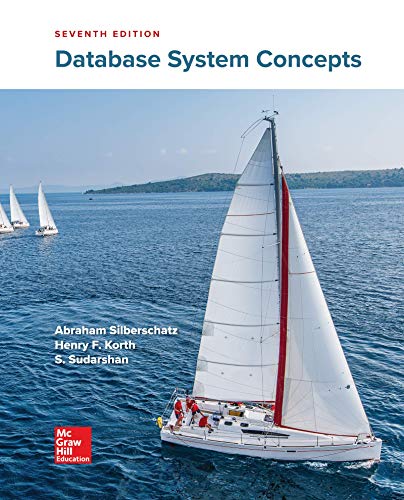
Concept explainers
Write in python and please include docstring. Write a class named Employee that has private data members for an employee's name, ID_number, salary, and email_address. It should have an init method that takes four values and uses them to initialize the data members. It should have get methods named get_name, get_ID_number, get_salary, and get_email_address.
Write a separate function (not part of the Employee class) named make_employee_dict that takes as parameters a list of names, a list of ID numbers, a list of salaries and a list of email addresses (which are all the same length). The function should take the first value of each list and use them to create an Employee object. It should do the same with the second value of each list, etc. to the end of the lists. As it creates these objects, it should add them to a dictionary, where the key is the ID number and the value for that key is the whole Employee object. The function should return the resulting dictionary.
For example, it could be used like this:
Remember in your testing that printing an object of a user-defined class will just print out the object's type and address in memory. If you want to print the values of its data members, you need to call its get functions, as shown in the print statement above.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- The hospital wants you to create software using your object-oriented concepts. It has nurses and doctors. You have to store information of all of these employees. Identify classes, their attributes and inheritance (if any) and implement following functionality: 1. Input function 2. Update salary of doctor 3. Update ward_no of nurse 4. Display functionarrow_forwardCreate pseudocode, flowchart and python code for the Patient Charges program. Thisfinal project requires multiple files (modules, drivers, and your main).Design a class named Patient that has fields for the following data:● First name, middle name, last name● Address, city, state, and ZIP code● Phone number● Name and phone number of emergency contactThe Patient class should have a constructor that accepts an argument for each field. ThePatient class should also have accessor and mutator methods for each field.Next, write a class named Procedure that represents a medical procedure that has beenperformed on a patient. The Procedure class should have fields for the following data:● Name of the procedure● Date of the procedure● Name of the practitioner who performed the procedure● Charges for the procedureThe Procedure class should have a constructor that accepts an argument for each field.The Procedure class should also have accessor and mutator methods for each field.Next, design a…arrow_forwardProgram Specifications Write a FancyCar class to support basic operations such as drive, add gas, honk horn and start engine. fancy_car.py is provided with function stubs. Follow each step to gradually complete all instance methods. Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main program includes basic calls to the instance methods. Add statements in the main program as instance methods are completed to support development mode testing. **Step 0: Complete the constructor to initialize the model and miles per gallon (MPG) with the values of the parameters. Initialize the odometer to five miles and the tank to be full of gas. By default, the model is initialized to "Old Clunker", and MPG is initialized to 24.0. Note the provided constant variable indicates the gas tank capacity of 14.0 gallons. Step 1 Complete the instance…arrow_forward
- Python Programming 3. Write an Employee class that keeps data attributes for the following pieces of information:(a). Employee name(b). Employee numberNext, write a class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information:(c). Shift number (an integer,such as1 for morning shift, 2 for evening shift)(d). Hourly payrateWrite the appropriate accessor and mutator methods for each class.arrow_forwardConvert the pseudocode into Python. 1. Pet ClassDesign a class named Pet, which should have the following fields:■ name: The name field holds the name of a pet.■ type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird".■ age: The age field holds the pet’s age.2. The Pet class should also have the following methods:■ setName: The setName method stores a value in the name field.■ setType: The setType method stores a value in the type field.■ setAge: The setAge method stores a value in the age field.■ getName: The getName method returns the value of the name field.■ getType: The getType method returns the value of the type field.■ getAge: The getAge method returns the value of the age field.3. Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the…arrow_forward82. Write a class definition for a class named Fraction that contains two integer fields named numerator and denominator. Include in the class a prototype for a method (function) named add that takes two Fraction objects as input arguments and returns a Fraction object as output. You do not need to provide an implementation of the add method.arrow_forward
- Farrell, Joyce. Microsoft Visual C#: An Introduction to Object-Oriented Programming (p. 479). Kindle Edition. 9. Write a program named SalespersonDemo that instantiates objects using classes named RealEstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each object’s data fields. First, create an abstract class named Salesperson. Fields include first and last names; the Salesperson constructor requires both these values. Include properties for the fields. Include a method that returns a string that holds the Salesperson’s full name—the first and last names separated by a space. Then perform the following tasks: • Create two child classes of Salesperson: RealEstateSalesperson and GirlScout. The RealEstateSalesperson class contains fields for total value sold in dollars and total commission earned (both of which are…arrow_forward# objected oriented programming in Python I need the solution of all below python question codes along with correct return. # 1. Implement a class named "MyClass"# This class should have:# * During instantiation of this class, the 'some_val' parameter should be set as# a variable with the same name in the instance# * Implement the method called 'plus_n' that takes one parameter (named 'n'), such that# it should return the sum of instance's 'some_val' variable and 'n'class MyClass: def __init__(self, some_val): # your code goes here instead of pass pass def plus_n(self, n): # your code goes here instead of pass pass ########################################################################################################################################################################################################################################## # 2. Given two classes 'Animal' and 'DigestiveSystem, implement, using COMPOSITION the method…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forward
- 5. CODE IN PYTHON Create a BankAccount class which has an __init__ method which accepts an account name and starting balance to store as instance fields. The class should also have a method to deposit money, a method to withdraw money, and a method called "get_balance" which returns a string of the format "{account_name} has a balance of {balance}" (do not print this out from within the class, just return the string). In the same file, create an instance of the BankAccount class with a hardcoded name and starting balance. Deposit any amount to the account and withdraw any amount from the account (both can be hardcoded) and then print the result of calling the get_balance method on the instance.arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forwardcreate a class in python with a constructor To create instances of the user defined class and practice applying them. Practice implementing loops. Part 1 Instructions:Create a class called BankAccount with the following variables: balancenameaccount_id Create 1 constructor for the class BankAccount (with or without parameters, your choice, but only 1): def __init_(self, name, account_id, balance)def __init_(self) Create the following methods in the BankAccount class: def viewAccountInfo() //prints all account information def withdraw(self, amount) //withdraws from the balance def deposit(self, amount) //deposits to the balance Your task after your class has been created: Create two objects, "personal" and "joint_account"with initial balances of:personal = $1000joint_account = $3000Use the constructor to create the objects. Create a program that prompts the user which account they would like to access personal or jointWhich account would you like to access? 1. Personal2. Joint…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
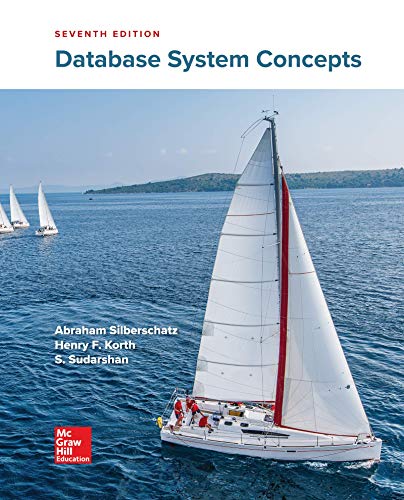
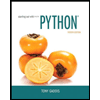
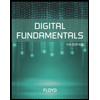
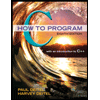
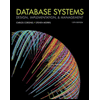
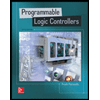