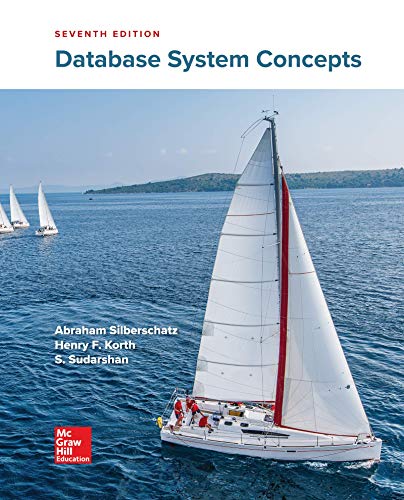
Concept explainers
Write an outline and logic for the following code:
EMPTY = '-'
BLACK = ‘X’
WHITE = ‘O’
BOARD_SIZE = 8
DIRECTIONS = [(0, 1), (0, -1), (1, 0), (-1, 0), (1, 1), (-1, -1), (1, -1), (-1, 1)]
def initialize_board():
board = [[EMPTY for _ in range(BOARD_SIZE)] for _ in range(BOARD_SIZE)]
board[3][3] = WHITE
board[3][4] = BLACK
board[4][3] = BLACK
board[4][4] = WHITE
return board
def print_board(board):
print(" 1 2 3 4 5 6 7 8")
for i in range(BOARD_SIZE):
print(f"{i + 1} ", end="")
for j in range(BOARD_SIZE):
print(f" {board[i][j]}", end="")
print()
def is_valid_move(board, row, col, player):
if board[row][col] != EMPTY:
return False
for direction in DIRECTIONS:
dr, dc = direction
r, c = row + dr, col + dc
while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE:
if board[r][c] == EMPTY:
break
if board[r][c] == player:
return True
r += dr
c += dc
return False
def make_move(board, row, col, player):
if not is_valid_move(board, row, col, player):
return False
board[row][col] = player
for direction in DIRECTIONS:
dr, dc = direction
r, c = row + dr, col + dc
to_flip = []
while 0 <= r < BOARD_SIZE and 0 <= c < BOARD_SIZE:
if board[r][c] == EMPTY:
break
if board[r][c] == player:
for flip_row, flip_col in to_flip:
board[flip_row][flip_col] = player
break
to_flip.append((r, c))
r += dr
c += dc
return True
def is_game_over(board):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
return False
return True
def count_pieces(board):
black_count = 0
white_count = 0
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == BLACK:
black_count += 1
elif board[i][j] == WHITE:
white_count += 1
return black_count, white_count
def determine_winner(black_count, white_count):
if black_count > white_count:
return BLACK
elif white_count > black_count:
return WHITE
else:
return EMPTY
def play_game():
board = initialize_board()
player = BLACK
while True:
print_board(board)
print("Player", player, "'s turn.")
print("Enter your move (row col): ")
move = input().split()
if len(move) != 2:
print("Invalid move. Please enter row and col separated by space.")
continue
row, col = int(move[0]) - 1, int(move[1]) - 1
if not is_valid_move(board, row, col, player):
print("Invalid move. Please choose a valid move.")
continue
make_move(board, row, col, player)
if is_game_over(board):
break
player = WHITE if player == BLACK else BLACK
black_count, white_count = count_pieces(board)
winner = determine_winner(black_count, white_count)
print_board(board)
print("Game Over!")
print("Black: ", black_count)
print("White: ", white_count)
if winner == BLACK:
print("Black wins!")
elif winner == WHITE:
print("White wins!")
else:
print("It's a draw!")
if __name__ == "__main__":
play_game()

Step by stepSolved in 3 steps

- int A[3] [4]={{ 4, 6, 7, 9},{ 1, 3, 4, 3},{-5, 6, 7, 2}}; int sum, row, col; sum = 0; for (col=0;col<=3;i++) sum+=A[0] [col]; what is the value of sum calculated the number of elements on row 0 the total addition of elements on row 0 the total addition of first 3 elements on row 0 the total addition of all the elementsarrow_forward{JAVA data structure set} Suppose you have a list of numbers: numbers = [1, 2, 13, 4, 4, 5, 5, 6, 6, 7, 7, 12] Convert this list into a set and assign it to a new variable called unique_numbers. Print unique_numbers to see the result.arrow_forwardIn Python, grades_dict = {'Wally': [87, 96, 70], 'Eva': [100, 87, 90], 'Sam': [94, 77, 90], 'Katie': [100, 81, 82], 'Bob': [83, 65, 85]} plot 5 box plots one for each student within a single graph. Note: Rename the x-axis data to students’ names using: plt.xticks([1, 2, 3, 4 ,5], [’Student Name 1’, ’Student Name 2’, ’Student Name 3’, ’Student Name 4’, ’Student Name 5’]), see Figure in the next page. The values in these two arguments should be retrieved automatically and should work for any number of students not just 5'''arrow_forward
- This is loosely based on a program in Python for a sub-list sorting program. What are the coupling and cohesion levels in each function block in this structure chart, and explain what makes them that type of coupling and cohesion:.arrow_forwardThe Spider Game Introduction: In this assignment you will be implementing a game that simulates a spider hunting for food using python. The game is played on a varying size grid board. The player controls a spider. The spider, being a fast creature, moves in the pattern that emulates a knight from the game of chess. There is also an ant that slowly moves across the board, taking steps of one square in one of the eight directions. The spider's goal is to eat the ant by entering the square it currently occupies, at which point another ant begins moving across the board from a random starting location. Game Definition: The above Figure illustrates the game. The yellow box shows the location of the spider. The green box is the current location of the ant. The blue boxes are the possible moves the spider could make. The red arrow shows the direction that the ant is moving - which, in this case, is the horizontal X-direction. When the ant is eaten, a new ant is randomly placed on one of the…arrow_forwarddef is_balanced(root): return __is_balanced_recursive(root) def __is_balanced_recursive(root): """ O(N) solution """ return -1 != __get_depth(root) def __get_depth(root): """ return 0 if unbalanced else depth + 1 """ if root is None: return 0 left = __get_depth(root.left) right = __get_depth(root.right) if abs(left-right) > 1 or -1 in [left, right]: return -1 return 1 + max(left, right) # def is_balanced(root):# """# O(N^2) solution# """# left = max_height(root.left)# right = max_height(root.right)# return abs(left-right) <= 1 and is_balanced(root.left) and# is_balanced(root.right) # def max_height(root):# if root is None:.arrow_forward
- The Java language definition demands that all references to array elements be checked to ensure that the index or indices are in their legal ranges, is an example of VS ____. (one word answers) Blank # 1 Blank # 2 A A/arrow_forwarddef rectangle_overlap(rect1_bl_x,rect1_bl_y, rect1_tr_x,rect1_tr_y, rect2_bl_x,rect2_bl_y, rect2_tr_x,rect2_tr_y): """ (int,int,int,int,int,int,int,int) -> str Function determines whether two rectangles overlap. When rectangles overlap, the function checks for the following scenarios 1. The two rectangles share the same coordinates 2. The first rectangle is contained within the second 3. The second rectangle is contained within the first 4. The rectangles have overlapping area, but neither is completely contained within the other Function inputs represent x and y coordinates of bottom left and top right corners of rectangles (see lab document) The function return one of the following strings corresponding to the scenario "no overlap" "identical coordinates" "rectangle 1 is contained within rectangle 2" "rectangle…arrow_forwardFor any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forward
- JAVA PROGRAM: Monkey Business A local zoo wants to keep track of how many pounds of food each of its three monkeys eats each day during a typical week. Write a program that stores this information in a two-dimensional 3 × 5 array, where each row represents a different monkey and each column represents a different day of the week. The program should first have the user input the data for each monkey, or use constant values rather than asking user for input. Then it should create a report that includes the following information: Display 3X5 array first. Average amount of food eaten per day by the whole family of The least amount of food eaten during the week by any one The greatest amount of food eaten during the week by any one Input Validation: Do not accept negative numbers for pounds of food eaten.arrow_forwardIn Java Assignment 5B : Maze Game! 2D Arrays can be used to store and represent informationabout video game levels or boards. In this exercise, you will use this knowledge tocreate an interactive game where players attempt to move through a maze. You willstart by creating a pre-defined 2D array with the following values:{"_","X","_","X","X"}{"_","X","_","X","W"}{"_","_","_","X","_"}{"X","X","_","_","_"}{"_","_","_","X","X"}You will then set the player (represented by “O”) at index 0, 0 of the array, the top-leftcorner of the maze. You will use a loop to repeatedly prompt the user to enter adirection (“Left”, “Right”, “Up”, or “Down”). Based on these directions, you will try tomove the player.• If the location is valid (represented by “_”), you will move the player there• If the location is out of bounds (e.g. index 0, -1) or the command is invalid, youwill inform the player and prompt them to enter another direction• If the location is a wall (represented by “X”), you will tell the…arrow_forwardstruct Info { int id; float cost; }; Given the struct above, the following code segment will correctly declare and initialize an instance of that struct. Info myInfo = {10,4.99}; Group of answer choices True False ============== Consider the following code fragment. int a[] = {3, -5, 7, 12, 9}; int *ptr = a; *ptr = (*ptr) + 1; cout << *ptr; Group of answer choices 4 -5 3 Address of -5 =============== What will happen if I use new without delete? Group of answer choices The program will never compile The program will never run The program will run fine without memory leak None of thesearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
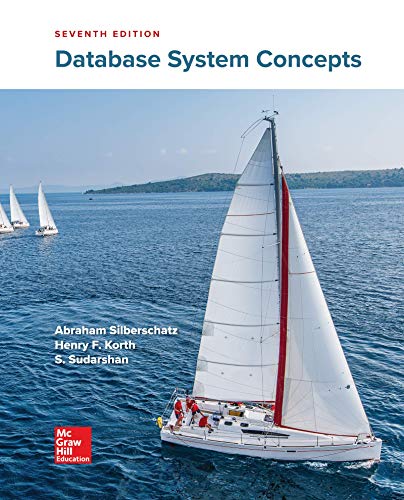
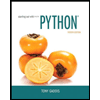
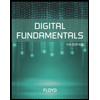
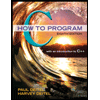
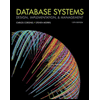
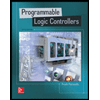