Write the magic number program in C++ so that the user can guess at more than one magic number where the magic number is an integer between 1 and 20. When the program is run, the user should be prompted if they would like to guess at a magic number and continue to be prompted as such after they have guessed a magic number correctly. A while loop is necessary for this capability, The user should be given as many guesses as necessary for each number until they guess the number correctly. A do-while loop must be implemented for this part of the program. They should also be responded with a clue after each guess on whether the guess is too high or low. The program should also make sure that if the user enters an invalid guess at the magic number (less than 1 or greater than 20), then the user should be prompted with an invalid guess message and not be penalized for a guess attempt.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Write the magic number program in C++ so that the user can guess at more than one magic number where the magic number is an integer between 1 and 20. When the program is run, the user should be prompted if they would like to guess at a magic number and continue to be prompted as such after they have guessed a magic number correctly. A while loop is necessary for this capability, The user should be given as many guesses as necessary for each number until they guess the number correctly.
A do-while loop must be implemented for this part of the program. They should also be responded with a clue after each guess on whether the guess is too high or low. The program should also make sure that if the user enters an invalid guess at the magic number (less than 1 or greater than 20), then the user should be prompted with an invalid guess message and not be penalized for a guess attempt. A while loop must be implemented for this part of the program. After the user guesses the number correctly, they should be graded on their effort. If they guess the number correctly within 3 guesses, rate them as "Excellent". If they guess the number correctly within 5 guesses, rate them as "Good". If they guess the number correctly within 7 guesses, rate them as "Average". If they guess the number correctly with more than 7 guesses, rate them as "Poor". Use either an if-else-if ladder or a switch block to rate the user.

Step by step
Solved in 3 steps with 1 images

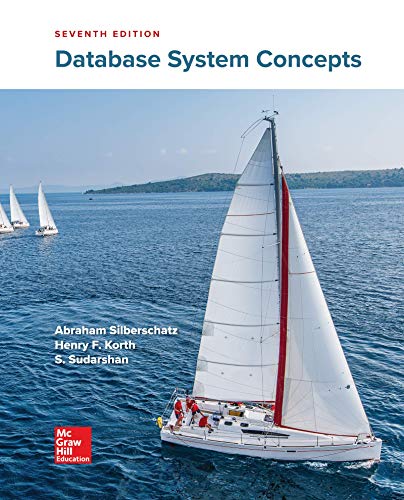
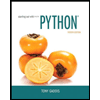
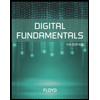
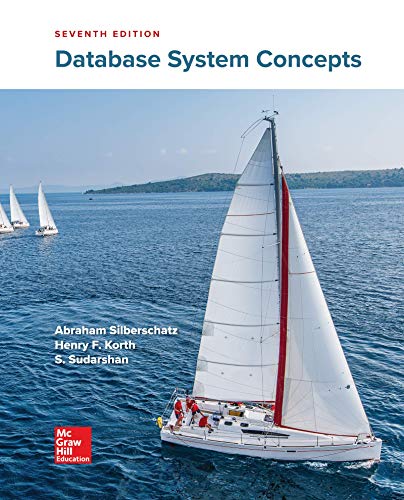
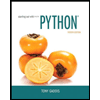
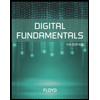
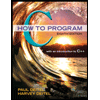
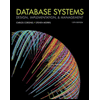
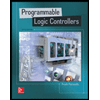