You have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as a two-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on. When the digger reaches row number n, it has to be air-lifted out of the mine, and is no longer usable. Every time the digger hits a square with resources in it, it collects those resources. We want to find out the maximum number of resources a digger can collect from this mine in one run. Let’s implement a recursive solution to this problem, reducing the problem of finding the maximum number of resources reachable from any point in the grid to finding the maximum number of resources that can be achieved by moving down-left, then finding the maximum number of resources by moving down-right, and selecting the highest of the two. Task 2.1 Recursive maxResources: Let’s write a method called maxResources. This method will take a two-dimensional integer array as input and produce one integer output. The input represents the grid map of the mine and the integer output should be equal to the maximum amount of resources that can be collected by a digger in a single run. We want to have a method that is implemented recursively, so it may be useful to implement a version with additional inputs to allow it to keep track of which cell it is looking at. The recursive formula should define maxResources for a particular coordinate (x,y) as the sum of the number of resources in (x,y) and the value o. Task 2.2 maxResourcesM: If you have implemented the maxResources method and tried testing it with large input sizes, you may have noticed that it starts to run very slowly after a certain depth. This is because the time complexity of the maxResources method is very large, close to O(2n ). Let’s improve this method by adding memoization to its implementation. Make a copy of the original maxResources method and call it maxResourcesM. Re-implement this method in a way that would allow it to store the results of previous calls and use those when the same calls are made again.
You have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as a two-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on. When the digger reaches row number n, it has to be air-lifted out of the mine, and is no longer usable. Every time the digger hits a square with resources in it, it collects those resources. We want to find out the maximum number of resources a digger can collect from this mine in one run. Let’s implement a recursive solution to this problem, reducing the problem of finding the maximum number of resources reachable from any point in the grid to finding the maximum number of resources that can be achieved by moving down-left, then finding the maximum number of resources by moving down-right, and selecting the highest of the two.
Task 2.1 Recursive maxResources:
Let’s write a method called maxResources. This method will take a two-dimensional integer array as input and produce one integer output. The input represents the grid map of the mine and the integer output should be equal to the maximum amount of resources that can be collected by a digger in a single run. We want to have a method that is implemented recursively, so it may be useful to implement a version with additional inputs to allow it to keep track of which cell it is looking at. The recursive formula should define maxResources for a particular coordinate (x,y) as the sum of the number of resources in (x,y) and the value o.
Task 2.2 maxResourcesM:
If you have implemented the maxResources method and tried testing it with large input sizes, you may have noticed that it starts to run very slowly after a certain depth. This is because the time complexity of the maxResources method is very large, close to O(2n ). Let’s improve this method by adding memoization to its implementation. Make a copy of the original maxResources method and call it maxResourcesM. Re-implement this method in a way that would allow it to store the results of previous calls and use those when the same calls are made again.

Step by step
Solved in 3 steps with 1 images

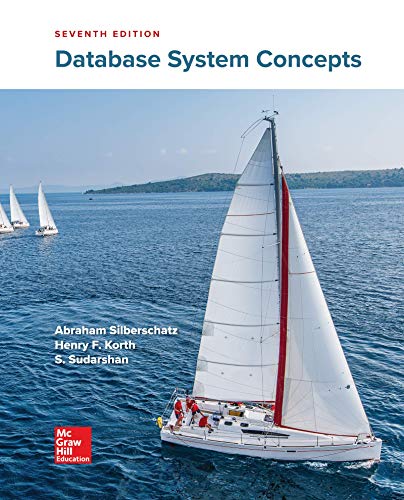
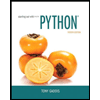
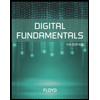
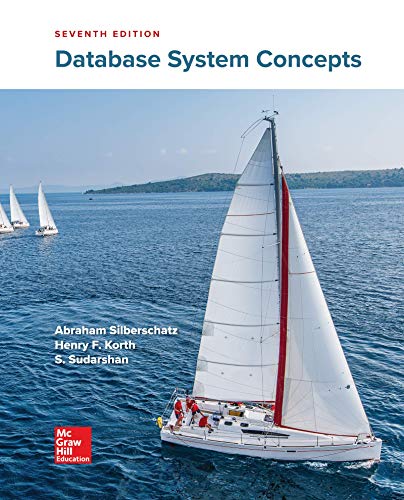
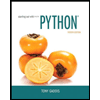
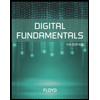
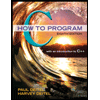
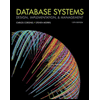
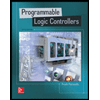