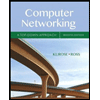
Concept explainers
Assignment 6 - More on Lists
Write pseudo-code not Python for problems requiring code. You are responsible for the
appropriate level of detail.
The questions in this assignment give you the opportunity to explore a new data structure
and to experiment with the hybrid implementation in Q3.
1. A deque (pronounced deck) is an ordered set of items from which items may be deleted at
either end and into which items may be inserted at either end. Call the two ends left and right.
This is an access-restricted structure since no insertions or deletions can happen other than at the
ends. Implement the deque as a doubly-linked list (not circular, no header). Write InsertLeft and
DeleteRight.
2. Implement a deque from problem 1 as a doubly-linked circular list with a header. Write
InsertRight and DeleteLeft.
3. Write a set of routines for implementing several stacks and queues within a single array.
Hint: Look at the lecture material on the hybrid implementation.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Can you please help me with this code because i am struggling on how to do this, this code has to be in C code.question that i need help with:Priority with round-robin schedules tasks in order of priority and uses round-robin scheduling for tasks with equal priority. There will be multiple queues in the system each representing one priority class. For ease of implementation, you need total 1 through max_priority numbers of queues. You should start scheduling job out of the max priority queue and serve the members of the queue following RR. The schedule of tasks has the form [task name] [priority] [CPU burst], with the following example format: T1, 4, 20 T2, 2, 25 T3, 3, 25 T4, 3, 15 T5, 10, 10 The output should look like this: Running task = [P1] [4] [5] for 5 units. Task P1 finished. Running task = [P5] [3] [4] for 4 units. Task P5 finished. Running task = [P4] [2] [7] for 1 units. Task P4 exhausted its Quantum hence will be Rescheduled. Running task = [P3] [2] [1] for 1 units. Task…arrow_forwardPlease answer the question in the screenshot. The language used is Java.arrow_forwardPlease answer the question in the screenshot. The language used here is in Java.arrow_forward
- The for construction is a loops that iteratively processes a given list. Consequently, it works so long even though there are objects to process. What about this claim: true or false?arrow_forwardThe specifications for the Sorted List ADT state that the item to bedeleted is in the list.1. Rewrite the specification for DeleteItem so that the listis unchanged if the item to be deleted is not in the list.2. Implement DeleteItem as specified in (a) using anarray-based implementation.3. Implement DeleteItem as specified in (a) using alinked implementation.4. Rewrite the specification for DeleteItem so that allcopies of the item to be deleted are removed if they exist.5. Implement DeleteItem as specified in (d) using anarray-based implementation.6. Implement DeleteItem as specified in (d) using alinked implementation.arrow_forwardUsing java, answer the following questions about the code below. 1) Create a new function or method that checks to see if an int parameter is present in the list. 2) Create a main() method or JUnit tests to show that your list code functions as intended. (Code) private class Nodeint data;Node next;Node(int data) {this.data = data;this.next = null;}}private Node first;private Node last; public void addToEnd(int data){ Node newNode = new Node(data);if (first == null){first = newNode;last = newNode;} else {last.next = newNode;last = newNode;}}public void printList(){ Node current = first;while (current != null){System.out.print(current.data + " ");current = current.next;}System.out.println();}public static void main(String[] args){node list = new node();list.addToEnd(2);list.addToEnd(4);list.addToEnd(6);list.printList();}}arrow_forward
- Please do this in C++. The only parts needed are commented as //TODO in MAIN. Please do not use cout, the PrintNodeData in MileageTrackerNode.cpp needs to be called. I am struggling with adding data into the linked list. Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new…arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not impact the overall…arrow_forward
- Java - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forward1. Create a UML using the UML Template attached. 2. In this lab, you will be creating a roulette wheel. The pockets are numbered from 0 to 36. The colors of the pockets are as follows: Pocket 0 is green. For pockets 1 through 10, the odd-numbered pockets are red and the even-numbered pockets are black. For pockets 11 through 18, the odd-numbered pockets are black and the even-numbered pockets are red. For pockets 19 through 28, the odd-numbered pockets are red and the even-numbered pockets are black. For pockets 29 through 36, the odd-numbered pockets are black and the even-numbered pockets are red. Create a class named RoulettePocket. The class's constructor should accept a pocket number. The class should have a method named getPocketColor that returns the pocket's color, as a string. Demonstrate the class in a program that asks the user to enter a pocket number, and displays whether the pocket is green, red, or black. The program should display an error message if the user…arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
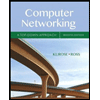
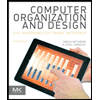
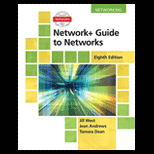
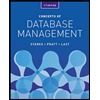
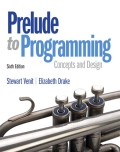
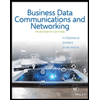