Write a python program that takes two lists, merges the two lists, sorts the resulting list, and then finds the median of the elements in the two lists. [You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead] ===================================================== Sample Input 1 list_one = [1, 2, 1, 4] list_two = [5, 4, 1] Sample Output 1 Sorted list = [1, 1, 1, 2, 4, 4, 5] Median = 2 ===================================================== Sample Input 2 list_one = [1, 7, 9, 10] list_two = [2, 7, 6, 5] Sample Output 2 Sorted list = [1, 2, 5, 6, 7, 7, 9, 10] Median = 6.5 #todo #You can use math functions like math.floor to help the calculation import math def task7(list_in_1, list_in_2): # YOUR CODE HERE return median please only use list_in_1, list_in_2 and return median no other function allowed and return median please use only them and no sort function thank you so much
Write a python
[You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead]
=====================================================
Sample Input 1
list_one = [1, 2, 1, 4]
list_two = [5, 4, 1]
Sample Output 1
Sorted list = [1, 1, 1, 2, 4, 4, 5]
Median = 2
=====================================================
Sample Input 2
list_one = [1, 7, 9, 10]
list_two = [2, 7, 6, 5]
Sample Output 2
Sorted list = [1, 2, 5, 6, 7, 7, 9, 10]
Median = 6.5
#todo
#You can use math functions like math.floor to help the calculation
import math
def task7(list_in_1, list_in_2):
# YOUR CODE HERE
return median
please only use list_in_1, list_in_2 and return median no other function allowed and return median please use only them and no sort function thank you so much

Step by step
Solved in 2 steps with 1 images

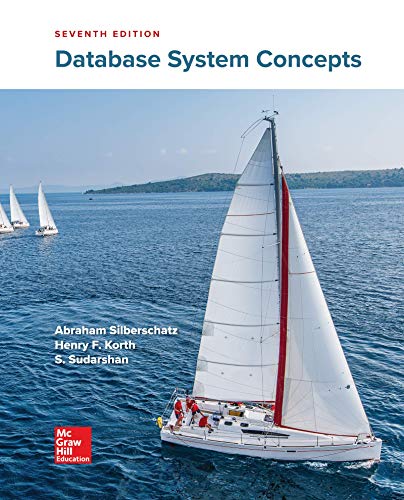
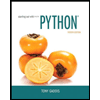
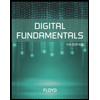
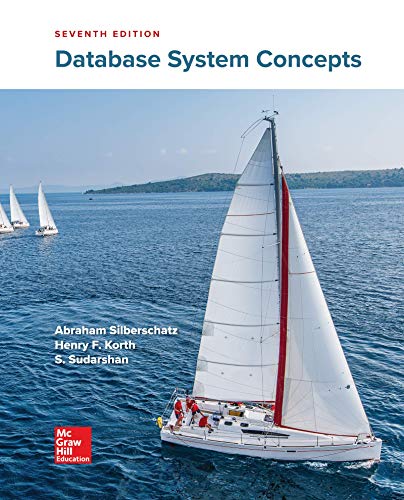
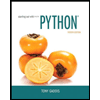
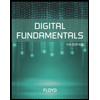
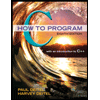
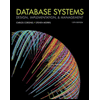
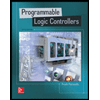