You should draw the hierarchy for your classes to understand how the coding should be structured. Be sure to notate any classes that are abstract. Write an abstract class for Shape. The intended subclasses are Polygon, Circle, Triangle, and Rectangle. All of these will have a name, an area, and a perimeter. (Consider which methods might be abstract.) Add a toString method that will return the information about the Shape, for example: Triangle, Area: 7.5, Perimeter: 12.0 Write the class Rectangle. A Rectangle is a Shape and should have a width and a height. When a Rectangle is outputted, it should read: Rectangle, Length: #, Width: #, Area: #, Perimeter: # Write the class for Circle. Every circle is a Shape that has a radius. Be sure to provide an accessor method (getter) for the radius. When a circle is outputted, it should read: Circle, Radius: #, Area: #, Circumference: # Write a TestShape class that has the main method, which creates Rectangle and Circle objects. Print out the specific attributes about the shape.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In-class Assignment Day 8
You should draw the hierarchy for your classes to understand how the coding should be structured. Be sure to notate any classes that are abstract.
- Write an abstract class for Shape. The intended subclasses are Polygon, Circle, Triangle, and Rectangle. All of these will have a name, an area, and a perimeter.
(Consider which methods might be abstract.) Add a toString method that will return the information about the Shape, for example: Triangle, Area: 7.5, Perimeter: 12.0
- Write the class Rectangle. A Rectangle is a Shape and should have a width and a height.
When a Rectangle is outputted, it should read: Rectangle, Length: #, Width: #, Area: #, Perimeter: #
- Write the class for Circle. Every circle is a Shape that has a radius. Be sure to provide an accessor method (getter) for the radius.
When a circle is outputted, it should read: Circle, Radius: #, Area: #, Circumference: #
- Write a TestShape class that has the main method, which creates Rectangle and Circle objects. Print out the specific attributes about the shape.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

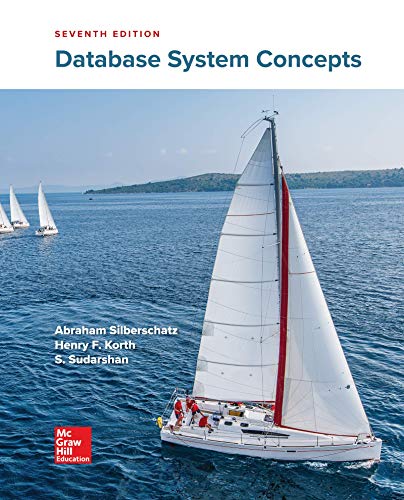
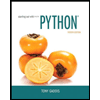
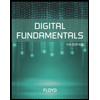
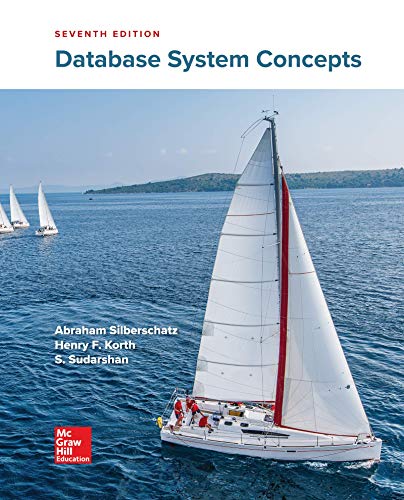
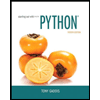
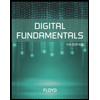
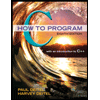
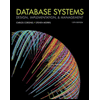
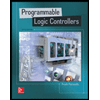