Write a Python program that takes a dictionary as an input and then outputs the average of all the values in the dictionary. [You are not allowed to use len() and sum()] =================================================================== Hint: you can use dictionary functions to get all the values from it, run loop to calculate the sum and the total number of values in the dictionary in order to find out the average. =================================================================== Sample Input 1: {'Jon': 100, 'Dan':200, 'Rob':300} Sample Output 1: Average is 200. =================================================================== Sample Input 2: {'Jon': 100, 'Dan':200, 'Rob':30, 'Ned':110} Sample Output 2: Average is 110. so when I call my function, I get "Average is 110" but I need to get rid of quotation marks and have dot at the end like sample s Avarge is 110.
Write a Python program that takes a dictionary as an input and then outputs the average of all the values in the dictionary.
[You are not allowed to use len() and sum()]
===================================================================
Hint: you can use dictionary functions to get all the values from it, run loop to calculate the sum and the total number of values in the dictionary in order to find out the average.
===================================================================
Sample Input 1:
{'Jon': 100, 'Dan':200, 'Rob':300}
Sample Output 1:
Average is 200.
===================================================================
Sample Input 2:
{'Jon': 100, 'Dan':200, 'Rob':30, 'Ned':110}
Sample Output 2:
Average is 110.
so when I call my function, I get "Average is 110" but I need to get rid of quotation marks and have dot at the end like sample s Avarge is 110.
![In [69]: #todo
def task8(dict_in):
# YOUR CODE HERE
S=0
c=1
for i in dict_in.values():
S+=i
C+=1
avg=(int(s/(c-1))
str_out="Average is "+str(avg)+"."
return str_out
Input In [69]
str_out="Average is "+str(avg)+"."
A
SyntaxError: invalid syntax
In [68]: assert task8({'Jon': 100, 'Dan':200,
assert task8({'Jon': 100, 'Dan' :200,
'Rob':30, 'Ned':110})
'Rob':300})
==
In [71]: task8({'Jon': 100, 'Dan':200, 'Rob':30, 'Ned': 110})
Out [71]: 'Average is 110¹
==
"Average is 110."
"Average is 200."
AssertionError
Traceback (most recent call last)
Input In [68], in <cell line: 1>()
----> 1 assert task8({'Jon': 100, 'Dan':200, 'Rob':30, 'Ned' :110})
2 assert task8({'Jon': 100, 'Dan':200, 'Rob' :300}) "Average is 200."
AssertionError:
==
=
"Average is 110."](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa1d592a5-ec96-4b1d-bddf-23e27014debe%2F33ac6441-c08b-4997-b2d7-273b52bcca3b%2Ft81xhbt_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

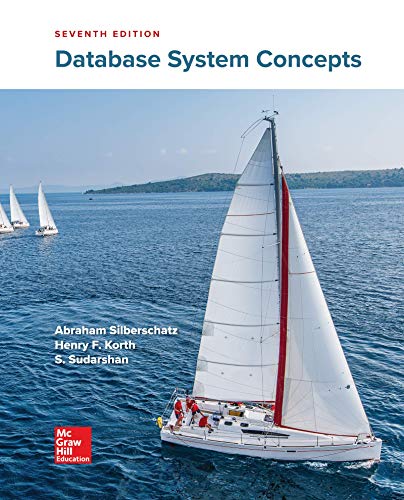
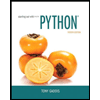
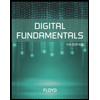
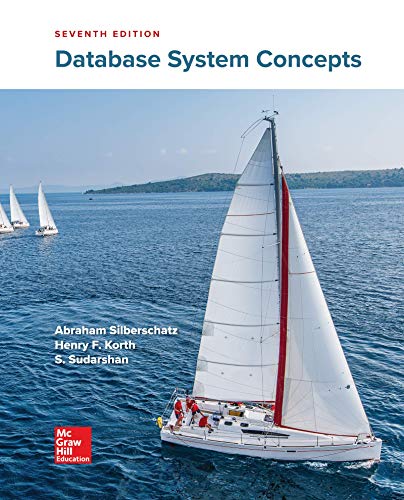
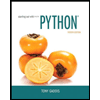
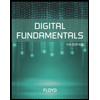
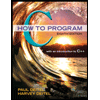
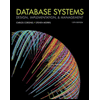
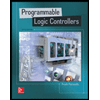