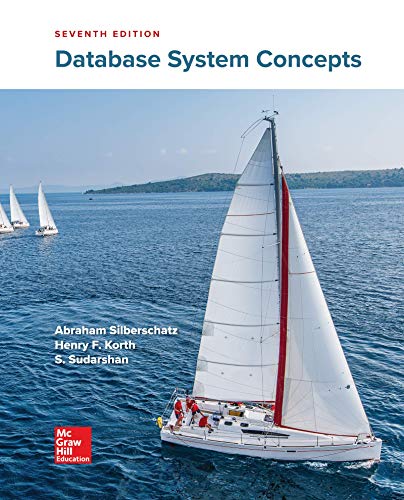
Concept explainers
Please check images for the rest of the question and provide code with comments and explanations.
Thank you.
Write a set of static methods, to make console input simpler.
Consider that the only console input method using the Scanner class is inputString(), shown below; include it in your solution. This means you are not permitted to use the Scanner class in any of your methods.
public static String inputString ()
{
Scanner scan = new Scanner (System.in); // attach to console
return ( scan.next() ); // return next string from input console stream
}
Example of calling and testing this method in your main():
String str = inputString(); // get input from console, assign to str
displayln ("Input A is: " + str); // display input string
displayln ("Input B is: " + inputString() ); // get input and display
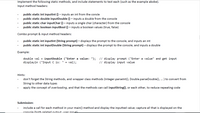

Step by stepSolved in 3 steps with 1 images

- The next sections will provide you with the definitions of the classes MonthCalender and DateTimerPicker respectively.arrow_forwardI need help writing a program using the DrawingPanel that contains a method for drawing a checkerboard. The checkerboard should be 160 units wide (and tall) and should have an input parameters that allow the method to place the checkerboard at any x and y coordinates. For example you should be able to do the following in your main program.drawCheckerboard(100,100, myG);drawCheckerboard(120,500, myG);To draw two different checkerboards.You may choose your own colors for the squares on the checkerboard.arrow_forwardThe first version of Abby’s Eggs worked but was a bit cumbersome. I.e. you couldn’t edit a single parameter, you had to always re-enter all of them. Store the parameters (number of chickens, eggs laid per day, etc.,) in static fields of the class. Have separate methods for updating the parameters. Use a switch statement to take menu selections for updating parameters or outputting results. If the menu choice is 0, the program should terminate, invalid inputs should be ignored and any prompt repeated. Before displaying updated menu, “clear the screen” I got most of the code working, but I can't find a way to clear the sceen before displaying the updated menu. I tried using "System.out.print("\033[H\033[2J"); System.out.flush();", but it is not working. I am using the Apache NetBeans IDE.arrow_forward
- Write a Rectangle class. Its constructor should accept length and width as a parameter. It should have methods that return its length, width, perimeter, and area. (Use Java) Show a demonstration where you create two separate rectangles.arrow_forwardWhat error message do you see in the Code Pad if you type the following? NumberDisplay.getValue() Take a careful look at this error message and try to remember it because you will likely encounter something similar on numerous future occasions. Note that the reason for the error is that the class name, NumberDisplay, has been used incorrectly to try to call the getValue method, rather than the variable name nd.arrow_forwardIs it possible to put a temporary stop to the progression of a single operation while it is being carried out? Your comments should always be supported by logical arguments, no matter what kind they are?arrow_forward
- Override the testOverriding() method in the Triangle class. Make it print “This is theoverridden testOverriding() method”. (add it to triangle class don't make a new code based on testOverriding in SimpleGeometricObject class.) (this was the step before this one) Add a void testOverriding() method to the SimpleGeometricObject class. Make it print“This is the testOverriding() method of the SimpleGeometricObject class”arrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forwardHi, I need help with Java code. Please see attached screenshot. Thank you! Input isarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
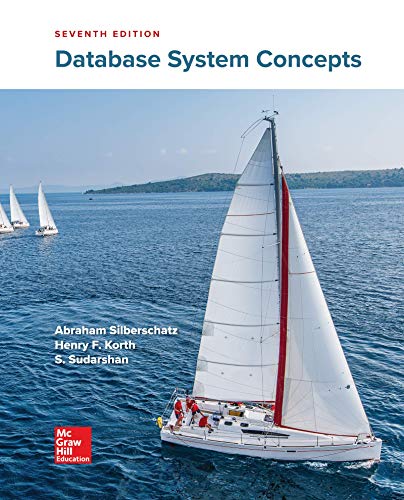
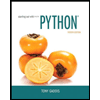
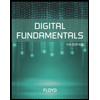
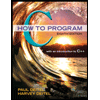
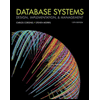
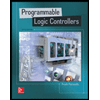