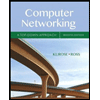
Write an LC-3 assembly language
Here are some hints
ORIG x3000
AND R5, R5, #0
ADD R5, R5, #1 ;R5 will act as a mask
AND R1, R1, #0 ;zero out the result register
AND R2, R2, #0 ;R2 will act as a counter
LD R3, NegSixt
MskLoop AND R4, R0, R5 ;mask off the bit
Add code here... if you want more than a 0
Solicit the number to be inspected via the keyboard.
10 points for a single digit (e.g. 5) decimal number, which must be converted to binary.
20 points for a two digit (e.g. 55) decimal number, which must be converted to binary.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Implementing matrix addition is pretty simple; see the program below. However, the given addition function matrix_add_double() does not run at full speed. On the instructors computer, which has 16GB of RAM, the program below shows that the call to matrix_add_double takes 38571 ms, while another, better implementation achieves the same result in 3794.7ms, which is 10.2 times faster! Your job for this exercise is: • to explain what in the Operating System and/or CPU and/or other part of the system makes the implementation below go so slowly. • to fix the code to achieve full speed. Hint: There are two reasons why the code runs slowly. The one reason is related to how virtual addresses get translated to physical addresses, the other reasons is related to another effect in the hardware. Only the performance for the matrix_add_double function() counts for your exam results. You can leave the other parts of the code untouched, but you may also change them.…arrow_forwardWrite a C++ program that depicts a multiplication table. your program should ask the user to enter the number of rows and columns. Sample output is something like the following: How many rows the multiplication table has? 3 How many columns? 4 your multiplication is the following: 1 2 3 4 1 1 2 3 4 2 2 4 6 8 3 3 6 9 12arrow_forwardWrite a program in python with a function timestable(n), which prints a multiplication table of size n. For example, timestable(5) would print: 12345 2 3 4 5 4 6 8 10 6 9 12 15 8 12 16 20 10 15 20 25Use the function in a program where you ask the user for n and you print the corresponding table.arrow_forward
- Write a C program addition.c that conducts addition operation on two integer numbers passed on the command line An example run of your program is as follows: $> /addition 8 9 The sum of 8 and 9 is 17. $>arrow_forwardWrite a program to handle a user's rolodex entries. (A rolodex is a system with tagged cards each representing a contact. It would contain a name, address, and phone number. In this day and age, it would probably have an email address as well.) Typical operations people want to do to a rolodex entry are: 1) Add entry 2) Edit entry 3) Delete entry 4) Find entry 5) Print all entries 6) Quit You can decide what the maximum number of rolodex entries is and how long each part of an entry is (name, address, etc.). When they choose to edit an entry, give them the option of selecting from the current rolodex entries or returning to the main menu — don't force them to edit someone just because they chose that option. Similarly for deleting an entry. Also don't forget that when deleting an entry, you must move all following entries down to fill in the gap. If they want to add an entry and the rolodex is full, offer them the choice to return to the main menu or select a person to overwrite. When…arrow_forwardIn Python, Given the 2D list below, convert all values to 255 if they are above a threshold or to 0if they are below. The threshold value is given as input by the user a =[[77,68,86,73],[96,87,89,81],[70,90,86,81]]arrow_forward
- Write a C++ program for the given instructions: Summary Suppose that the first number of a sequence is x, where x is an integer. Define: a0 = x; an+1 = an / 2 if an is even; an+1 = 3 X an + 1 if an is odd. Then there exists an integer k such that ak = 1. Instructions Write a program that prompts the user to input the value of x. The program outputs: The numbers a0, a1, a2, . . . , ak. The integer k such that ak = 1 (For example, if x = 75, then k = 14, and the numbers a0, a1, a2, ..., a14, respectively, are 75, 226, 113, 340, 170, 85, 256, 128, 64, 32, 16, 8, 4, 2, 1.) Test your program for the following values of x: 75, 111, 678, 732, 873, 2048, and 65535.arrow_forwardWrite an assembly language program in MIPS that repeatedly asks the user for a scale F or a C (for"Fahrenheit" or "Celsius") on one line followed by an integertemperature on the next line. It then converts the given temperature tothe other scale. Use the formulas:F = (9/5)C + 32C = (5/9)(F - 32)2. Exit the loop when the user types "Q/q". Assume that all input is correct.For example:Enter Scale : FEnter Temperature: 32Celsius Temperature: 0CEnter Scale : CEnter Temperature: 100Fahrenheit Temperature: 212FEnter Scale : Qdoneprovide a picture of the output.arrow_forwardWrite a C program that asked the user to enter an integer MAX where 101000. The program then produces the following table of y=x/n where x=1 ..MAX; n=1 ..5 TABLE OF DIVISION 1 | 1 | Y.yyy | 2 | Y.yyy | | Y.yyy | 4 | Y.yyy | 3 | MAX | Y.yyy 2 | 3 Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Y.yyy | Note: Y.yyy are values of y=x/n displayed with 3 decimals. 4 | 5 | Y.yyy | Y.yyy Y.yyy | Y.yyy Y.yyy | Y.yyy | Y.yyy Y.yyy Y.yyy | Y.yyyarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
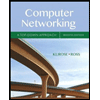
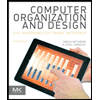
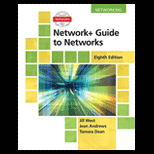
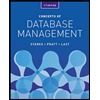
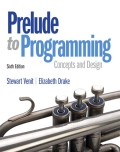
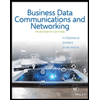