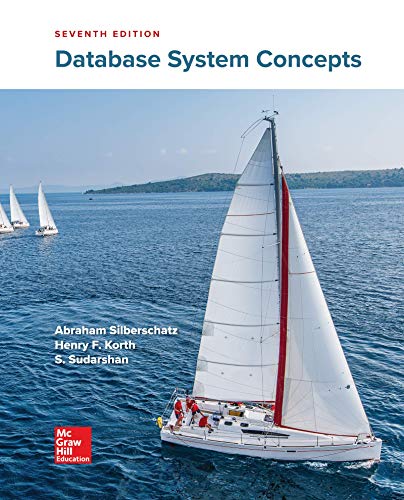
In this lab, you will write a MIPS
You may need to learn a few syscall parameters that deal with character and single precision floating point numbers. You will find those in the help section of Mars. We use mov.s to copy the value of one single precision floating point register to another.
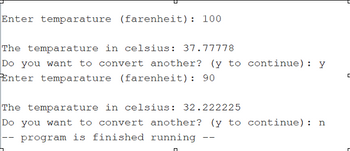

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- in C program For this assignment, you will need to build a program that does the following: The process of finding the largest value is used frequently in computer applications. For example, a program that determines the winner of a sales competition would input the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a program that has the user input a series of 10 integers and determine and print the largest integer. Your program should use at least the following three variables: counter: A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed). number: The integer most recently input by the user. largest: The largest number found so far. You must use a while loop as well as an if statement. Properly give the user instructions, take input and print the largest number before saying goodbye to the user. The program output will look something like…arrow_forwardI need help on this?arrow_forwardYou are tasked with writing a program to process students’ end of semester marks. For each student you must enter into your program the student’s id number (an integer), followed by their mark (an integer out of 100) in 4 courses. A student number of 0 indicates the end of the data. A student moves on to the next semester if the average of their 4 courses is at least 60 (greater than or equal to 60). Write a program to read the data and print, for each student: The student number, the marks obtained in each course, final mark of the student (average of all the marks), their final grade (A,B,C,D or F) and whether or not they move on to the next semester. In addition, after all data entry is completed, print The number of students who move on to the next semester and the number who do not. The student with the highest final mark (ignore the possibility of a tie). Grades are calculated as follows: A = 70 and over, B = 60 (inclusive) -70, C = 50(inclusive)-60 D =…arrow_forward
- Design the logic (flowchart and/or pseudocode) using arrays for a program. It asks a user to enter a product number, then searches through an existing list of product numbers, for each one of which a recall has been issued recently. Based on the search result, a message will be displayed to the user (e.g., "A recall has been issued for this product." or "No recall found for this product."). Here is the existing list of product numbers: 100, 103, 108, 110, and 125. PreviousNextarrow_forwardC level program 1. Write a program that takes an integer n as input (from Keyboard) and compute and display (a) The sum of digits. (b) The integer with digits reversed, and multiplied by 3. For example, if the input is 4318, then in part (a), you'll display integer 4318, and sum of digits 4 + 3 + 1 + 8 = 16; and in part (b), you'll compute and display 8134 and also 24402, which is 4318 * 3. (c) Test your program with 3 integers: 4318, 401, and an integer of 5 digits of your choice. Show and explain source code and show outputarrow_forwardA bit confused on where to begin this program. I'm confused by the information I'm being given I think? Honestly I'm not sure. I need to write the program in pseudocode."A dramatic theater has three seating sections. It charges section A seats 20$ each, section B seats 15$ each, and section C seats 10$ each. There are 300 seats in section A, 500 seats in section B, and 200 seats in section C. Design a program that asks for the number of tickets sold in each section, then display the amount of income generated from ticket sales. The program should validate the numbers from each section."I think I know how to finish the program but I'm not sure where I should start. I don't know what variables to declare and I don't know if I need to declare ALL the variables, if any, before the "While" loop.arrow_forward
- using loops in C++ Write a program that will predict the size of a population of organisms. The program should ask the user for the starting number of organisms, their average daily population increase (as a percentage, expressed as a fraction in decimal form: for example 0.052 would mean a 5.2% increase each day), and the number of days they will multiply. A loop should display the size of the population for each day.Prompts, Output Labels and Messages .The three input data should be prompted for with the following prompts: "Enter the starting number of organisms: ", "Enter the average daily population increase (as a percentage): , and "Enter the number of days they will multiply: " respectively. The population sizes displayed should appear on separate lines, each of the form "On day D the population size was P." where D is the day number (starting with 1) and P is the population you calculated for that day.Input Validation.Do not accept a number less than 2 for the starting size of…arrow_forwardprogram must include a processing loop that allows multiple sets of data to be processed and the program should be terminated when there is no more data to process Develop a program in python that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 Run the program with the following values: Start Stop Multiplier 2 10 4 4 12 6arrow_forwardThis program in C++ obtains a series of letter grades as input from a user. The program should calculate and output the total grade points and the grade point average (note: you may have to track the total number of inputs and the grade points to calculate the grade point average). The algorithm should execute 3 times for testing purposes.Use the appropriate loop for the main structure of the program that will stop accepting letter grades when the user inputs X. Also, choose the appropriate selection statement to convert the letter grade to the correct grade points.Additional Requirements:• Use appropriate data types and variable names throughout the code.• Use constants appropriately. Output needs to look like the image below.arrow_forward
- I am creating a c++ snakes and ladders game (no visuals). I want the game to have up to 5 or 6 players. For the snakes/ladders I am going to create a function which needs the dice roll and adds it to the score which will then decide if the player has landed on a snake or a ladder, is there any way I can get all players scores to be stored in the “score” variable in the switch statements without using multiple switch statements? So I basically want a switch statement which can accommodate all 1-6 players . E.g p1 initially at 1 rolls a 4. So their new score is 1+4=5, then the switch function checks for a ladder or snake. Then player 2 who's initially a 2 rolls a 5, so their new score is 2+5=7 then THE SAME switch function checks for a snake or ladder... And so on for all other players.arrow_forwardA place to buy candy is from a candy machine. A new candy machine is bought for the gym, but it is not working properly. The candy machine has four dispensers to hold and release items sold by the candy machine and a cash register. The machine sells four products — candies, chips, gum, and cookies—each of which is stored in a separate dispenser. You have been asked to write a program for this candy machine so that it can be put into operation. The program should do the following: Show the customer the different products sold by the candy machine Let the customer make the selection Show the customer the cost of the item selected Accept money from the customer Return change Release the item, that is, make the sale Note: java programming, please used given outline code //Implement the blueprint of the Candy Machine import java.util.*; public class CandyMachine { // Each candy machine is made of 1 CashRegister and 4 Dispensers CashRegistercashRegister; Dispensercandy;…arrow_forwardWrite a console program, that will calculate the pocket money saved over a period, with one cent at the start of account setup, will double every day. For example, if the opening balance is one cent the first day, it will be two cents the second day, and continues to double each day. The console program you will write, should ask the user for the number of days, and display a table showing what the saving was for each day, and then show the total savings at the end of the period. The output should be displayed in a dollar amount, not the number of cents.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
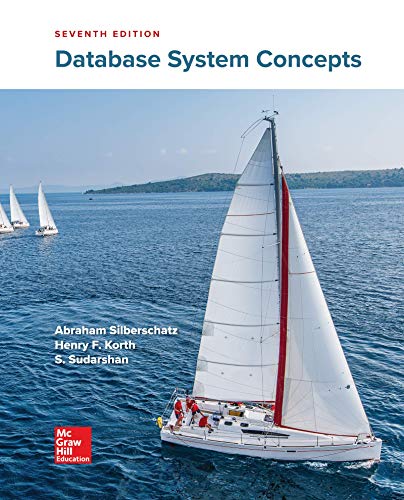
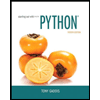
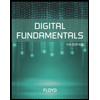
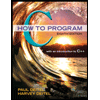
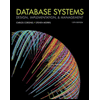
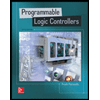