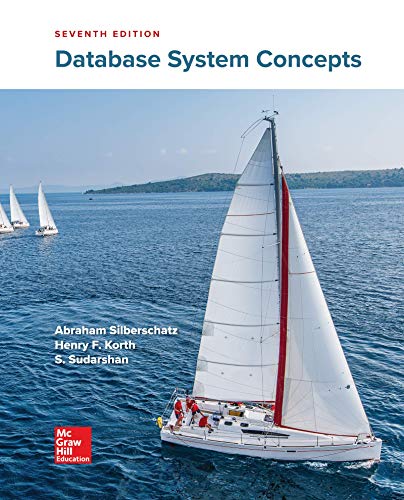
Concept explainers
make a C program that implements the "guess my number" game. The computer chooses a random number using the following random generator function
srand(time(NULL));
int r = rand() % 100 + 1;
that creates a random number between 1 and 100 and puts it in the variable r. (Note that you have to include <time.h>) Then it asks the user to make a guess. Each time the user makes a guess, the program tells the user if the entered number is larger or smaller than its number. The user then keeps guessing till he/she finds the number.
If the user doesn't find the number after 10 guesses, a proper game over message will be shown and the actual guess is revealed. If the user makes a correct guess in its allowed 10 guesses, then a proper message will be shown and the number of guesses the user made to get the correct answer is also printed.
After each correct guess or game over, the user decides to play again or quit and based on the user choice, the computer will make another guess and goes on or prints a proper goodbye message and quits A sample run is as follows (user inputs are in red):
Welcome to the Number Guess Game!
I choose a number between 1 and 100 and you have only 10 chanced to guess it!
OK, I made my mind!
What is your guess? > 5
My number is larger than 5!
9 guesses left.
What is your guess? > 75
My number is smaller than 75!
8 guesses left.
What is your guess? > 40
My number is smaller than 40!
7 guesses left.
What is your guess? > 23
My number is larger than 23!
6 guesses left.
What is your guess? > 30
My number is larger than 30!
5 guesses left.
What is your guess? > 35
You did it! My number is 35!
You found it with just 6 guesses.
Do you want to play again? (Y/N) > Y
OK, I made my mind!
What is your guess? > 15
My number is larger than 15!
9 guesses left.
...
And if the user makes 10 guesses without a success
...
What is your guess? > 405
Please enter a number between 1 and 100
What is your guess? > 45
My number is larger than 45!
1 guess left.
What is your guess? > 47
SORRY! You couldn't find it with 10 guesses!
My number was 46. Maybe next time!
Do you want to play again? (Y/N) > N
Thanks for playing! See you later.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Write a program that generates random numbers between -25 and 25 by using the randint function. The program will add up the numbers and terminate when the sum of the values becomes zero. The program should contain the following functions: add() : add up the values main(): the function where the program execution should begin and end. randomgen(): a function that returns a number between -25 and 25 A global variable subsetsum that keeps track of the sum The program should output the count of the number of values generated before exiting saying that the subset sum was zero.arrow_forwardDraw a flowchart for the Frazzle function.arrow_forwardThere are five errors in this code. Correct the errors and make the code work. Explain what are the errors found. import java.util.Scanner; //Testing.java //Displays running total of numbers in lines of standard //input correct to two decimal places. //Uses an out of range number (100) to quit. public class TWODPS{ public static void main (String [] args) { Scanner input new Scanner (System.in); double total=0; boolean flag=true; System.out.println ("Use an out of range entry 100 to quit."); while (flag) { System.out.println ("Enter a number on a line:"); double d = input.nextDouble (); if (outofRange (d) ) { flag=false; else{ dispTwoDPs ("The number value is: ",d); total = total + d; dispTwoDPs ("The total is: ",total); System.out.println (); System.out.println ("Next."); } //end of else }//end of while System.out.println ("You quit."); }//end of main static boolean outOfRange (double d) { if (d>-100) return true; if (d>100) return true; return false; }arrow_forward
- Write a program that paints the 4 areas inside the mxm square as follow: Upper left: black. RGB (0, 0, 0) Upper right: white. RGB (255, 255, 255) Lower left: red. RGB (255, 0, 0) Lower right: random colors Random colors Hint: Define a function that paints one of the four squares. Call the function 4 times to complete the painting. (painting means setting the pixels)arrow_forwardComputer Science please in java. must use while loop.arrow_forwardHelp Me With C Programming. At Lili's birthday party, there is a game arranged by Jojo as the host. The game is handing out a number of Y candies to a number of X people where all the sweets taste sweet except for the last one candy that tastes like rotten beans. The distribution of sweets will be sequential starting from position Z and if it passes the last position then the distribution of candy continues to the first position. Write down the person in the position of who will get the last candy. Format Input The first line of input is T, which is the number of test cases. The second row and the next number of T lines are X, Y, Z. X is the number of people who will be handed out candy. Y is the number of candies available. Z is the initial position of the person who will be handed out the candy. Format Output A string of "Case #N: " and a number that is the position of the person who got the last candy [Look at Image] In the sample above, for example, which is inputted in the…arrow_forward
- python3 Write a recursive function called is_palindrome(string) that takes a string parameter and checks if it is a palindrome ignoring the spaces, if any, and returns True/False. Sample output:>>> print(is_palindrome("never odd or even"))True>>> print(is_palindrome("step on no pets"))Truearrow_forwardWrite a Python program that will generate 1000 random numbers between 1 and 100 inclusive from the random generator. For each value returned from the random generator, keep a count of the number of even numbers generated and the number of odd numbers generated. Use the following functions within this program: - getRandom(): this function will call the random generator and return the generated integer value. - isOdd(): this function will return true or false depending on whether the number generated is odd or even. - update(): this function will update the counters for odd or even. - display(): once the program has completed 1000 random numbers, the counters for the number of odd and even will be displayed. REQUIREMENTS: - Add a beginning statement when the program starts to execute. - Add an ending statement when the program is complete. - Call the random generator 1000 times for a randomly generated number between…arrow_forward3) Suppose an airline company has hired you to write a program for choosing the passengers who should leave an overbooked flight. That means the airline has booked too many passengers hoping some of them would not show up on time, but passengers are on board and the aircraft doesn't have enough seats available for all these passengers. Your program chooses the passenger who must leave the flight. Passengers will be ranked based on the fare they have paid for booking (a double number called fare), and their number of loyalty points (an integer number called points). Fare and points are equally important, that means, in order to decide the position of each passenger in the list, fare and points each have 50% importance. A Person with minimum priority (combination of fare and points) will leave the flight. You need to define a class called Passenger with these member variables: passenger's name • fare points. Passenger class must have 3 accessors, 3 mutators, a constructor with three…arrow_forward
- Write a program that will:• Select a random number between 1 and 100.• Test if the number is prime• Call a function named isPrime(). Pass the number to be tested. isPrime() should test whetherthe number has any factors other than 1 and itself. isPrime() returns either True or False.• isPrime() should use a function isDivisible(x,y) to test if y divides evenly into x. That is,isDivisible() will identify whether y is a factor of x.• Print the resultarrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
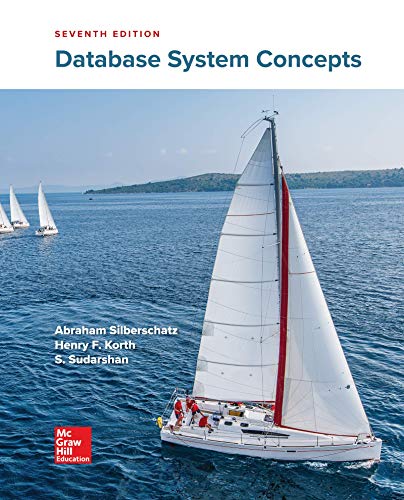
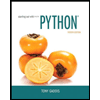
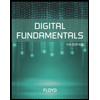
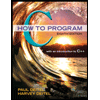
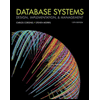
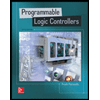