Write a program to implement the algorithm for evaluating postfix expressions that involve only single-digit integers and the integer operations +, -, *, and /. To trace the action of postfix evaluation, display each token as it is encountered, and display the action of each stack operation. 1. Each digit or operator should be separated by a space, and end with ':' character. 2. You should apply the stack ADT in the program. 3. You may need to use function is digit(int c) in standard header file #include to check if the token character is a digit or not. 4. To convert the single-digit char c to the represented integer num, a simple method is to assign num by aChar - '0'
Write a
1. Each digit or operator should be separated by a space, and end with ':' character.
2. You should apply the stack ADT in the program.
3. You may need to use function is digit(int c) in standard header file #include <cctype> to check if the token character is a digit or not.
4. To convert the single-digit char c to the represented integer num, a simple method is to assign num by aChar - '0'

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

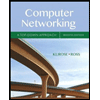
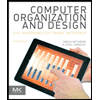
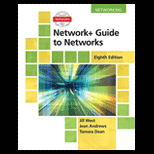
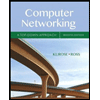
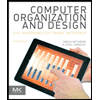
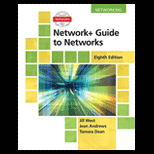
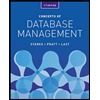
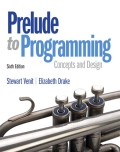
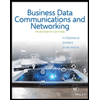