in C program For this assignment, you will need to build a program that does the following: The process of finding the largest value is used frequently in computer applications. For example, a program that determines the winner of a sales competition would input the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a program that has the user input a series of 10 integers and determine and print the largest integer. Your program should use at least the following three variables: counter: A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed). number: The integer most recently input by the user. largest: The largest number found so far. You must use a while loop as well as an if statement. Properly give the user instructions, take input and print the largest number before saying goodbye to the user.
in C program
For this assignment, you will need to build a program that does the following:
The process of finding the largest value is used frequently in computer applications. For example, a program that determines the winner of a sales competition would input the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a program that has the user input a series of 10 integers and determine and print the largest integer. Your program should use at least the following three variables:
counter: A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed).
number: The integer most recently input by the user.
largest: The largest number found so far.
You must use a while loop as well as an if statement.
Properly give the user instructions, take input and print the largest number before saying goodbye to the user.
The program output will look something like this:
Please enter 10 integers and I will find the largest for you.
12
200
52
63
85
99
41
400
10
0
The largest number is: 400
Have a great day.

Step by step
Solved in 3 steps with 1 images

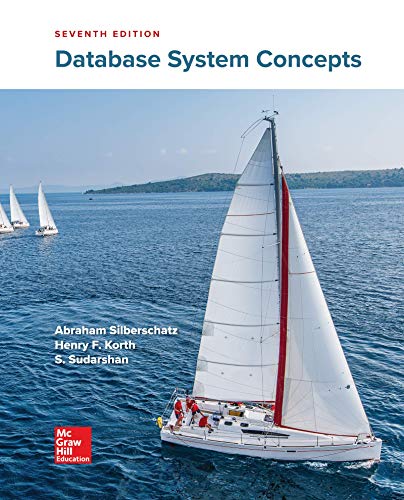
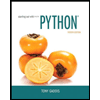
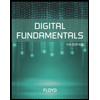
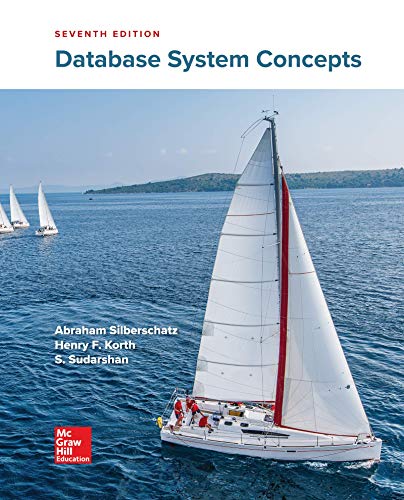
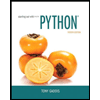
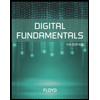
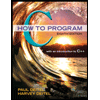
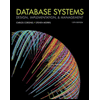
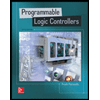