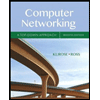
Write a python program that will take input from the user until the user
gives “STOP” as input. In every line the user will provide input as follow:
[Brand Name] [PRICE]
Your task is to create a dictionary where the brand’s name will be key and
the value will be a list of that brand’s watches ID. ID generate process:
inputno_FirstTwoLetterOfBrand_Price. Finally print the dictionary.
Sample Input 1:
OMEGA 10000
TITAN 5000
TITAN 3000
CREDENCE 150000
OMEGA 12000
CREDENCE 90000
STOP
Sample Output 1:
{'OMEGA': ['1_OM_10000', '5_OM_12000'], 'TITAN':
['2_TI_5000', '3_TI_3000'], 'CREDENCE': ['4_CR_150000', '6_CR_90000']}
Sample Input 2:
CREDENCE 5000
CREDENCE 11000
OMEGA 8000
TITAN 7500
QUARTZ 8000
CASIO 6000
CASIO 7000
STOP
Sample Output 2:
{'CREDENCE': ['1_CR_5000', '2_CR_11000'], 'OMEGA': ['3_OM_8000'], 'TITAN':
['4_TI_7500'], 'QUARTZ': ['5_QU_8000'], 'CASIO': ['6_CA_6000', '7_CA_7000']}
![Write a python program that will take input from the user until the user
gives "STOP" as input. In every line the user will provide input as follow:
[Brand Name] [PRICE]
Your task is to create a dictionary where the brand's name will be key and
the value will be a list of that brand's watches ID. ID generate process:
inputno_FirstTwoLetterOfBrand_Price. Finally print the dictionary.
Sample Input 1:
OMEGA 10000
TITAN 5000
TITAN 3000
CREDENCE 150000
OMEGA 12000
CREDENCE 90000
STOP
Sample Output 1:
('OMEGA": ['1_OM_10000', '5_OM_12000'], 'TITAN":
[2_TL_5000', "3_TI_3000], "CREDENCE: [4_CR_150000', '6_CR_90000']}
Sample Input 2:
CREDENCE 5000
CREDENCE 11000
OMEGA 8000
TITAN 7500
QUARTZ 8000
CASIO 6000
CASIO 7000
STOP
Sample Output 2:
{'CREDENCE": ['1_CR_5000', '2_CR_11000'), 'OMEGA": ['3 OM_8000'), 'TITAN':
[4_TI_7500], 'QUARTZ": ['5_QU_8000'], 'CASIO: ['6_CA_6000', 7_CA_7000]}](https://content.bartleby.com/qna-images/question/8208a85f-3b3c-453e-8fea-3541e20e4da1/337d973f-43fd-4bfa-b0d5-ff914824bbaa/3xupxtm_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Please in python, MUST use dictionaries This program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers and the ratings in a dictionary. Output the dictionary's elements with the jersey numbers in ascending order (i.e., output the roster from smallest to largest jersey number). Hint: Dictionary keys can be stored in a sorted list. (3 pts)Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Jersey number: 4, Rating: 5 Jersey number: 23, Rating: 4 Jersey number 30, Rating: 2 ... (2) Implement a menu of options for…arrow_forwardTry to create a dictionary that has a listing of people and includes one interesting fact about each of them. Display each person and their interesting fact on the screen. From there, alter a fact about one of the people on the list. Also, add an extra person and corresponding fact to the list. Display the newly created list of people and facts. Try running the program multiple times and take note of whether the order changes. Sample output: Jeff: Was born in France. David: Was a mascot in college. Anna: Has arachnophobia. Dylan: Has a pet hedgehog. Jeff: Was born in France. David: Can juggle. Anna: Has arachnophobia. Code Listing 209 106arrow_forwardWrite a function named count_vowels that accepts a string as an argument. The function should count the number of times each vowel (the letters a, e, i, o, and u) appears in the string, and store those counts in a dictionary. When the function ends, the dictionary should have exactly 5 elements. In each element, the key will be a vowel (lowercase) and the value will be the number of times the vowel appears in the string.arrow_forward
- Need some help on this Python Quesionarrow_forwardIn pythonWrite a function called compter_les_votes that accepts a list of ballots as input (of arbitrary length) and produces a report as a dictionary. The ballots have the form of a couple (valid, condidat) where candidate is the name of one of the candidates in the election, and valid is a boolean that indicates whether or not the ballot is valid. For example, for the following list of bulletins: [(True, 'Pierre'), (False, 'Jean'), (True, 'Pierre'), (True, 'Jacques')]Your function must return the following dictionary: { "number of ballots": 4, "invalid ballots": 1, "results": { "Stone": 2, "Jacques": 1 }}Note that you do not have to display the dictionary, only return it.arrow_forwardThis section has been set as optional by your instructor. OBJECTIVE: Write a program in Python that prints the middle value of a dictionary's values, using test_dict from the test_data.py module. NOTE: test_dict is created & imported from the module test_data.py into main.py Example 1: test dict = {'a':1,'b':9,'c':2,'d':5} prints 5 Example 2: test_dict = {'b':9,'d':5} prints 5 Hint: You may(or may not) find the following function useful: list.sort() which sorts the elements in a list. Note: the result is automatically stored in the original list, and return value from the function is None Туре list = [1, 2,3,2,1] After calling: list = [1,1,2,2,3] list.sort(), 339336.2266020 x3zgy7 LAB 13.11.1: Middlest Value (Structured Types) АCTIVITY 0/11 File is marked as read only Current file: test_data.py 1 #This module creates the test_dict to be used in main.py 2 #Reads input values from the user & places them into test_dict 3 dict_size = int(input()) #Stores the desired size of test_dict. 4…arrow_forward
- Write a Python program that takes a dictionary as an input and then outputs the average of all the values in the dictionary. [You are not allowed to use len() and sum()] Hint: you can use dictionary functions to get all the values from it, run loop to calculate the sum and the total number of values in the dictionary in order to find out the average. def task8(dict_in): # YOUR CODE HERE return str_out could you please use dict_in and str_outarrow_forwardAs part of this assignment, the program that you will be writing will store current grades in a dictionary using course codes as keys and with values consisting of percent grades in lists. The main functions of this program are to print a student's gradebook, to drop the lowest grade in each course, print the student's gradebook again, drop the course with lowest average, and finally printing the student's gradebook again. This program requires a main function and a custom value-returning function. In the main function, code these basic steps in this sequence (intermediate steps may be missing): start with an empty dictionary that represents a gradebook and then use a while loop to allow the input of course codes from the keyboard. End the while loop when the user presses enter without entering data.within the while loop:for each course entered, use a list comprehension to generate five random integers in the range of 70 through 100. These random integers in a list represent the…arrow_forwardHelp me on below python program.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
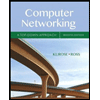
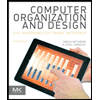
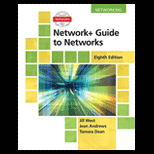
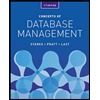
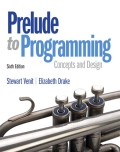
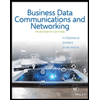