Lab Goal : This lab was designed to teach you more object oriented programming and start you down the path of creating larger programs and games like BlackJack and Elevens [ one of the new AP CS A Labs ]. You will create a Deck class that contains a List < Card > Lab Description : You need to design a class that contains an instance variables which is a List < Card > and an int that keeps track of the top card position . You then need to make a constructor and related methods. You will need a dealCard method and a shuffle method. Use the template shown below. /make a Deck class public static final int NUMCARDS = 52; public static String[] SUITS = "CLUBS HEARTS DIAMONDS SPADES".split(" "); private List cards; private int top; //make a Deck constructor //refer cards to new ArrayList //set top to the top of the deck 51 //loop through all suits //loop through all faces 1 to 13 //add a new BlackJackCard to the deck //make a dealCard() method that returns the top card //write a shuffle() method //use Colletions.shuffle //reset the top card
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Lab Goal : This lab was designed to teach you more object oriented programming and start you
down the path of creating larger programs and games like BlackJack and Elevens [ one of the new AP CS A
Labs ]. You will create a Deck class that contains a List < Card >
Lab Description : You need to design a class that contains an instance variables which is a List < Card
> and an int that keeps track of the top card position . You then need to make a constructor and related
methods. You will need a dealCard method and a shuffle method. Use the template shown below.
/make a Deck class
public static final int NUMCARDS = 52;
public static String[] SUITS = "CLUBS HEARTS DIAMONDS SPADES".split(" ");
private List<Card> cards;
private int top;
//make a Deck constructor
//refer cards to new ArrayList
//set top to the top of the deck 51
//loop through all suits
//loop through all faces 1 to 13
//add a new BlackJackCard to the deck
//make a dealCard() method that returns the top card
//write a shuffle() method
//use Colletions.shuffle
//reset the top card

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

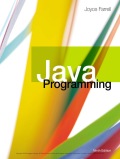
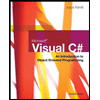
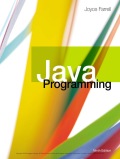
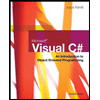