to ask for 2 things). Invoke (call) each method, passing the appropriate information.
Hints
- Before invoking (calling) your methods consider asking the user for both the make and if the car is an import. Use an if statement to turn the yes or no response into a boolean.
- Adding 28% to the price, then 8% is not the same as adding 36%. For example, if the cost is $100, 28% + 8% is $138.24. At 36%, this price is $136.00.
- For your output screenshot, also include the following scenario: an Oil Change, Tune up, and Brake Job for an import would be 361.97 before taxes and labor and 545.42 after. Deliverables Submit files containing the following: 1) Java source file (.java file) 2) Screenshots of successful test run. 3) Screenshots of successful run including Tune Up, Brake Job, and Oil Change.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

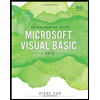
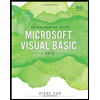